LeetCode刷题实战142: 环形链表 II
共 2339字,需浏览 5分钟
·
2021-01-04 20:30
Given a linked list, return the node where the cycle begins. If there is no cycle, return null.
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer. Internally, pos is used to denote the index of the node that tail's next pointer is connected to. Note that pos is not passed as a parameter.
Notice that you should not modify the linked list.
题意
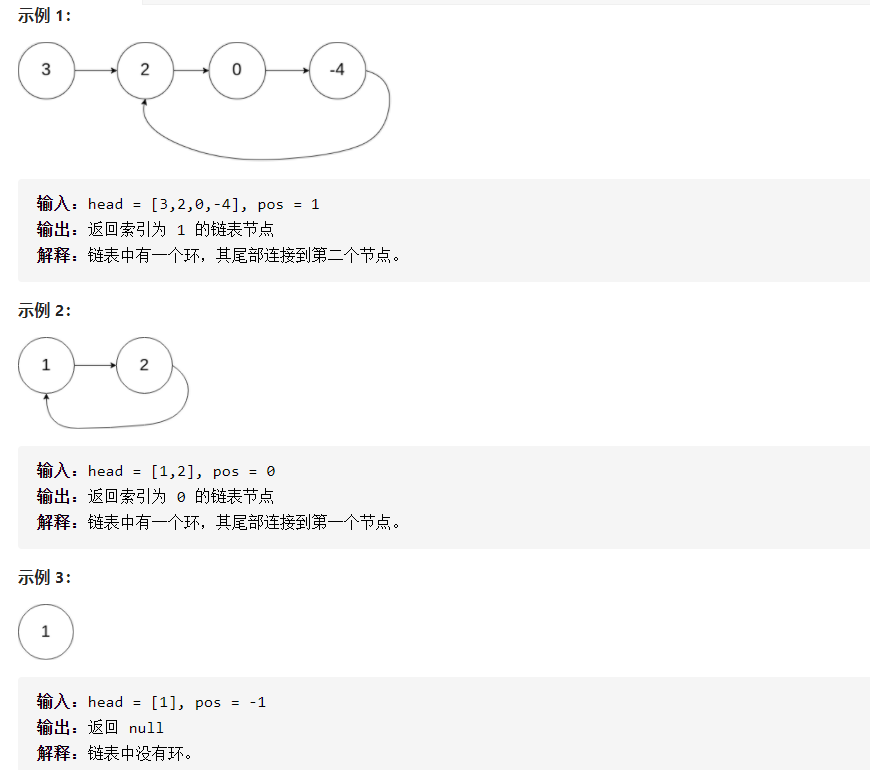
解题
思路:用快慢双指针遍历链表
public class Solution {
public ListNode detectCycle(ListNode head) {
ListNode dummyHead = new ListNode(-1);
dummyHead.next = head;
ListNode cur1 = dummyHead;
ListNode cur2 = dummyHead;
while(true){
if(null == cur2.next || null == cur2.next.next){
return null;
}
cur1 = cur1.next;
cur2 = cur2.next.next;
if(cur1 == cur2){
break;
}
}
cur1 = dummyHead;
while(cur1 != cur2){
cur1 = cur1.next;
cur2 = cur2.next;
}
return cur1;
}
}