【每日一题】用for实现forEach
人生苦短,总需要一点仪式感。比如学前端~
forEach
语法:
arr.forEach(callback(currentValue [, index [, array]])[, thisArg])
// 完整的参数使用,比如:
arr.forEach(function (currentValue, index, array) {
console.log(this, currentValue, index, array)
}, thisArg)
参数
callback
为数组中每个元素执行的函数,该函数接收一至三个参数:
currentValue
数组中正在处理的当前元素。
index 可选
数组中正在处理的当前元素的索引。
array 可选
forEach() 方法正在操作的数组。
thisArg 可选
可选参数。当执行回调函数 callback
时,用作 this 的值。
若不传该参数,非严格模式下,this默认指向window;非非严格模式下,this默认指向undefined。
实现
Array.prototype.myForEach = function (fn, context = undefined) { // 将myForEach函数挂载到Array原型上
if (typeof fn !== "function") { // 判断,调用myForEach时传入的是否是函数
throw new TypeError(fn + "is not a function"); // 不是函数则抛出类型错误
}
for (let i = 0; i < this.length; i++) { // this指向调用myForEach的数组,使用for循环遍历这个目标数组
fn.call(context || Window, this[i], i, this); // 使用call调用该回调函数,为了是修改回调函数内部的this。
}
};
测试:非严格模式下,this默认指向window
注意:测试this,callback千万记得别用错成了箭头函数。否则测不对
var arr = [3, 4, 5, 6];
arr.myForEach(function (item, index) {
console.log(this, item, index, "==普通使用==");
});
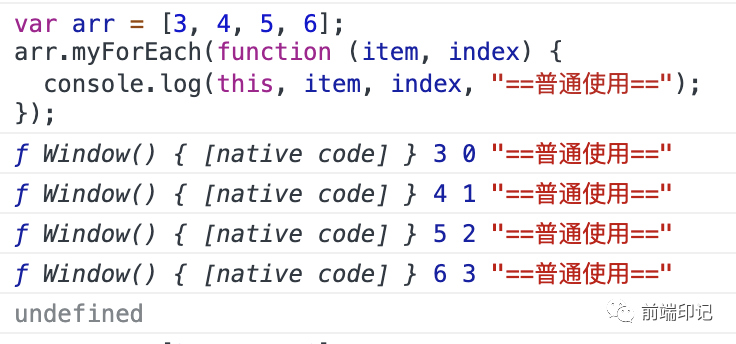
const thisArg = {name: 'this指向本对象'}
arr.myForEach(function(item, index) { // 注意这里别用箭头函数,不然函数内部this永远指向上个非箭头作用域的this
console.log(this, item, index, '==this指向==')
}, thisArg)
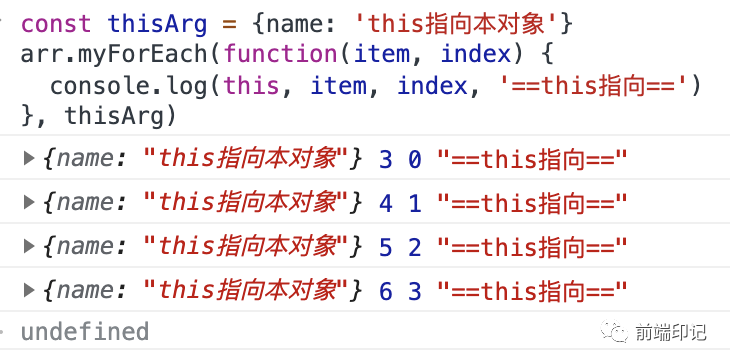
while 版本
代码:
Array.prototype.myForEach = function (callback, thisArg) {
if (this == null) {
throw new TypeError("this is null or not defined");
}
if (typeof callback !== "function") {
throw new TypeError(callback + "is not a function");
}
let obj = Object(this),
len = obj.length;
idx = 0;
// 得到目标数组的长度,进行while循环
while (idx < len) {
let value;
if (idx in obj) {
value = obj[idx];
callback.call(thisArg, value, idx, obj);
}
idx++;
}
};
var a = [3, 4, 5, 6];
a.myForEach((item, index) => {
console.log(item, index, "====");
});
测试:
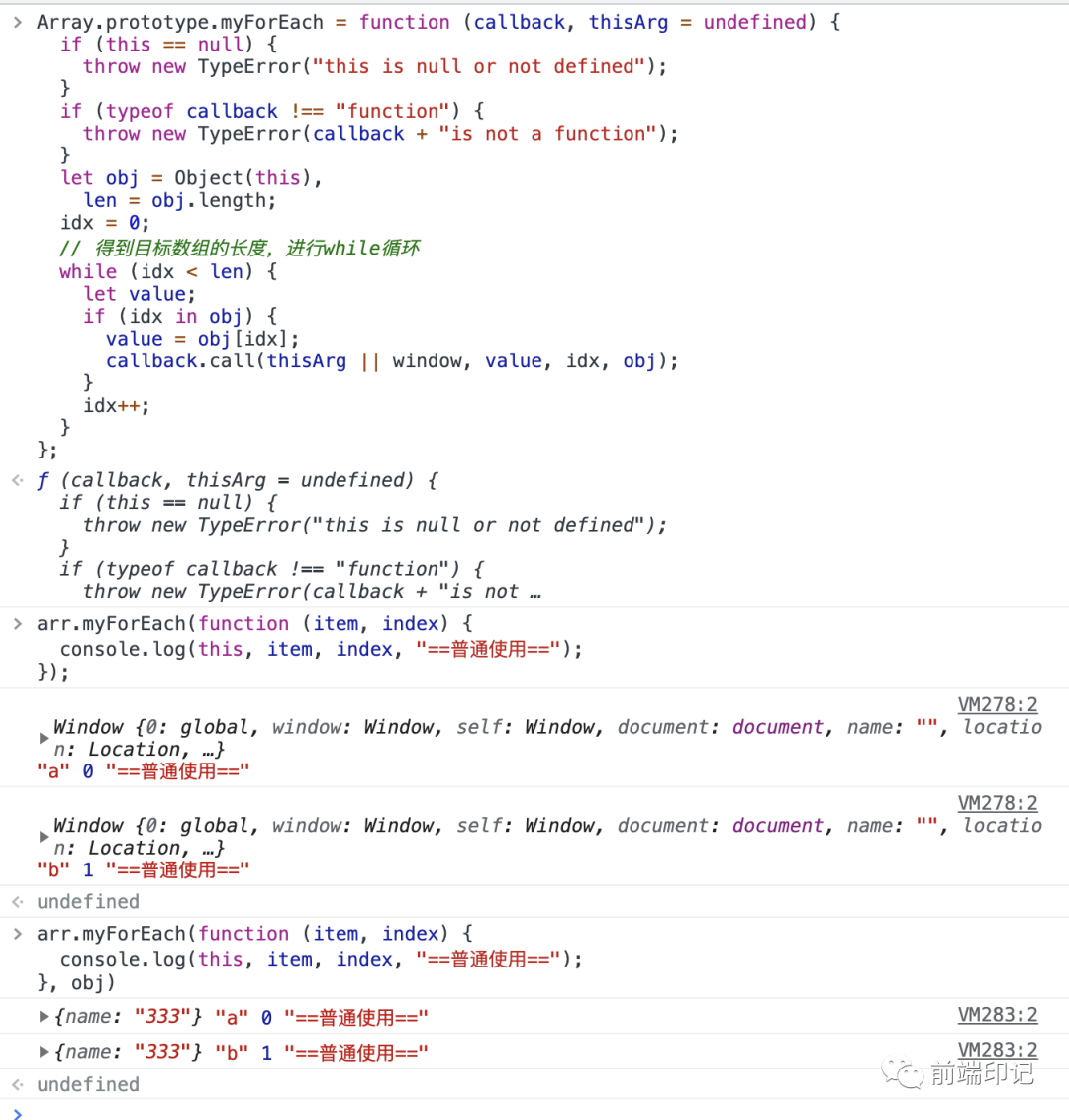

让我们一起携手同走前端路!
关注公众号回复【加群】即可

评论