SpringBoot整合Mybatis图文教程
这里介绍两种整合SpringBoot和Mybatis的模式,分别是“全注解版” 和 “注解xml合并版”。
前期准备
开发环境
开发工具:
IDEA
JDK:
1.8
技术:
SpringBoot、Maven、Mybatis
创建项目
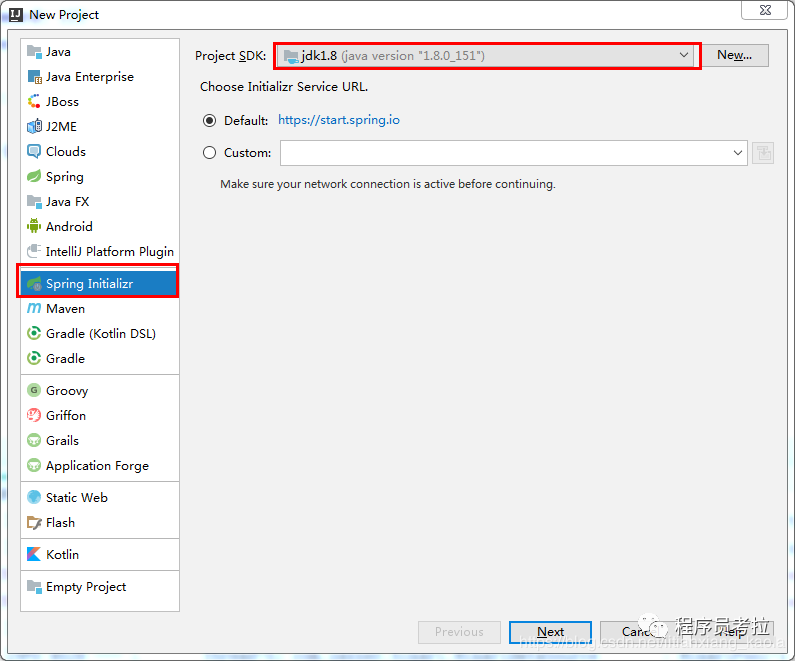
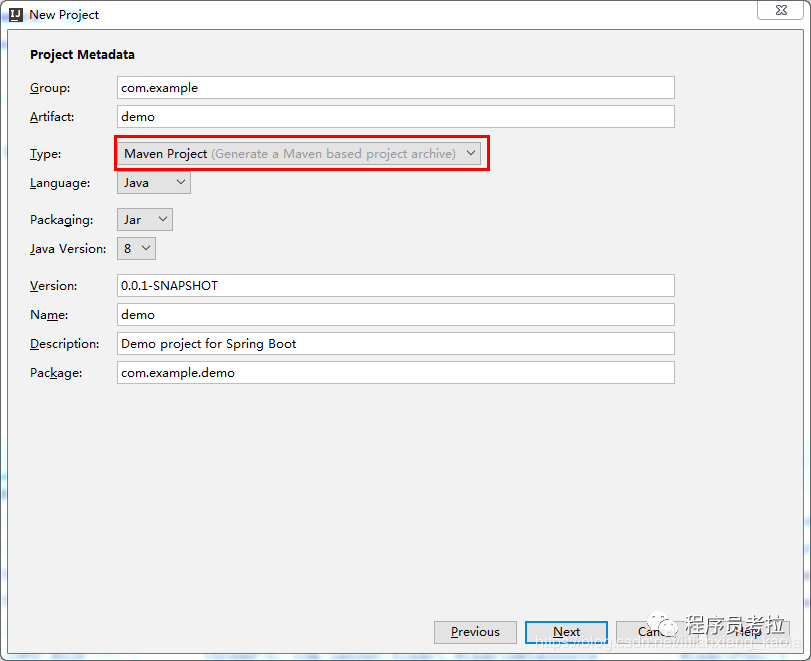
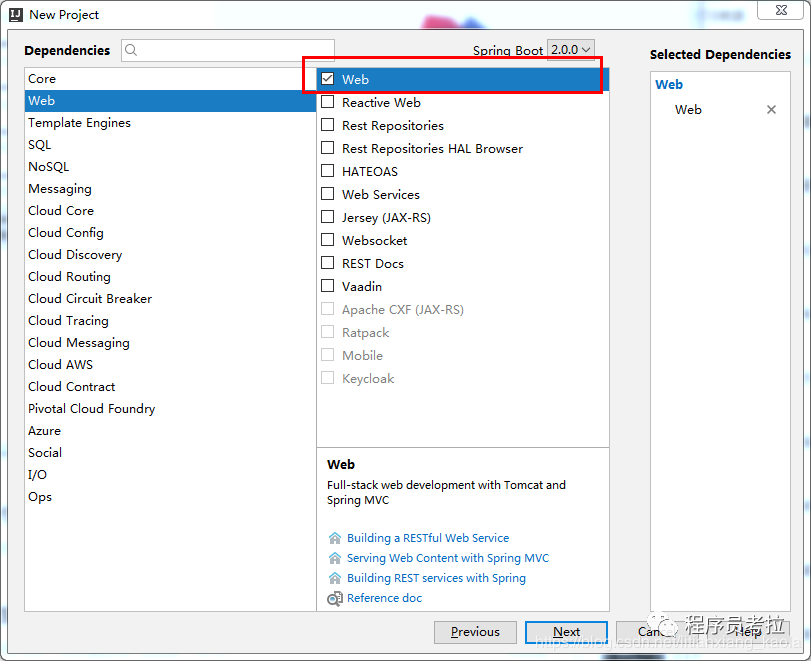
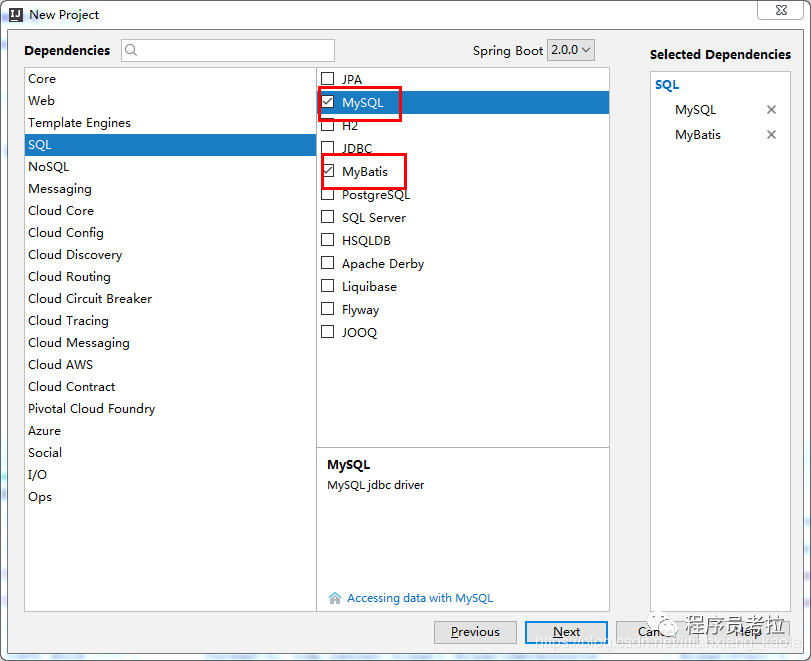
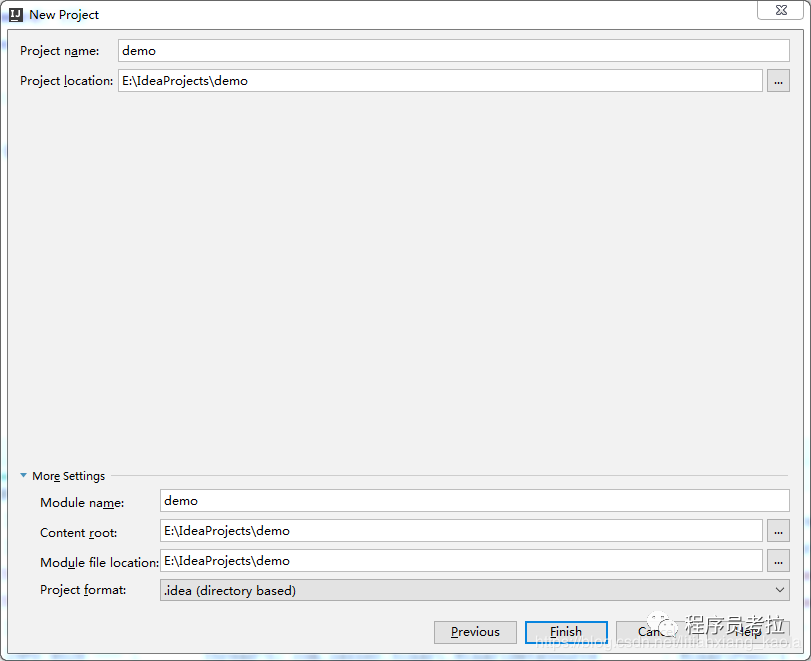
项目结构
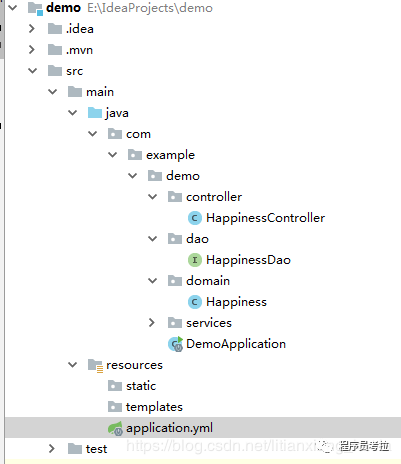
Maven依赖
xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0modelVersion>
<groupId>com.examplegroupId>
<artifactId>demoartifactId>
<version>0.0.1-SNAPSHOTversion>
<packaging>jarpackaging>
<name>demoname>
<description>Demo project for Spring Bootdescription>
<parent>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-parentartifactId>
<version>2.0.0.RELEASEversion>
<relativePath/>
parent>
<properties>
<project.build.sourceEncoding>UTF-8project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8project.reporting.outputEncoding>
<java.version>1.8java.version>
properties>
<dependencies>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-webartifactId>
dependency>
<dependency>
<groupId>org.mybatis.spring.bootgroupId>
<artifactId>mybatis-spring-boot-starterartifactId>
<version>1.3.1version>
dependency>
<dependency>
<groupId>mysqlgroupId>
<artifactId>mysql-connector-javaartifactId>
<scope>runtimescope>
dependency>
<dependency>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-starter-testartifactId>
<scope>testscope>
dependency>
dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.bootgroupId>
<artifactId>spring-boot-maven-pluginartifactId>
plugin>
plugins>
build>
project>
全注解版
SpringBoot配置文件
这里使用yml格式的配置文件,将application.properties改名为application.yml。
#配置数据源
spring:
datasource:
url: jdbc:mysql://127.0.0.1:3306/dianping?useUnicode=true&characterEncoding=utf8
username: root
password: 123
driver-class-name: com.mysql.jdbc.Driver
SpringBoot会自动加载application.yml相关配置,数据源就会自动注入到sqlSessionFactory中,sqlSessionFactory会自动注入到Mapper中。
实体类
public class Happiness {
private Long id;
private String city;
private Integer num;
//getters、setters、toString
}
映射类
@Mapper
public interface HappinessDao {
@Select("SELECT * FROM happiness WHERE city = #{city}")
Happiness findHappinessByCity(@Param("city") String city);
@Insert("INSERT INTO happiness(city, num) VALUES(#{city}, #{num})")
int insertHappiness(@Param("city") String city, @Param("num") Integer num);
}
Service类
事务管理只需要在方法上加个注解:@Transactional
@Service
public class HappinessService {
@Autowired
private HappinessDao happinessDao;
public Happiness selectService(String city){
return happinessDao.findHappinessByCity(city);
}
@Transactional
public void insertService(){
happinessDao.insertHappiness("西安", 9421);
int a = 1 / 0; //模拟故障
happinessDao.insertHappiness("长安", 1294);
}
}
Controller类
@RestController
@RequestMapping("/demo")
public class HappinessController {
@Autowired
private HappinessService happinessService;
@RequestMapping("/query")
public Happiness testQuery(){
return happinessService.selectService("北京");
}
@RequestMapping("/insert")
public Happiness testInsert(){
happinessService.insertService();
return happinessService.selectService("西安");
}
}
测试
http://localhost:8080/demo/query
http://localhost:8080/demo/insert
注解xml合并版
项目结构
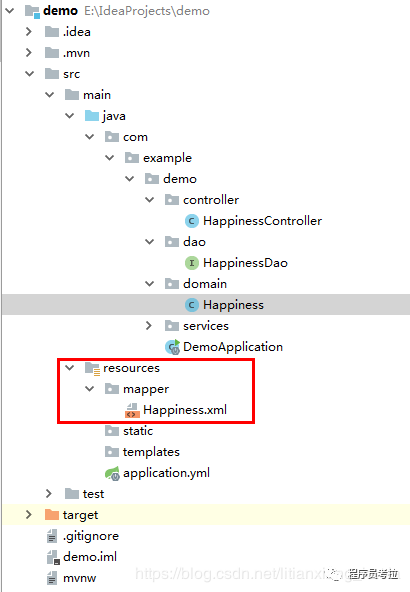
SpringBoot配置文件
#配置数据源
spring:
datasource:
url: jdbc:mysql://127.0.0.1:3306/dianping
username: root
password: 123
driver-class-name: com.mysql.jdbc.Driver
#指定mybatis映射文件的地址
mybatis:
mapper-locations: classpath:mapper/*.xml
映射类
@Mapper
public interface HappinessDao {
Happiness findHappinessByCity(String city);
int insertHappiness(HashMap<String, Object> map);
}
映射文件
<mapper namespace="com.example.demo.dao.HappinessDao">
<select id="findHappinessByCity" parameterType="String" resultType="com.example.demo.domain.Happiness">
SELECT * FROM happiness WHERE city = #{city}
select>
<insert id="insertHappiness" parameterType="HashMap" useGeneratedKeys="true" keyProperty="id">
INSERT INTO happiness(city, num) VALUES(#{city}, #{num})
insert>
mapper>
Service类
事务管理只需要在方法上加个注解:@Transactional
@Service
public class HappinessService {
@Autowired
private HappinessDao happinessDao;
public Happiness selectService(String city){
return happinessDao.findHappinessByCity(city);
}
@Transactional
public void insertService(){
HashMap map = new HashMap();
map.put("city", "西安");
map.put("num", 9421);
happinessDao.insertHappiness(map);
int a = 1 / 0; //模拟故障
happinessDao.insertHappiness(map);
}
}
Controller类
@RestController
@RequestMapping("/demo")
public class HappinessController {
@Autowired
private HappinessService happinessService;
@RequestMapping("/query")
public Happiness testQuery(){
return happinessService.selectService("北京");
}
@RequestMapping("/insert")
public Happiness testInsert(){
happinessService.insertService();
return happinessService.selectService("西安");
}
}
测试
http://localhost:8080/demo/query
http://localhost:8080/demo/insert
原文链接:csdn.net/litianxiang_kaola/article/details/79481422
评论