拥抱 Vue 3 系列之 JSX 语法
“别再更新了,学不动了”。这句话不知道出了多少开发者的辛酸。在过去的一年中,Vue 团队一直都在开发 Vue.js 的下一个主要版本,就在 6 月底,尤大更新同步了 Vue 3 及其周边生态的状态:Vue 3: mid 2020 status update (https://github.com/vuejs/rfcs/issues/183)。
if (isTrue("I am planning to use Vue 3 for a new project")) {
if (isTrue("I need IE11 support")) {
await IE11CompatBuild() // July 2020
}
if (isTrue("RFCs are too dense, I need an easy-to-read guide")) {
await migrationGuide() // July 2020
}
if (isTrue("I'd rather wait until it's really ready") {
await finalRelease() // Targeting early August 2020
})
run(`npm init vite-app hello-vue3`)
return
}
我们可以看到,如果一切顺利的话,预计在 8 月份,Vue 3 的正式版本就可以和我们见面了,目前距离发布正式版还有一定的差距,还要做一些兼容性的工作。同时还会提供对 IE11 的支持。
全面拥抱 TypeScript
重构 complier
重构 Virtual DOM
......
写在前面
比如当开始写一个只能通过 level
prop 动态生成标题 (heading) 的组件时,你可能很快想到这样实现:
<script type="text/x-template" id="anchored-heading-template">
if
="level === 1">
<slot>slot>
h1>
<h2 v-else-if="level === 2">
<slot>slot>
h2>
<h3 v-else-if="level === 3">
<slot>slot>
h3>
script>
这里用模板并不是最好的选择,在每一个级别的标题中重复书写了
,不够优雅。
const App = {
render() {
const tag = `h${this.level}`
return <tag>{this.$slots.default}tag>
}
}
Vue JSX 简介
const el = <div>Vue 3div>;
这段代码既不是 HTML 也不是字符串,被称之为 JSX,是 JavaScript 的扩展语法。JSX 可能会使人联想到模板语法,但是它具备 Javascript 的完全变成能力。
<div id="app">{{ msg }}div>
function render() {
with(this) {
return _c('div', {
attrs: {
"id": "app"
}
}, [_v(_s(msg))])
}
}
观察上述代码我们发现,到运行阶段实际上都是 render 函数在执行。Vue 推荐在绝大多数情况下使用 template 来创建你的 HTML。然而在一些场景中,就需要使用 render 函数,它比 template 更加灵活。
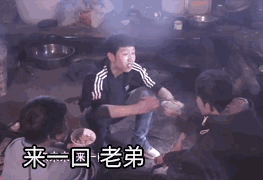
使用过 React 的同学对于如何写 JSX 语法一定非常熟悉了,然而,Vue 2 中 的 JSX 写法和 React 还是有一些略微的区别。React 中所有传递的数据都挂在顶层。
const App = <A className="x" style={style} onChange={onChange} />
props
,普通 html 属性 attrs
,DOM 属性 domProps
。想要更多了解如何在 Vue 2 中写 JSX 语法,可以看这篇,在 Vue 中使用 JSX 的正确姿势 (https://zhuanlan.zhihu.com/p/37920151)。Vue 3 中对 JSX 带来的改变
属性传递
// before
{
class: ['foo', 'bar'],
style: { color: 'red' },
attrs: { id: 'foo' },
domProps: { innerHTML: '' },
on: { click: foo },
key: 'foo'
}
// after
{
class: ['foo', 'bar'],
style: { color: 'red' },
id: 'foo',
innerHTML: '',
onClick: foo,
key: 'foo'
}
指令改版
v-model
、v-show
这些 API 全部通过模块导出的方式来引入“基线体积:无法舍弃的代码体积
我们来看一段非常简单的代码 ,在 Vue 2 和 Vue 3 中的编译结果有何不同
// before
function render() {
with(this) {
return _c('input', {
directives: [{
name: "model",
rawName: "v-model",
value: (x),
expression: "x"
}],
domProps: {
"value": (x)
},
on: {
"input": function ($event) {
if ($event.target.composing) return;
x = $event.target.value
}
}
})
}
}
// after
import { vModelText as _vModelText, createVNode as _createVNode, withDirectives as _withDirectives, openBlock as _openBlock, createBlock as _createBlock } from "vue"
export function render(_ctx, _cache) {
return _withDirectives((_openBlock(), _createBlock("input", {
"onUpdate:modelValue": $event => (_ctx.x = $event)
}, null, 8 /* PROPS */, ["onUpdate:modelValue"])), [
[_vModelText, _ctx.x]
])
}
可以看到在 Vue 3 中,对各个 API 做了更加细致的拆分,理想状态下,用户可以在构建时利用摇树优化 (tree-shaking) 去掉框架中不需要的特性,只保留自己用到的特性。模版编译器会生成适合做 tree-shaking 的代码,不需要使用者去关心如何去做,这部分的改动同样需要在 JSX 写法中实现。
模板编译器中增加了 PatchFlag
,在 JSX 的编译过程同样也做了处理,性能会有提升,但是考虑到 JSX 的灵活性,做了一些兼容处理,该功能还在测试阶段。
从 Vue 2 到 Vue 3 的过渡
Vue 3 虽然引入了一部分破坏性的更新,但对于绝大多数 Vue 2 的 API 还是兼容的。那么同样的,我们也要尽可能让使用 JSX 的用户通过最小的成本升级到 Vue 3,这是一个核心的目标。写这篇文章的时候,antdv 已经使用 @ant-design-vue/babel-plugin-jsx (https://github.com/vueComponent/ant-design-vue) 重构了大约 70% 的功能,预计会在 Vue 3 正式版之前发布测试版,大概率会是东半球最快兼容 Vue 3 的企业级组件库。
Vue 3 JSX 的 API 设计
函数式组件
const App = () => <div>Vue 3 JSXdiv>
普通组件
const App = {
render() {
return <div>Vue 3.0div>
}
}
const App = defineComponent(() => {
const count = ref(0);
const inc = () => {
count.value++;
};
return () => (
<div onClick={inc}>
{count.value}
div>
)
})
Fragment
const App = () => (
<>
<span>I'mspan>
<span>Fragmentspan>
)
Fragment 参考 React 的写法,尽可能写起来更加方便
Attributes/Props
const App = () => <input type="email" />
const placeholderText = 'email'
const App = () => (
<input
type="email"
placeholder={placeholderText}
/>
)
指令
“建议在 JSX 中使用驼峰 (
vModel
),但是v-model
也能用
v-show
const App = {
data() {
return { visible: true };
},
render() {
return <input vShow={this.visible} />;
},
};
v-model
“修饰符:使用 (
_
) 代替 (.
) (vModel_trim={this.test}
)
export default {
data: () => ({
test: 'Hello World',
}),
render() {
return (
<>
{this.test}
)
},
}
自定义指令
const App = {
directives: { antRef },
setup() {
return () => (
<a
vAntRef={(ref) => { this.ref = ref; }}
/>
);
},
}
插槽
关于指令、插槽最终的 API 还在讨论中,有想法的可以去留言。Vue 3 JSX Design (https://github.com/vuejs/jsx/issues/141)
Vue 2 的 JSX 写法如何快速迁移到 Vue 3
{
"plugins": ["@ant-design-vue/babel-plugin-jsx", { "transformOn": true, "compatibleProps": true }]
}
transformOn
on: { click: xx }
写法的兼容,在运行时中会转为 onClick: xxx
compatibleProps
props
、attrs
这些都不存在了,因此如果设置了这个属性为 true
,在运行时也会被解构到第一层的属性中。createVNode
的第二个参数,都会包一个 compatibleProps
和 transformOn
方法,所以酌情开启这两个参数。对于使用 Vue 2 的 JSX 同学,如果没有使用到比较”不为人知“ 的 API的情况下,都可以快速得迁移。compatibleProps
势必不太优雅,因此没有选择开启这个两个开关。这里插一句,目前 antdv 的迁移还在进行中,相关的进度都在这个 issue 里面:Vue 3 支持 (https://github.com/vueComponent/ant-design-vue/issues/1913),有兴趣的同学可以关注下,提一些 PR 过去。
如果是通过对象来传递的属性,只需要把原有分散在 props
、on
、attrs
中的值直接铺开即可。
const vcUploadProps = {
- props: {
- ...this.$props,
- prefixCls,
- beforeUpload: this.reBeforeUpload,
- },
- on: {
- start: this.onStart,
- error: this.onError,
- progress: this.onProgress,
- success: this.onSuccess,
- reject: this.onReject,
- },
+ ...this.$props,
+ prefixCls,
+ beforeUpload: this.reBeforeUpload,
+ onStart: this.onStart,
+ onError: this.onError,
+ onProgress: this.onProgress,
+ onSuccess: this.onSuccess,
+ onReject: this.onReject,
+ ref: 'uploadRef',
+ attrs: this.$attrs,
+ ...this.$attrs,
};
但是关于 inheritAttrs
有个较为底层的变动,需要开发者根据实际情况去修改。什么是inheritAttrs? (https://cn.vuejs.org/v2/api/index.html#inheritAttrs) 在 Vue 2 中,这个选项不影响 class
和 style
绑定,但是在 Vue 3 中会影响到。因此可能在属性的传递上,需要额外对这两个参数做处理。
emit
来触发。例如声明了 onClick
事件,仍然可以使用 emit('click')
。Vue 3 对 context 的 API 也做了改动,一般如果不是复杂的组件,不会涉及到这个 API。这部分的改动可以看原先 Vue Compositon API 的相关文档,Dependency Injection (https://composition-api.vuejs.org/api.html#dependency-injection),注意一点,在 setup 中取不到 this
。
总结
Ant Design Vue https://github.com/vueComponent/ant-design-vue
@ant-design-vue/babel-plugin-jsx https://github.com/vueComponent/jsx
❤️ 看完三件事
点个「在看」,让更多的人也能看到这篇内容(喜欢不点在看,都是耍流氓 -_-)
关注我的官网 https://muyiy.cn,让我们成为长期关系
关注公众号「高级前端进阶」,公众号后台回复「面试题」 送你高级前端面试题,回复「加群」加入面试互助交流群