CSS 实现元素水平垂直居中的 N 种方式
回复交流,加入前端编程面试算法每日一题群
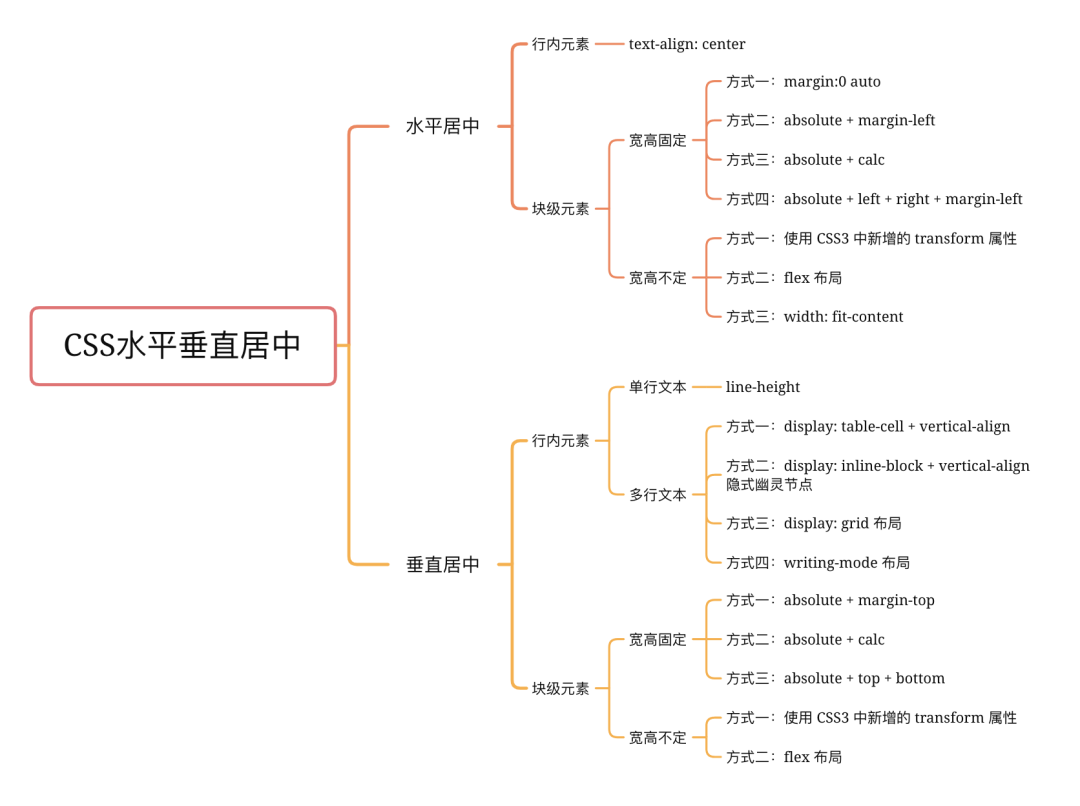
水平居中
1. 行内元素
若是行内元素,给其父元素设置 text-align:center
即可实现行内元素水平居中
<div class="parent">
<span class="child">测试</span>
</div>
<style>
.parent {
background-color: aqua;
text-align: center; /* 水平居中 */
}
.child {
color: #fff;
background-color: blueviolet;
}
</style>

2. 块级元素
2.1 宽高固定
当宽高固定时,以下 html 示例:
<div class="parent">
<div class="child"></div>
</div>
<style>
.parent {
height: 100px;
background-color: aqua;
}
.child {
width: 100px;
height: 100px;
background-color: blueviolet;
}
</style>
以下四种方式显示效果均为:
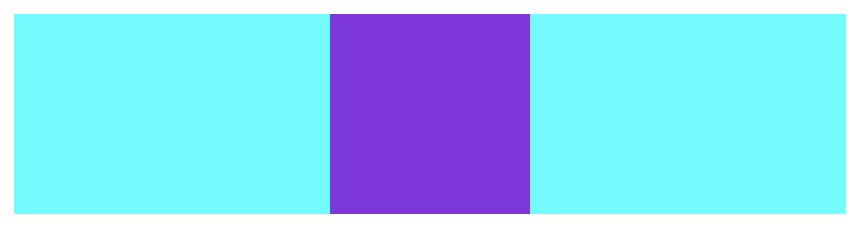
方式一:margin:0 auto
<style>
.child {
margin:0 auto;
}
</style>
方式二:absolute + margin-left
<style>
.child {
position: absolute;
margin-left: -50px;
left: 50%;
}
</style>
方式三:absolute + calc
<style>
.child {
position: absolute;
left: calc(50% - 50px);
}
</style>
方式四:absolute + left + right + margin-left
<style>
.child {
position: absolute;
left: 0;
right: 0;
margin: 0 auto;
}
</style>
2.2 宽高不定
当宽高不定时,以下测试示例:
<div class="parent">
<div class="child">测试示例</div>
</div>
<style>
.parent {
height: 100px;
background-color: aqua;
}
.child {
background-color: blueviolet;
color: #fff;
}
</style>
以下三种方式显示效果均为:
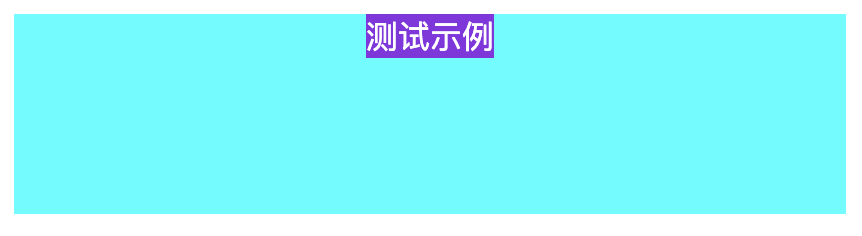
方式一:使用 CSS3 中新增的 transform 属性
<style>
.child {
position: absolute;
left: 50%;
transform:translate(-50%, 0);
}
</style>
方式二:flex 布局
<style>
.child {
display: flex;
justify-content: center;
}
</style>
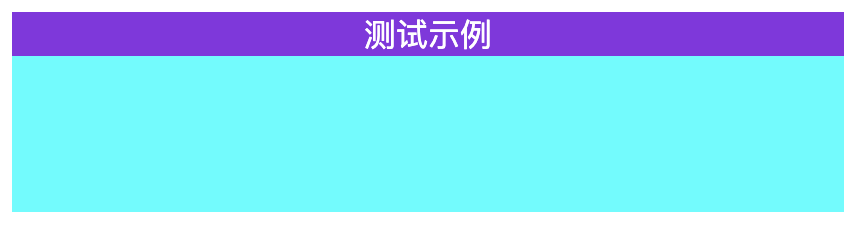
方式三:width: fit-content
<style>
.child {
width: fit-content;
margin: 0 auto;
}
</style>
fit-content
是 CSS3中 给 width
属性新加的一个属性值,它配合 margin
可以轻松实现水平居中
垂直居中
1. 行内元素
代码示例:
<div class="parent">
<span class="child">测试示例</span>
</div>
<style>
.parent {
height: 100px;
background-color: aqua;
text-align: center; /* 水平居中 */
}
.child {
color: #fff;
background-color: blueviolet;
}
</style>
方式一:line-height(单行文本)
<style>
.child {
line-height: 100px;
}
</style>
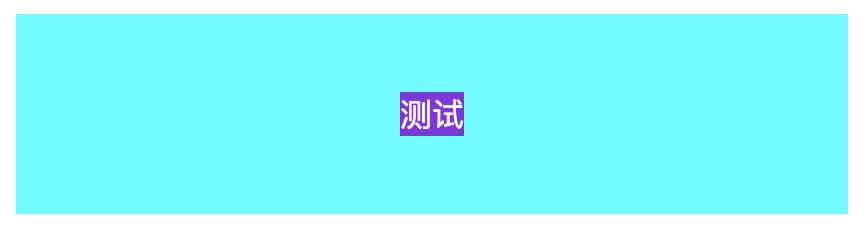
当多行时会样式错乱,需要用到 vertical-align: middle
布局
方式二:display: table-cell + vertical-align (多行文本)
可用 vertical-align 属性, 而 vertical-align
只有在父层为 td
或者 th
时, 才会生效,对于其他块级元素, 例如 div
、 p
等,默认情况是不支持的。
为了使用 vertical-align
,我们需要设置父元素 display:table
, 子元素 display:table-cell; vertical-align:middle;
<style>
.parent {
display: table;
}
.child {
display: table-cell;
vertical-align: middle;
}
</style>
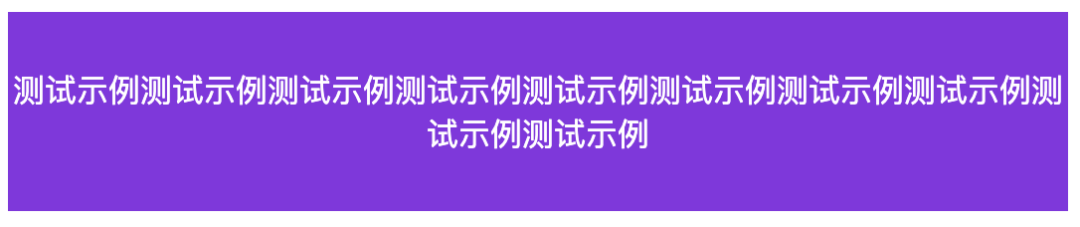
方式三:display: inline-block + vertical-align 隐式幽灵节点
设置幽灵节点的高度以及幽灵节点的基线(通过 line-height
),来设置幽灵节点的 x-height
, 是 span
的中线与幽灵节点的中线对齐,同样也可以使 vertical-align: middle;
居中
<style>
.parent {
line-height: 100px; /* 通过 line-height 设置幽灵节点的基线 */
}
.child {
vertical-align: middle;
line-height: normal; /* 块级元素时需要加 */
display: inline-block; /* 重点,要把 line-height 设置成 normal, 要不然会继承100 */
}
</style>
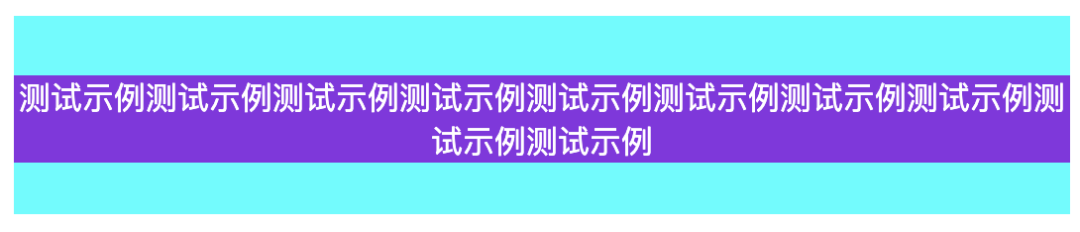
方式四:display: grid 布局
<style>
.parent {
display: grid;
}
.child {
margin: auto;
}
</style>
效果如上
方式五:writing-mode 布局
writing-mode
属性定义了文本在水平或垂直方向上如何排布。
<style>
.parent {
writing-mode: vertical-lr;
}
.child {
writing-mode: horizontal-tb;
}
</style>
效果如上
2. 块级元素
参照 水平居中的块级元素布局 ,同样把对水平方向的转换为垂直方向的
2.1 宽高固定
示例代码:
<div class="parent">
<div class="child"></div>
</div>
<style>
body {
background-color: aqua;
}
.child {
width: 50px;
height: 50px;
background-color: blueviolet;
}
</style>
以下几种方式显示效果均为:
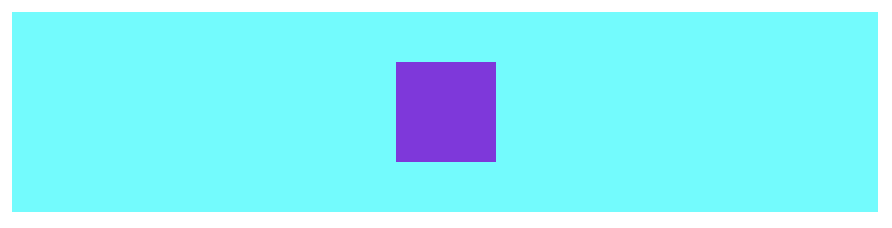
方式一:absolute + margin-top
<style>
.child {
position: absolute;
margin-left: -25px;
left: 50%;
margin-top: -25px;
top: 50%;
}
</style>
方式二:absolute + calc
<style>
.child {
position: absolute;
left: calc(50% - 25px);
top: calc(50% - 25px);
}
</style>
方式三:absolute + left + right + top + bottom
<style>
.child {
position: absolute;
left: 0;
right: 0;
top: 0;
bottom: 0;
margin: auto;
}
</style>
2.2 宽高不定
当宽高不定时,以下测试示例:
<div class="parent">
<div class="child">测试示例</div>
</div>
<style>
.parent {
height: 100px;
background-color: aqua;
}
.child {
background-color: blueviolet;
}
</style>
以下两种方式显示效果均为:
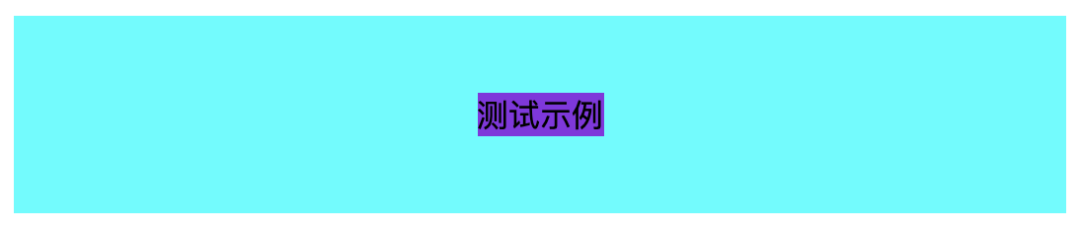
方式一:使用 CSS3 中新增的 transform 属性
需要设定 parent 为相对定位( position: relative
)
<style>
.parent {
position: relative;
}
.child {
position: absolute;
left: 50%;
top: 50px;
transform: translate(-50%, -50%);
}
</style>
方式二:flex 布局
<style>
.parent {
display: flex;
height: 100%;
justify-content: center; /* 水平居中 */
align-items: center; /* 垂直居中 */
}
</style>
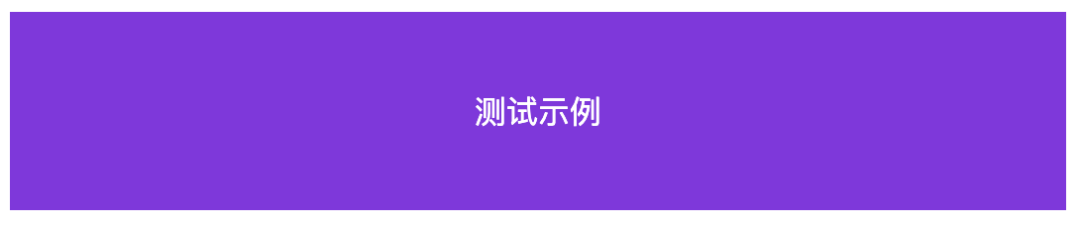
来源:https://github.com/Advanced-Frontend/Daily-Interview-Question
最后
号内回复:
120
套模版