27个重要的JavaScript数组函数整理汇总
web前端开发
共 13130字,需浏览 27分钟
·
2021-12-29 03:58
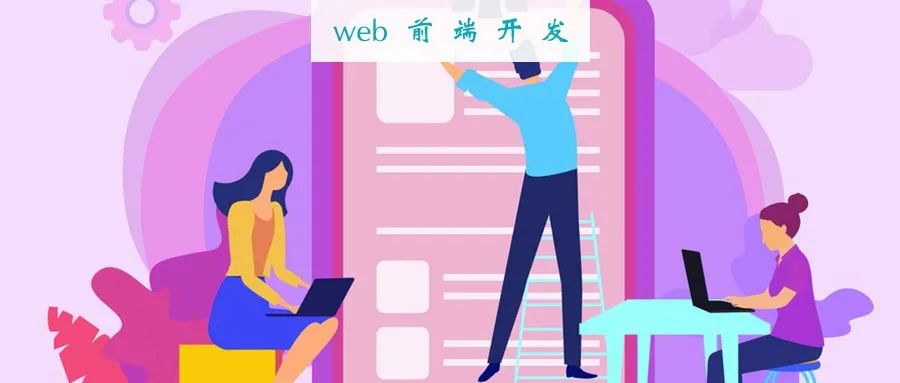
Array.isArray(arr)
Array.isArray([1, 'something', true]);
// true
Array.isArray({anything: 123});
// false
Array.isArray('some string');
// false
Array.isArray(undefined);
// false
arr.length;
[].length;
// 0
[1, 'something', true].length;
// 3
arr.forEach(callbackFn);
const callbackFn = (arrItem, index) => {callbackFn Scope Code Exec}
// combining above statements together into one line of code
arr.forEach((arrItem, index) => {callbackFn Scope Code Exec});
['apple', 'banana', 'carrot'].forEach((arrItem, index) => {
console.log(index + ' => ' + arrItem);
});
// 0 => apple
// 1 => banana
// 2 => carrot
arr.map((arrItem, index) => { return resultIteration });
const arr = [2, 4, 6, 8, 16];
const mapResult = arr.map(arrItem => arrItem * 2);
console.log(mapResult);
// [4, 8, 12, 16, 32]
arr.filter((arrItem, index) => { condition to return arrItem });
const arr = [2, 4, 6, 8, 16];
const filterResult =
arr.filter(arrItem => arrItem < 5 || arrItem > 10);
console.log(mapResult);
// [2, 4, 16]
const arr = ['banana', 'orange', 'apple', 'mango'];
arr.sort();
// ['apple', 'banana', 'mango', 'orange']
const arr = [22, 14, 0, 100, 89, 201];arr.sort();
// [0, 100, 14, 201, 22, 89]
// compareFn for ascending order
const compareFnAsc = (a, b) => a - b;
// compareFn for descending order
const compareFnDes = (a, b) => b - a;
const arr = [22, 14, 0, 100, 89, 201];
arr.sort(compareFnAsc);
// [0, 14, 22, 89, 100, 201]
arr.sort(compareFnDes);
// [201, 100, 89, 22, 14, 0]
arr.concat(value);arr.concat(value0, value1, ... , valueN);
// Example 1
// Concat 2 arrays of string and number type
const letters = ['a', 'b', 'c'];
const numbers = [1, 2, 3];
letters.concat(numbers);
// ['a', 'b', 'c', 1, 2, 3]
// Example 2
// Concat a string array with 2 values (number and number array)
const letters = ['a', 'b', 'c'];
const alphaNumeric = letters.concat(1, [2, 3]);
console.log(alphaNumeric);
// ['a', 'b', 'c', 1, 2, 3]
arr.every(callbackFn);
const callbackFn = (arrItem, index) => {
condition to check on every arrItem
}
const arr1 = [89, 0, -4, 34, -1, 10];
const arr2 = [89, 0, 45, 34, 1, 100];
arr1.every(arrItem => arrItem >= 0);
// false
arr2.every(arrItem => arrItem >= 0);
// true
const arr1 = [89, 0, 44, 34, -1, 10];
const arr2 = [-8, -45, -1, -100, -9];
arr1.some(arrItem => arrItem >= 0);
// true
arr2.some(arrItem => arrItem >= 0);
// false
const arr = [1, "2", 3, 4, 5];
// Type of matching argument should match with the array entry
// In the code below 2 is of type number but in arr "2" is stringconsole.log(arr.includes(2));
// false
console.log(arr.includes("2"));
// true
arr.join();arr.join(separator);
const arr = ['Hello', 'World', '!'];
arr.join();
// 'Hello,World,!'
arr.join(', ');
// 'Hello, World, !'
arr.join(' + ');
// 'Hello + World + !'
arr.join('');
// 'HelloWorld!'
arr.join(' ');
// 'Hello World !'
const callbackFn = (total, currArrItem, index, arr) => { ... }
arr.reduce(callbackFn, initVal)
// Combining above statements into one statement
arr.reduce((total, currArrItem, index, arr) => { ... }, initVal)
const arr = [1, 2, 3, 4];
const reducer = (prevVal, currVal) => prevVal + currVal;
arr.reduce(reducer);
// 10
// In the code below initVal is set to 5, which means that we have // to init the sum with initial value 5: 5 + 1 + 2 + 3 + 4 arr.reduce(reducer, 5);
// 15
arr.find((arrItem) => condition to check on arrItem);
const arr = [5, 10, 100, 12, 8, 130, 44];
const result = arr.find(arrItem => arrItem > 10);
console.log(result);
// 100
const arr = [5, 10, 100, 12, 8, 130, 44];
const result1 = arr.findIndex(arrItem => arrItem > 10);
const result2 = arr.findIndex(arrItem => arrItem > 1000);
console.log(result1);
// 2
console.log(result2);
// -1
const arr = [5, 10, 100, 12, 8, 130, 44];
arr.indexOf(12);
// 3
// As the input argument '12' is of type string and the input array // arr elements are of type number so there will be no match in
// input arr
arr.indexOf('12');
// -1
arr.fill(value)
// default startIndex = 0
arr.fill(value, startIndex)
// default endIndex is arr.length
arr.fill(value, startIndex, endIndex)
const arr1 = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H'];
arr1.fill(0);
// [0, 0, 0, 0, 0, 0, 0, 0]
const arr2 = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H'];
arr2.fill(0, 5);
// ['A', 'B', 'C', 'D', 'E', 0, 0, 0]
const arr3 = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H'];
arr3.fill(0, 5, 7);
// ['A', 'B', 'C', 'D', 'E', 0, 0, 'H']
const arr4 = [1, 2, 3, 4, 5];
arr4.fill();
//[undefined, undefined, undefined, undefined, undefined]
arr.slice()
arr.slice(startIndex)
arr.slice(startIndex, endIndex)
const arr = ['rats', 'sheep', 'cows', 'chickens', 'dogs', 1001];
arr.slice();
// ['rats', 'sheep', 'cows', 'chickens', 'dogs', 1001]
arr.slice(3);
// ['chickens', 'dogs', 1001]
arr.slice(2, 5);
// ['cows', 'chickens', 'dogs']
arr.slice(-4);
// ['cows', 'chickens', 'dogs', 1001]
arr.splice(startIndex)
arr.splice(startIndex, deleteCount)
arr.splice(startIndex, deleteCount, item1)
arr.splice(startIndex, deleteCount, item1, ..., itemN)
// Example 1
// Remove 0 items before index 2 & insert "drum"
let arr = ['angel', 'clown', 'mandarin', 'sturgeon'];
let removedArr = arr.splice(2, 0, 'drum');
// arr: ["angel", "clown", "drum", "mandarin", "sturgeon"]
// removedArr: []
// Example 2
// Remove 0 items before index 2 & insert "drum", "guitar"
let arr = ['angel', 'clown', 'mandarin', 'sturgeon'];
let removedArr = arr.splice(2, 0, 'drum', 'guitar');
// arr: ["angel", "clown", "drum", "guitar", "mandarin", "sturgeon"]
// removedArr: []
// Example 3
// Remove 1 element at index 3
let arr = ['angel', 'clown', 'drum', 'mandarin', 'sturgeon'];
let removedArr = myFish.splice(3, 1);
// arr: ["angel", "clown", "drum", "sturgeon"]
// removedArr: ["mandarin"]
// Example 4
// Remove 1 element at index 2 & insert "trumpet"
let arr = ['angel', 'clown', 'drum', 'sturgeon'];
let removedArr = arr.splice(2, 1, 'trumpet');
// arr: ["angel", "clown", "trumpet", "sturgeon"]
// removedArr: ["drum"]
// Example 5
// Remove 2 items from index 0 & insert "parrot", "anemone", "blue"
let arr = ['angel', 'clown', 'trumpet', 'sturgeon'];
let removedArr = arr.splice(0, 2, 'parrot', 'anemone', 'blue');
// arr: ["parrot", "anemone", "blue", "trumpet", "sturgeon"]
// removedArr: ["angel", "clown"]
const arr1 = [1, 2, 3];
arr1.reverse();
// [3, 2, 1]
const arr2 = ['1', '4', '3', 1, 'some string', 100];
arr2.reverse();
// [100, 'some string', 1, '3', '4', '1']
arr.push(value);arr.push(value0, value1, ... , valueN);
const animals = ['cats', 'rats', 'sheep'];
animals.push('cows');
// 4
console.log(animals);
// ['cats', 'rats', 'sheep', 'cows']
animals.push('chickens', 'dogs', 1001);
// 7
console.log(animals);
// ['cats', 'rats', 'sheep', 'cows', 'chickens', 'dogs', 1001]
const arr = ['rats', 'sheep', 'cows', 'chickens', 'dogs', 1001];
arr.pop();
// 1001
console.log(arr);
// ['rats', 'sheep', 'cows', 'chickens', 'dogs']
const arr = ['rats', 'sheep', 'cows', 'chickens', 'dogs', 1001];
arr.shift();
// 'rats'
console.log(arr);
// ['sheep', 'cows', 'chickens', 'dogs', 1001]
const animals = ['cats', 'rats', 'sheep'];
animals.unshift('cows');
// 4
console.log(animals);
// ['cows', 'cats', 'rats', 'sheep']
animals.unshift('chickens', 'dogs', 1001);
// 7
console.log(animals);
// ['chickens', 'dogs', 1001, 'cows', 'cats', 'rats', 'sheep']
Array.of(1);
// [1]
Array.of(1, 2, 3);
// [1, 2, 3]
Array.of(undefined);
// [undefined]
Array.from(value, callbackFn)
Array.from('hello');
// ['h', 'e', 'l', 'l', 'o']
Array.from([1, 2, 3], arrItem => arrItem * 4);
// [4, 8, 12]
arr.flat()
arr.flat(depth)
// Example 1
const arr1 = [0, 1, 2, [3, 4]];
console.log(arr1.flat());
// [0, 1, 2, 3, 4]
// Example 2
const arr2 = [0, 1, 2, [[[3, 4]]]];
console.log(arr2.flat(2));
// [0, 1, 2, [3, 4]]
console.log(arr2.flat(3));
// [0, 1, 2, 3, 4]
arr.at(index)
const arr = ['chickens', 'dogs', 1001, 'cows', 'cats', 'sheep']
arr.at(1);
// 'dogs'
arr.at(-1);
// 'sheep'
arr.at(2);
// 1001
arr.at(-2);
// 'cats'
arr.at(-100);
// undefined
学习更多技能
请点击下方公众号 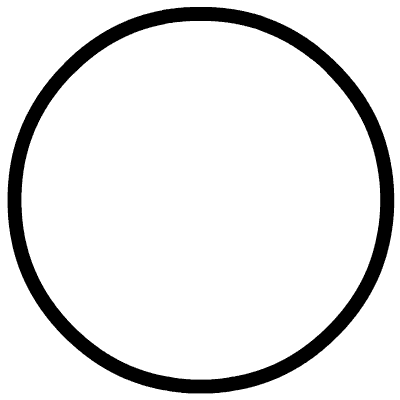
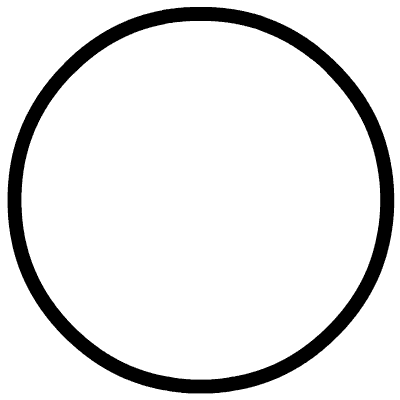

评论