LeetCode刷题实战173:二叉搜索树迭代器
题意
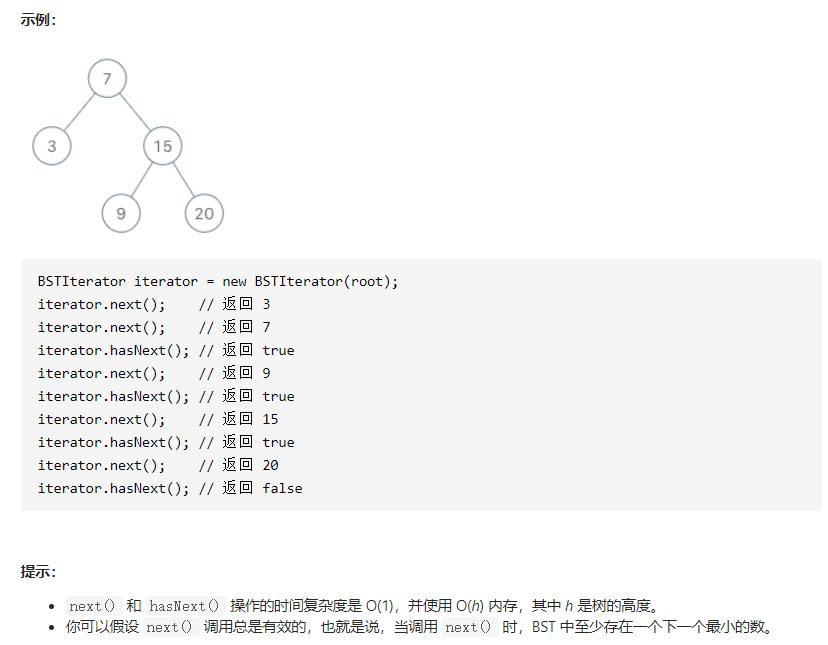
解题
class TreeNode{
int val;
TreeNode left;
TreeNode right;
TreeNode(int x){
val = x;
}
}
class BSTIterator {
int index;
ArrayListlist;
public BSTIterator(TreeNode root) {
this.list = new ArrayList<>();
this.index = -1;
this.inorder(root);
}
// 先进行中序遍历
private void inorder(TreeNode root){
if(root==null){
return;
}
inorder(root.left);
list.add(root.val);
inorder(root.right);
}
/** @return the next smallest number */
public int next() {
return list.get(++index);
}
/** @return whether we have a next smallest number */
public boolean hasNext() {
return this.index+1 < this.list.size();
}
}
class BSTIterator {
// 栈
Stackstack;
public BSTIterator(TreeNode root) {
// 初始化
stack = new Stack<>();
this.pre(root);
}
// 先存储一部分值
private void pre(TreeNode root){
while(root!=null){
stack.push(root);
root = root.left;
}
}
/** @return the next smallest number */
public int next() {
TreeNode temp = this.stack.pop();
if(temp.right!=null){
this.pre(temp.right);
}
return temp.val;
}
/** @return whether we have a next smallest number */
public boolean hasNext() {
return this.stack.size()>0;
}
}