您应该知道的11个JavaScript和TypeScript速记
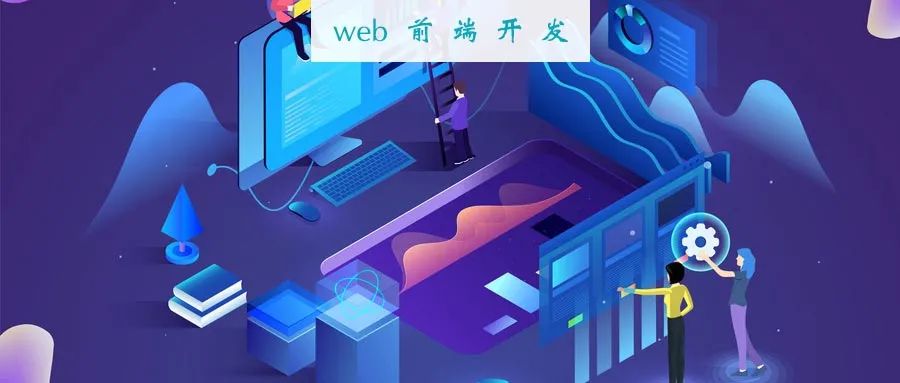
1.空位合并运算符
null
或undefined
,返回的值不完全是该名称所隐含的含义,但是很好。这是您的使用方式:function myFn(variable1, variable2) {
let var2 = variable2 ?? "default value"
return variable1 + var2
}
myFn("this has", " no default value") //returns "this has no default value"
myFn("this has no") //returns "this has no default value"
myFn("this has no", 0) //returns "this has no 0"
2.逻辑无效分配
function myFn(variable1, variable2) {
variable2 ??= "default value"
return variable1 + var2
}
myFn("this has", " no default value") //returns "this has no default value"
myFn("this has no") //returns "this has no default value"
myFn("this has no", 0) //returns "this has no 0"
3. TypeScript的构造函数速记
//Old way...
class Person {
private first_name: string;
private last_name: string;
private age: number;
private is_married: boolean;
constructor(fname:string, lname:string, age:number, married:boolean) {
this.first_name = fname;
this.last_name = lname;
this.age = age;
this.is_married = married;
}
}
//New, shorter way...
class Person {
constructor( private first_name: string,
private last_name: string,
private age: number,
private is_married: boolean){}
}
4.三元运算符
// Original IF..ELSE statement
let isOdd = ""
if(variable % 2 == 0) {
isOdd = "yes"
} else {
isOdd = "no"
}
//The ternary approach
let isOdd = (variable % 2 == 0) ? "yes" : "no"
let variable = true;
(variable) ? console.log("It's TRUE") : console.log("It's FALSE")
5.利用OR的惰性评估
if( expression1 || expression2 || expression3 || expression4)
function myFn(variable1, variable2) {
let var2 = variable2 || "default value"
return variable1 + var2
}
myFn("this has", " no default value") //returns "this has no default value"
myFn("this has no") //returns "this has no default value"
6.双按位NOT运算符
let x = 3.8
let y = ~x //this turns x into -(3 + 1), remember, the number gets turned into an integer
let z = ~y //this turns y (which is -4) into -(-4 + 1) which is 3
//So you can do:
let flooredX = ~~x //and this performs both steps from above at the same time
7.对象属性分配
let name:string = "Fernando";
let age:number = 36;
let id:number = 1;
type User = {
name: string,
age: number,
id: number
}
//Old way
let myUser: User = {
name: name,
age: age,
id: id
}
//new way
let myNewUser: User = {
name,
age,
id
}
8.箭头函数的隐式返回
let myArr:number[] = [1,2,3,4,5,6,7,8,9,10]
//Long way of doing it:
let oddNumbers:number[] = myArr.filter( (n:number) => {
return n % 2 == 0
})
let double:number[] = myArr.map( (n:number) => {
return n * 2;
})
//Shorter way:
let oddNumbers2:number[] = myArr.filter( (n:number) => n % 2 == 0 )
let double2:number[] = myArr.map( (n:number) => n * 2 )
const m = _ => if(2) console.log("true") else console.log("false")
9.默认功能参数
//We can function without the last 2 parameter because a default value
//can be assigned to them
function myFunc(a, b, c = 2, d = "") {
//your logic goes here...
}
const mandatory = _ => {
throw new Error("This parameter is mandatory, don't ignore it!")
}
function myFunc(a, b, c = 2, d = mandatory()) {
//your logic goes here...
}
//Works great!
myFunc(1,2,3,4)
//Throws an error
myFunc(1,2,3)
10.用!!将任何值转换为布尔值!
!!23 // TRUE
!!"" // FALSE
!!0 // FALSE
!!{} // TRUE
11.解构和传播运营商
将对象分解为多个变量
const myObj = {
name: "Fernando",
age: 37,
country: "Spain"
}
//Old way of doing this:
const name = myObj.name;
const age = myObj.age;
const country = myObj.country;
//Using destructuring
const {name, age, country} = myObj;
const { get } from 'lodash'
传播合并
const arr1 = [1,2,3,4]
const arr2 = [5,6,7]
const finalArr = [...arr1, ...arr2] // [1,2,3,4,5,6,7]
const partialObj1 = {
name: "fernando"
}
const partialObj2 = {
age:37
}
const fullObj = { ...partialObj1, ...partialObj2 } // {name: "fernando", age: 37}
两者结合
const myList = [1,2,3,4,5,6,7]
const myObj = {
name: "Fernando",
age: 37,
country: "Spain",
gender: "M"
}
const [head, ...tail] = myList
const {name, age, ...others} = myObj
console.log(head) //1
console.log(tail) //[2,3,4,5,6,7]
console.log(name) //Fernando
console.log(age) //37
console.log(others) //{country: "Spain", gender: "M"}
const [...values, lastItem] = [1,2,3,4]
结论

评论