通用Mapper快速开发,搭建项目
不一样的菜鸟
共 2791字,需浏览 6分钟
· 2021-01-28
搭建环境
配置maven依赖的架包
tk.mybatis
mapper
4.0.0-beta3
junit
junit
4.9
log4j
log4j
1.2.17
cglib
cglib
2.2
org.aspectj
aspectjweaver
1.6.8
org.slf4j
slf4j-api
1.7.7
org.slf4j
slf4j-log4j12
1.7.7
org.mybatis
mybatis
3.2.8
org.mybatis
mybatis-spring
1.2.2
com.mchange
c3p0
0.9.2
org.springframework
spring-context
4.3.10.RELEASE
mysql
mysql-connector-java
5.1.37
org.springframework
spring-orm
4.3.10.RELEASE
Spring整合mybatis
"1.0" encoding="UTF-8"?>
"-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
Spring的配置文件
"1.0" encoding="UTF-8"?>
"http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.3.xsd">
"classpath:jdbc.properties"/>
"dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
"user" value="${jdbc.user}"/>
"password" value="${jdbc.password}"/>
"jdbcUrl" value="${jdbc.url}"/>
"driverClass" value="${jdbc.driver}"/>
"sqlSessionFactoryBean" class="org.mybatis.spring.SqlSessionFactoryBean">
"configLocation" value="classpath:mybatis-config.xml"/>
"dataSource" ref="dataSource"/>
class="tk.mybatis.spring.mapper.MapperScannerConfigurer">
"basePackage" value="com.yang.mapper.mappers"/>
package="com.yang.mapper.services"/>
"dataSourceTransactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
"dataSource" ref="dataSource"/>
"txAdvice" pointcut="execution(* *..*Service.*(..))"/>
"txAdvice" transaction-manager="dataSourceTransactionManager">
"get*" read-only="true"/>
"save*" rollback-for="java.lang.Exception" propagation="REQUIRES_NEW"/>
"remove*" rollback-for="java.lang.Exception" propagation="REQUIRES_NEW"/>
"update*" rollback-for="java.lang.Exception" propagation="REQUIRES_NEW"/>
整合log4j.properties配置文件
log4j.rootLogger=DEBUG,myConsole
log4j.appender.myConsole=org.apache.log4j.ConsoleAppender
log4j.appender.myConsole.ImmediateFlush=true
log4j.appender.myConsole.Target=System.out
log4j.appender.myConsole.layout=org.apache.log4j.PatternLayout
log4j.appender.myConsole.layout.ConversionPattern=[%-5p] %d(%r) --> [%t] %l: %m %x %n
log4j.logger.com.mchange.v2=ERROR
数据库jdbc.properties配置文件
jdbc.user=root
jdbc.password=root
jdbc.url=jdbc:mysql://localhost:3306/girls?useUnicode=true&characterEncoding=utf8
jdbc.driver=com.mysql.jdbc.Driver
数据库表的设计
CREATE TABLE `tabple_emp` (
`emp_id` int NOT NULL AUTO_INCREMENT ,
`emp_name` varchar(500) NULL ,
`emp_salary` double(15,5) NULL ,
`emp_age` int NULL , PRIMARY KEY (`emp_id`)
);
INSERT INTO `tabple_emp` (`emp_name`, `emp_salary`, `emp_age`) VALUES ('tom', '1254.37', '27');
INSERT INTO `tabple_emp` (`emp_name`, `emp_salary`, `emp_age`) VALUES ('jerry', '6635.42', '38');
INSERT INTO `tabple_emp` (`emp_name`, `emp_salary`, `emp_age`) VALUES ('bob', '5560.11', '40');
INSERT INTO `tabple_emp` (`emp_name`, `emp_salary`, `emp_age`) VALUES ('kate', '2209.11', '22');
INSERT INTO `tabple_emp` (`emp_name`, `emp_salary`, `emp_age`) VALUES ('justin', '4203.15', '30');
建立实体类
@Table(name = "tabple_emp")
public class Employee {
// @Transient当数据库里面没有某个字段的时候可以用此注解
private Integer empId;
// 当数据字段和实体类字段不一致时可以用该字段
@Column(name = "emp_name")
private String empName;
private Double empSalary;
private Integer empAge;
get,set方法省略.......
}
建立mapper的dao层
package com.yang.mapper.dao;
import com.yang.mapper.entity.Employee;
import tk.mybatis.mapper.common.Mapper;
/**
* 继承Mapper<实体类
*
*/
public interface EmployeeMapper extends Mapper<Employee> {
}
简单的测试
package com.yang.mapper.services;
import com.yang.mapper.entity.Employee;
import org.apache.ibatis.session.RowBounds;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import tk.mybatis.mapper.entity.Example;
import java.util.List;
import static org.junit.Assert.*;
public class EmployeeServiceTest {
private ApplicationContext iocContainer = new ClassPathXmlApplicationContext("spring-context.xml");
private EmployeeService employeeService = iocContainer.getBean(EmployeeService.class);
@Test
public void testSelectOne() {
//1.创建封装查询条件的实体类对象
Employee employeeQueryCondition = new Employee(null, "bob", 5560.11, null);
//2.执行查询
Employee employeeQueryResult = employeeService.getOne(employeeQueryCondition);
//3.打印
System.out.println(employeeQueryResult);
}
@Test
public void testSelectByPrimaryKey() {
//1.提供id值
Integer empId = 3;
//2.执行根据主键进行的查询
Employee employee = employeeService.getEmployeeById(empId);
//3.打印结果
System.out.println(employee);
}
@Test
public void testExistsWithPrimaryKey() {
//1.提供主键值
Integer empId = 33;
//2.执行查询
boolean exists = employeeService.isExists(empId);
//3.打印结果
System.out.println(exists);
}
@Test
public void testInsert() {
//1.创建实体类对象封装要保存到数据库的数据
Employee employee = new Employee(null, "emp03", 3000.00, 23);
//2.执行插入操作
employeeService.saveEmployee(employee);
//3.获取employee对象的主键字段值
Integer empId = employee.getEmpId();
System.out.println("empId="+empId);
}
@Test
public void testInsertSelective() {
//1.创建实体类对象封装要保存到数据库的数据
Employee employee = new Employee(null, "emp04", null, 23);
//2.执行插入操作
employeeService.saveEmployeeSelective(employee);
}
@Test
public void testUpdateByPrimaryKeySelective() {
//1.创建用于测试的实体类
Employee employee = new Employee(7, "empNewName", null, null);
//2.执行更新
employeeService.updateEmployeeSelective(employee);
}
@Test
public void testDelete() {
//1.声明实体类变量作为查询条件
Employee employee = null;
//2.执行删除
employeeService.removeEmployee(employee);
}
@Test
public void testDeleteByPrimaryKey() {
//1.提供主键值
Integer empId = 13;
//2.执行删除
employeeService.removeEmployeeById(empId);
}
@Test
public void testSelectByExample() {
//目标:WHERE (emp_salary>? AND emp_age) OR (emp_salary AND emp_age>?)
//1.创建Example对象
Example example = new Example(Employee.class);
//***********************
//i.设置排序信息
example.orderBy("empSalary").asc().orderBy("empAge").desc();
//ii.设置“去重”
example.setDistinct(true);
//iii.设置select字段
example.selectProperties("empName","empSalary");
//***********************
//2.通过Example对象创建Criteria对象
Example.Criteria criteria01 = example.createCriteria();
Example.Criteria criteria02 = example.createCriteria();
//3.在两个Criteria对象中分别设置查询条件
//property参数:实体类的属性名
//value参数:实体类的属性值
criteria01.andGreaterThan("empSalary", 3000)
.andLessThan("empAge", 25);
criteria02.andLessThan("empSalary", 5000)
.andGreaterThan("empAge", 30);
//4.使用OR关键词组装两个Criteria对象
example.or(criteria02);
//5.执行查询
List empList = employeeService.getEmpListByExample(example);
for (Employee employee : empList) {
System.out.println(employee);
}
}
@Test
public void testSelectByRowBounds() {
int pageNo = 3;
int pageSize = 5;
int index = (pageNo - 1) * pageSize;
RowBounds rowBounds = new RowBounds(index, pageSize);
List empList = employeeService.getEmpListByRowBounds(rowBounds);
for (Employee employee : empList) {
System.out.println(employee);
}
}
}
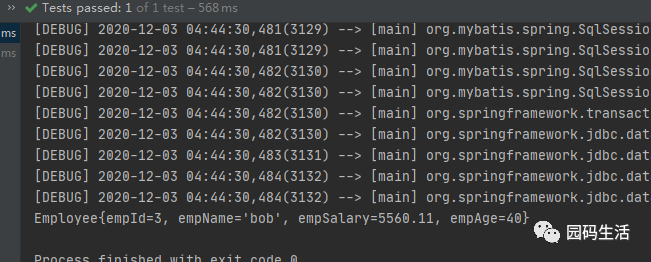
当数据库为空时可以加个注解@Id
package com.yang.mapper.entity;
import javax.persistence.Column;
import javax.persistence.Id;
import javax.persistence.Table;
//指定数据库表名
@Table(name = "tabple_emp")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer empId;
private Integer empId;
// 当数据字段和实体类字段不一致时可以用该字段
@Column(name = "emp_name")
private String empName;
private Double empSalary;
private Integer empAge;
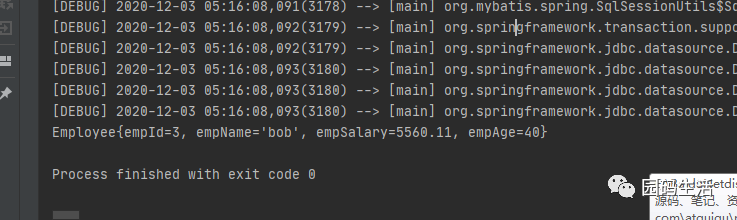
评论
MyBatis Mapper新一代通用 Mapper
项目地址:https://mapper.mybatis.io介绍这是一个不需要任何配置就可以直接使用
MyBatis Mapper新一代通用 Mapper
0
MyBatis Mapper新一代通用 Mapper
项目地址:https://mapper.mybatis.io介绍这是一个不需要任何配置就可以直接使用的通用Mapper,通过简单的学习就可以直接在项目中使用。1.1主要目标1.开箱即用,无需任何配置,
MyBatis Mapper新一代通用 Mapper
0
common-mappermybatis 通用 mapper
简介mybatis通用mapper,支持简单表关系注解配置快速使用目前没有发布到中心仓库,可以把项目克隆下来,用maven编译为jar包使用maven本地引入。<dependency>&l
common-mappermybatis 通用 mapper
0
common-mappermybatis 通用 mapper
简介mybatis 通用 mapper,支持简单表关系注解配置快速使用目前没有发布到中心仓库,可以把
common-mappermybatis 通用 mapper
0
MapperMybatis 通用 Mapper
通用Mapper4是一个可以实现任意MyBatis通用方法的框架,项目提供了常规的增删改查操作以及Example 相关的单表操作。通用Mapper是为了解决MyBatis使用中90%的基本操作,使用它
MapperMybatis 通用 Mapper
0
general-biz基于通用 mapper 的通用 biz 基础业务实现技术
general-biz是基于通用mapper的通用biz基础业务实现技术0.技术概述 一般而言,java项目一般都会有controller、service和Dao层,根据业务复杂度加大,
general-biz基于通用 mapper 的通用 biz 基础业务实现技术
0