这几个Python内置的高阶函数,真香
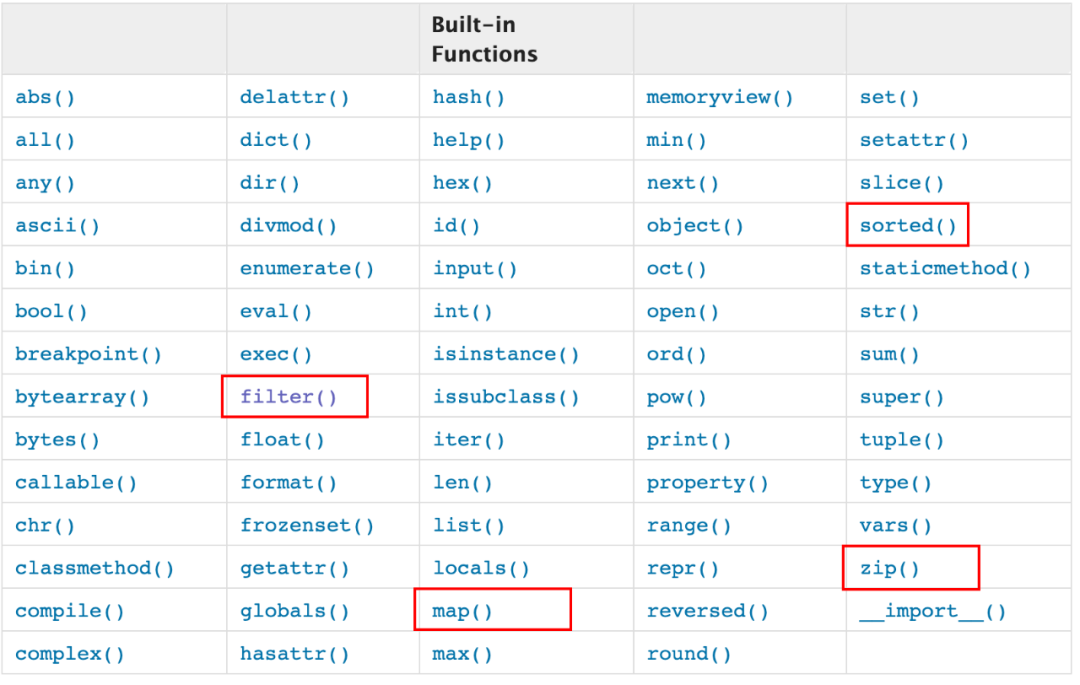
奇怪,reduce去哪了?
1、sorted 函数
sorted(iterable, *, key=None, reverse=False)
key = str.lower
,如果不指定,则默认按照可迭代对象中的元素进行比较。>>> v_list = [5,2,3,4,1]
>>> sorted(v_list)
[1, 2, 3, 4, 5]
>>> v_tuple = (5,2,3,4,1)
>>> sorted(v_tuple)
[1, 2, 3, 4, 5]
>>> v_dict = {5:'a',2:'b',3:'c',4:'d',1:'e'}
>>> sorted(v_dict)
[1, 2, 3, 4, 5]
>>>
>>> v_dict = {5:'a',2:'b',3:'c',4:'d',1:'e'}
>>> sorted(v_dict,key=lambda x:v_dict[x])
[5, 2, 3, 4, 1]
>>> student_tuples = [
... ('john', 'A', 15),
... ('jane', 'B', 12),
... ('dave', 'B', 10),
... ]
>>> sorted(student_tuples, key=lambda student: student[2]) # sort by age
[('dave', 'B', 10), ('jane', 'B', 12), ('john', 'A', 15)]
class Student:
def __init__(self, name, grade, age):
self.name = name
self.grade = grade
self.age = age
def __repr__(self):
return repr((self.name, self.grade, self.age))
student_objects = [
Student('john', 'A', 15),
Student('jane', 'B', 12),
Student('dave', 'B', 10),
]
sorted(student_objects, key=lambda student: student.age) # sort by age
#[('dave', 'B', 10), ('jane', 'B', 12), ('john', 'A', 15)]
>>> student_tuples
[('john', 'A', 15), ('jane', 'B', 12), ('dave', 'B', 10)]
>>> from operator import itemgetter, attrgetter
>>> sorted(student_tuples, key=itemgetter(2))
[('dave', 'B', 10), ('jane', 'B', 12), ('john', 'A', 15)]
>>> class Student:
... def __init__(self, name, grade, age):
... self.name = name
... self.grade = grade
... self.age = age
... def __repr__(self):
... return repr((self.name, self.grade, self.age))
...
>>> student_objects = [
... Student('john', 'A', 15),
... Student('jane', 'B', 12),
... Student('dave', 'B', 10),
... ]
>>> student_objects
[('john', 'A', 15), ('jane', 'B', 12), ('dave', 'B', 10)]
>>> sorted(student_objects, key=attrgetter('age'))
[('dave', 'B', 10), ('jane', 'B', 12), ('john', 'A', 15)]
>>>
>>> sorted(student_tuples, key=itemgetter(1,2))
[('john', 'A', 15), ('dave', 'B', 10), ('jane', 'B', 12)]
>>> sorted(student_objects, key=attrgetter('grade', 'age'))
[('john', 'A', 15), ('dave', 'B', 10), ('jane', 'B', 12)]
>>> sorted(student_tuples, key=itemgetter(2), reverse=True)
[('john', 'A', 15), ('jane', 'B', 12), ('dave', 'B', 10)]
>>> data = [('red', 1), ('blue', 1), ('red', 2), ('blue', 2)]
>>> sorted(data, key=itemgetter(0))
[('blue', 1), ('blue', 2), ('red', 1), ('red', 2)]
2、filter 函数
filter(function, iterable)
>>> v_list = [1,2,3,4,5,6]
>>> new = filter(lambda x: x%2 ==0, v_list)
>>> list(new)
[2, 4, 6]
>>> number_list = range(-5, 5)
>>> less_than_zero = list(filter(lambda x: x < 0, number_list))
>>> less_than_zero
[-5, -4, -3, -2, -1]
3、zip 函数
zip(*iterables)
>>> list(zip("abc","xy"))
[('a', 'x'), ('b', 'y')]
>>> list(zip("abc","xy",[1,2,3,4]))
[('a', 'x', 1), ('b', 'y', 2)]
def zip(*iterables):
# zip('ABCD', 'xy') --> Ax By
sentinel = object()
iterators = [iter(it) for it in iterables]
while iterators:
result = []
for it in iterators:
elem = next(it, sentinel)
if elem is sentinel:
return
result.append(elem)
yield tuple(result)
>>> x = [1, 2, 3]
>>> y = [4, 5, 6]
>>> zipped = zip(x, y)
>>> list(zipped)
[(1, 4), (2, 5), (3, 6)]
>>> array = [ [1,2,3,4],[5,6,7,8],[9,10,11,12]]
>>> list(zip(*array))
[(1, 5, 9), (2, 6, 10), (3, 7, 11), (4, 8, 12)]
>>> x = [1,2,3]
>>> y = [4,5]
>>> z = [6,7,8,9]
>>> list(zip(x,y,z))
[(1, 4, 6), (2, 5, 7)]
>>> from itertools import zip_longest
>>> list(zip_longest(x,y,z))
[(1, 4, 6), (2, 5, 7), (3, None, 8), (None, None, 9)]
4、map/reduce 函数
map(function, iterable, …)
reduce(function, iterable[, initializer])
>>> def fun(x):
... return x*x
...
>>> v_list = [1,2,3,4,5,6,7,8,9,10]
>>> map(fun,v_list)
L = []
for n in [1, 2, 3, 4, 5, 6, 7, 8, 9,10]:
L.append(fun(n))
print(L)
>>> from functool import reduce
>>> def add(x,y):
... return x+y
...
>>> reduce(add,[1,3,5,7])
16
>>> from functools import reduce
>>> def fn(x, y):
... return x * 10 + y
...
>>> reduce(fn, [1, 3, 5, 7, 9])
13579
from functools import reduce
DIGITS = {'0': 0, '1': 1, '2': 2, '3': 3, '4': 4, '5': 5, '6': 6, '7': 7, '8': 8, '9': 9}
def char2num(s):
return DIGITS[s]
def str2int(s):
return reduce(lambda x, y: x * 10 + y, map(char2num, s))
Python 官方文档:https://docs.python.org/zh-tw/3/library/functions.html
map/reduce:https://www.liaoxuefeng.com/wiki/1016959663602400/1017329367486080
Timsort:https://zh.wikipedia.org/wiki/Timsort
评论