Java8 Stream常用API整理(值得收藏)
共 7918字,需浏览 16分钟
·
2020-08-21 19:55
题 图:pexels
作 者:程铭程铭你快成名
来 源:https://blog.csdn.net/wangchengming1/article/details/89245402
Java8中有两大最为重要的改变,第一个是Lambda表达式,另外一个则是Stream API。
流是 Java8 引入的全新概念,它用来处理集合中的数据。
众所周知,集合操作非常麻烦,若要对集合进行筛选、投影,需要写大量的代码,而流是以声明的形式操作集合,它就像 SQL 语句,我们只需告诉流需要对集合进行什么操作,它就会自动进行操作,并将执行结果交给你,无需我们自己手写代码。
在项目中使用 Stream API 可以大大提高效率以及代码的可读性,使我们对数据进行处理的时候事半功倍。
这篇博文以实战角度出发,讲解平时开发中常用 API 的用法以及一些注意的地方。
首先创建一个对象
public class Employee {
private int id;
private String name;
private int age;
private double salary;
private Status status;
public enum Status {
FREE, BUSY, VOCATION;
}
public Employee() {
}
public Employee(String name) {
this.name = name;
}
public Employee(String name, int age) {
this.name = name;
this.age = age;
}
public Employee(int id, String name, int age, double salary) {
this.id = id;
this.name = name;
this.age = age;
this.salary = salary;
}
public Employee(int id, String name, int age, double salary, Status status) {
this.id = id;
this.name = name;
this.age = age;
this.salary = salary;
this.status = status;
}
//省略get,set等。。。
}
随便初始化一些数据
List
empList = Arrays.asList(new Employee(102, "李四", 59, 6666.66, Status.BUSY),
new Employee(101, "张三", 18, 9999.99, Status.FREE), new Employee(103, "王五", 28, 3333.33, Status.VOCATION),
new Employee(104, "赵六", 8, 7777.77, Status.BUSY), new Employee(104, "赵六", 8, 7777.77, Status.FREE),
new Employee(104, "赵六", 8, 7777.77, Status.FREE), new Employee(105, "田七", 38, 5555.55, Status.BUSY));
中间操作
根据条件筛选 filter
/**
* 接收Lambda, 从流中排除某些元素。
*/@Test
void testFilter() {
empList.stream().filter((e) -> {
return e.getSalary() >= 5000;
}).forEach(System.out::println);
}
跳过流的前n个元素 skip
/**
* 跳过元素,返回一个扔掉了前n个元素的流。
*/
@Test
void testSkip() {
empList.stream().filter((e) -> e.getSalary() >= 5000).skip(2).forEach(System.out::println);
}
去除重复元素 distinct
/**
* 筛选,通过流所生成元素的 hashCode() 和 equals() 去除重复元素
*/
@Test
void testDistinct() {
empList.stream().distinct().forEach(System.out::println);
}
截取流的前n个元素 limit
/**
* 截断流,使其元素不超过给定数量。
*/
@Test
void testLimit() {
empList.stream().filter((e) -> {
return e.getSalary() >= 5000;
}).limit(3).forEach(System.out::println);
}
映射 map
/**
* 接收一个函数作为参数,该函数会被应用到每个元素上,并将其映射成一个新的元素
*/
@Test
void testMap() {
empList.stream().map(e -> e.getName()).forEach(System.out::println);
empList.stream().map(e -> {
empList.forEach(i -> {
i.setName(i.getName() + "111");
});
return e;
}).collect(Collectors.toList());
}
自然排序 sorted
/**
* 产生一个新流,其中按自然顺序排序
*/
@Test
void testSorted() {
empList.stream().map(Employee::getName).sorted().forEach(System.out::println);
}
自定义排序 sorted(Comparator comp)
/**
* 产生一个新流,其中按自然顺序排序
*/
@Test
void testSortedComparator() {
empList.stream().sorted((x, y) -> {
if (x.getAge() == y.getAge()) {
return x.getName().compareTo(y.getName());
} else {
return Integer.compare(x.getAge(), y.getAge());
}
}).forEach(System.out::println);
}
最终操作
是否匹配任一元素 anyMatch
/**
* 检查是否至少匹配一个元素
*/
@Test
void testAnyMatch() {
boolean b = empList.stream().anyMatch((e) -> e.getStatus().equals(Status.BUSY));
System.out.println("boolean is : " + b);
}
是否匹配所有元素 allMatch
/**
* 检查是否匹配所有元素
*/
@Test
void testAllMatch() {
boolean b = empList.stream().allMatch((e) -> e.getStatus().equals(Status.BUSY));
System.out.println("boolean is : " + b);
}
/**
* 检查是否没有匹配的元素
*/
@Test
void testNoneMatch() {
boolean b = empList.stream().noneMatch((e) -> e.getStatus().equals(Status.BUSY));
System.out.println("boolean is : " + b);
}
返回第一个元素 findFirst
/**
* 返回第一个元素
*/
@Test
void testFindFirst() {
Optionalop = empList.stream().sorted((e1, e2) -> Double.compare(e1.getSalary(), e2.getSalary()))
.findFirst();
if (op.isPresent()) {
System.out.println("first employee name is : " + op.get().getName().toString());
}
}
/**
* 返回当前流中的任意元素
*/
@Test
void testFindAny() {
Optionalop = empList.stream().sorted((e1, e2) -> Double.compare(e1.getSalary(), e2.getSalary()))
.findAny();
if (op.isPresent()) {
System.out.println("any employee name is : " + op.get().getName().toString());
}
}
返回流的总数 count
/**
* 返回流中元素的总个数
*/
@Test
void testCount() {
long count = empList.stream().filter((e) -> e.getStatus().equals(Status.FREE)).count();
System.out.println("Count is : " + count);
}
返回流中的最大值 max
/**
* 返回流中最大值
*/
@Test
void testMax() {
Optionalop = empList.stream().map(Employee::getSalary).max(Double::compare);
System.out.println(op.get());
}
返回流中的最小值 min
/**
* 返回流中最小值
*/
@Test
void testMin() {
Optionalop2 = empList.stream().min((e1, e2) -> Double.compare(e1.getSalary(), e2.getSalary()));
System.out.println(op2.get());
}
归约 reduce
归约是将集合中的所有元素经过指定运算,折叠成一个元素输出
/**
* 可以将流中元素反复结合起来,得到一个值。返回T
*/
@Test
void testReduce() {
Optionalop = empList.stream().map(Employee::getSalary).reduce(Double::sum);
System.out.println(op.get());
}
/**
* 可以将流中元素反复结合起来,得到一个值,返回Optional< T>
*/
@Test
void testReduce1() {
Optionalsum = empList.stream().map(Employee::getName).flatMap(Java8Stream::filterCharacter)
.map((ch) -> {
if (ch.equals('六'))
return 1;
else
return 0;
}).reduce(Integer::sum);
System.out.println(sum.get());
}
将元素收集到 list 里 Collectors.toList()
/**
* 把流中的元素收集到list里。
*/
@Test
void testCollectorsToList() {
Listlist = empList.stream().map(Employee::getName).collect(Collectors.toList());
list.forEach(System.out::println);
}
将元素收集到 set 里 Collectors.toSet()
/**
* 把流中的元素收集到set里。
*/
@Test
void testCollectorsToSet() {
Setlist = empList.stream().map(Employee::getName).collect(Collectors.toSet());
list.forEach(System.out::println);
}
把流中的元素收集到新创建的集合里 Collectors.toCollection(HashSet::new)
/**
* 把流中的元素收集到新创建的集合里。
*/
@Test
void testCollectorsToCollection() {
HashSeths = empList.stream().map(Employee::getName).collect(Collectors.toCollection(HashSet::new));
hs.forEach(System.out::println);
}
根据比较器选择最大值 Collectors.maxBy()
/**
* 根据比较器选择最大值。
*/
@Test
void testCollectorsMaxBy() {
Optionalmax = empList.stream().map(Employee::getSalary).collect(Collectors.maxBy(Double::compare));
System.out.println(max.get());
}
根据比较器选择最小值 Collectors.minBy()
/**
* 根据比较器选择最小值。
*/
@Test
void testCollectorsMinBy() {
Optionalmax = empList.stream().map(Employee::getSalary).collect(Collectors.minBy(Double::compare));
System.out.println(max.get());
}
对流中元素的某个字段求和 Collectors.summingDouble()
/**
* 对流中元素的整数属性求和。
*/
@Test
void testCollectorsSummingDouble() {
Double sum = empList.stream().collect(Collectors.summingDouble(Employee::getSalary));
System.out.println(sum);
}
对流中元素的某个字段求平均值 Collectors.averagingDouble()
/**
* 计算流中元素Integer属性的平均值。
*/
@Test
void testCollectorsAveragingDouble() {
Double avg = empList.stream().collect(Collectors.averagingDouble(Employee::getSalary));
System.out.println(avg);
}
分组,类似sql的 group by Collectors.groupingBy
/**
* 分组
*/
@Test
void testCollectorsGroupingBy() {
Map> map = empList.stream().collect(Collectors.groupingBy(Employee::getStatus));
System.out.println(map);
}
/**
* 多级分组
*/
@Test
void testCollectorsGroupingBy1() {
Map>> map = empList.stream()
.collect(Collectors.groupingBy(Employee::getStatus, Collectors.groupingBy((e) -> {
if (e.getAge() >= 60)
return "老年";
else if (e.getAge() >= 35)
return "中年";
else
return "成年";
})));
System.out.println(map);
}
字符串拼接 Collectors.joining()
/**
* 字符串拼接
*/
@Test
void testCollectorsJoining() {
String str = empList.stream().map(Employee::getName).collect(Collectors.joining(",", "----", "----"));
System.out.println(str);
}
public static Stream
filterCharacter(String str) {
Listlist = new ArrayList<>();
for (Character ch : str.toCharArray()) {
list.add(ch);
}
return list.stream();
}
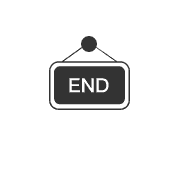
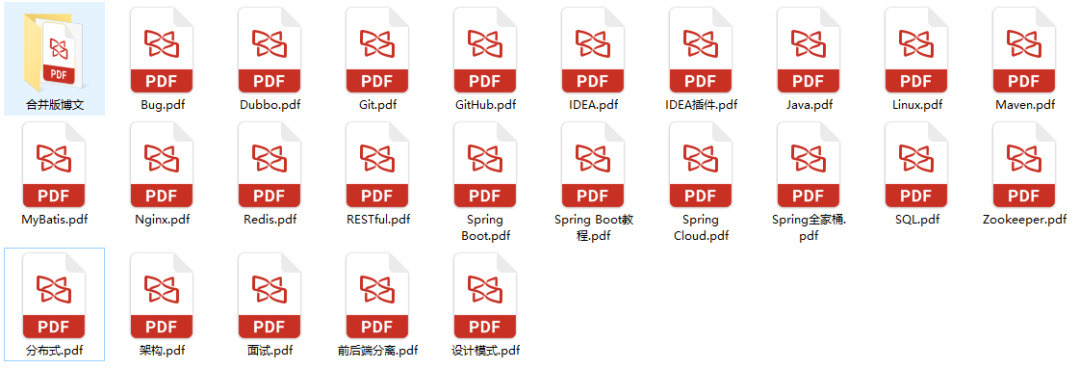
下载方式
1. 首先扫描下方二维码
2. 后台回复「555」即可获取
注明:仅仅作为知识分享,切勿用于其它商业活动 。感谢所有技术分享者的付出。
点赞是最大的支持