用canvas 实现矩形的移动(点、线、面)
关注并将「趣谈前端」设为星标
每早08:30按时推送技术干货/优秀开源/技术思维
前言
面的移动
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const width = 100;
const height = 100;
drawRect();
function drawRect(x = 10,y = 10, scale = 1) {
ctx.clearRect( 0, 0, 1800, 800 );
const halfwidth = width * scale / 2;
const halfheight = height * scale / 2;
ctx.strokeRect(x - halfwidth, y - halfheight,width * scale, height * scale)
}
let isMove = true;
canvas.addEventListener('mousemove',(e)=>{
if(!isMove) {
return
}
const x = e.clientX;
const y = e.clientY;
drawRect(x,y)
})
canvas.addEventListener('click',(e)=> {
isMove = !isMove;
});
点的移动
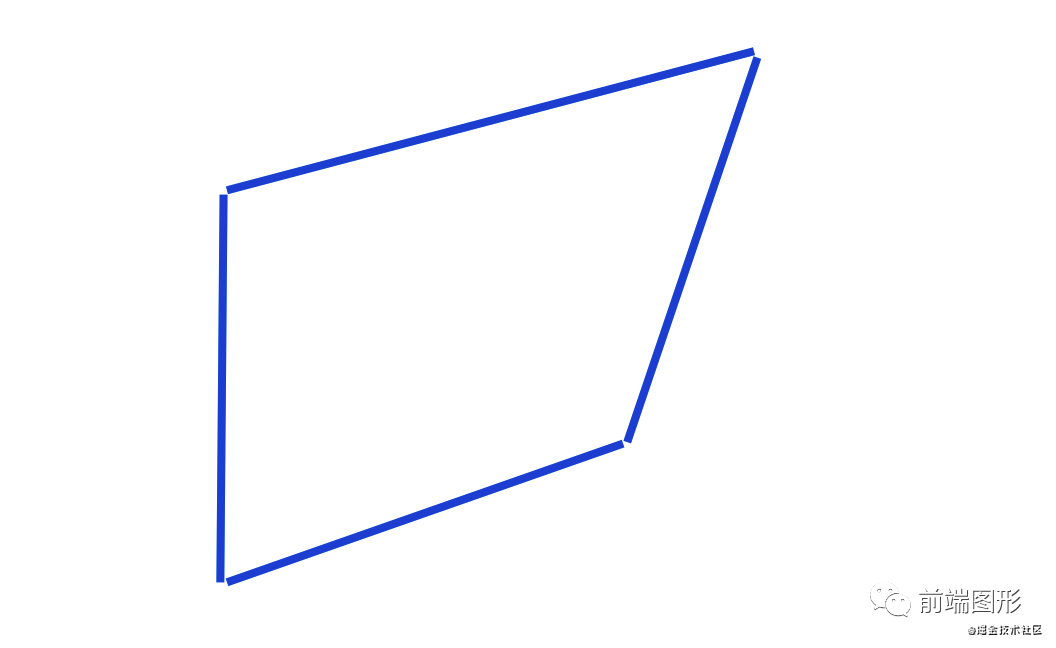
class Point2d {
constructor(x,y) {
this.x = x || 0;
this.y = y || 0;
}
clone() {
return this.constructor(this.x, this.y);
}
add(v) {
this.x += v.x;
this.y += v.y
return this;
}
random() {
this.x = Math.random() *1800;
this.y = Math.random() * 800;
return this
}
}
function drawFourPolygon(x, y ,width = 50, height = 50) {
ctx.clearRect( 0, 0, 1800, 800 );
ctx.beginPath();
ctx.moveTo(x- width /2, y - height/2)
ctx.lineTo(x+ width / 2, y -height/2 )
ctx.lineTo(x+ width / 2, y + height/2 )
ctx.lineTo(x - width / 2, y + height/2 )
ctx.closePath()
ctx.stroke()
}
function drawDashLine(start, end) {
if (!start || !end) {
return
}
ctx.strokeStyle = 'red';
ctx.setLineDash( [5, 10] );
ctx.beginPath();
ctx.moveTo( start.x, start.y );
ctx.lineTo( end.x, end.y );
ctx.closePath()
ctx.stroke();
}
ctx.setLineDash([]);
const moveVec = end.clone().sub(start);
const rightTop = new Point2d(x+ width / 2, y - height/2).clone().add(moveVec)
ctx.lineTo(isSelect ? rightTop.x : x+ width / 2, isSelect ? rightTop.y : y height/2)
// 看下click和move 事件 开关就是isSelect这个变量
canvas.addEventListener('mousemove',(e)=>{
if(!isMove) {
return
}
const x = e.clientX;
const y = e.clientY;
clearRect();
end = new Point2d(x,y);
drawDashLine(start,end);
drawFourPolygon(start)
})
canvas.addEventListener('click',(e)=> {
// 这是一个每次清除画布的函数
clearRect()
isMove = !isMove;
const x = e.clientX;
const y = e.clientY;
start = new Point2d(x,y);
drawFourPolygon(start)
isSelect = true;
});
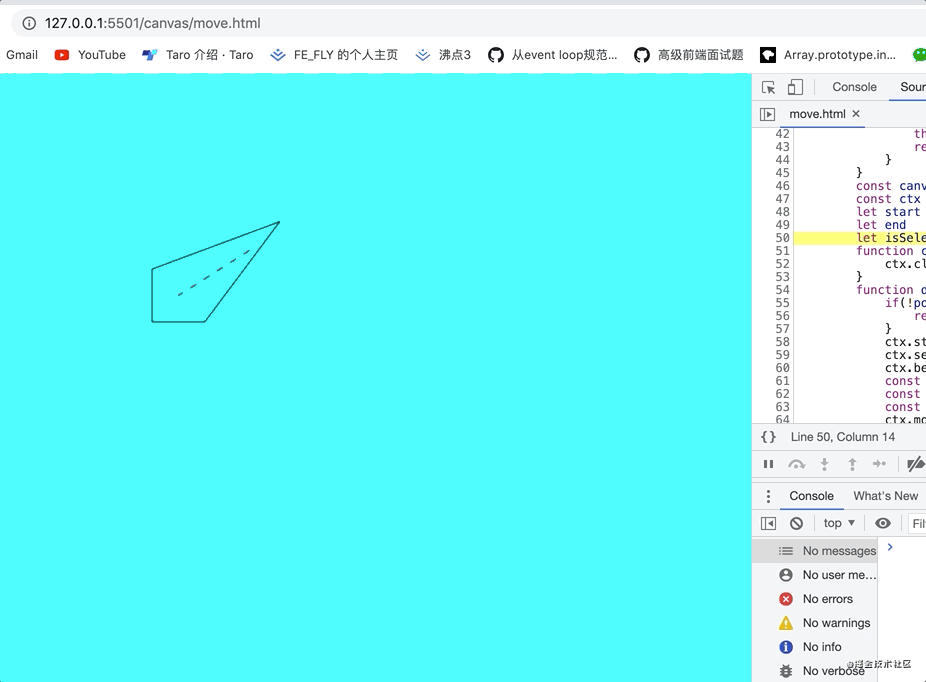
线的移动
function drawFourPolygon( point, width = 50, height = 50) {
if(!point) {
return
}
ctx.strokeStyle = 'black'
ctx.setLineDash([]);
ctx.beginPath();
const { x, y } = point;
const moveVec = end.clone().sub(start);
// 其实就是 右上和右下这两个点同时移动
const rightTop = new Point2d(x+ width / 2, y - height/2).clone().add(moveVec)
const rightBottom = new Point2d(x+ width / 2, y + height/2).clone().add(moveVec)
ctx.moveTo(x- width /2, y - height/2)
ctx.lineTo(isSelect ? rightTop.x : x+ width / 2, isSelect ? rightTop.y : y - height/2)
ctx.lineTo(isSelect ? rightBottom.x : x+ width / 2, isSelect ? rightBottom.y : y + height/2)
ctx.lineTo(x - width / 2, y + height/2 )
ctx.closePath()
ctx.stroke()
}
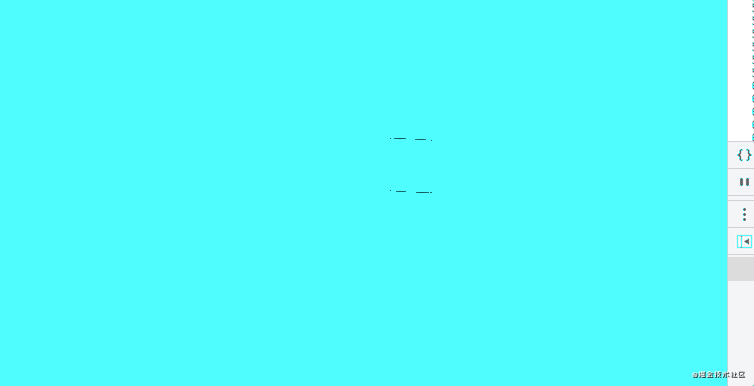
总结
❤️ 看完三件事
如果你觉得这篇内容对你挺有启发,我想邀请你帮我三个小忙:
点个【在看】,或者分享转发,让更多的人也能看到这篇内容
关注公众号【趣谈前端】,定期分享 工程化 / 可视化 / 低代码 / 优秀开源。
基于Koa + React + TS从零开发全栈文档编辑器(进阶实战)
点个在看你最好看

评论