poj 1039 Pipe
共 1999字,需浏览 4分钟
· 2021-11-30
Pipe
Description
The GX Light Pipeline Company started to prepare bent pipes for the new transgalactic light pipeline. During the design phase of the new pipe shape the company ran into the problem of determining how far the light can reach inside each component of the pipe. Note that the material which the pipe is made from is not transparent and not light reflecting.
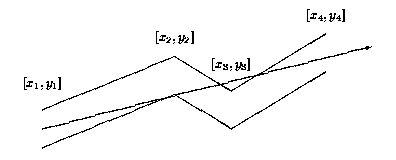
Each pipe component consists of many straight pipes connected tightly together. For the programming purposes, the company developed the description of each component as a sequence of points [x1; y1], [x2; y2], . . ., [xn; yn], where x1 < x2 < . . . xn . These are the upper points of the pipe contour. The bottom points of the pipe contour consist of points with y-coordinate decreased by 1. To each upper point [xi; yi] there is a corresponding bottom point [xi; (yi)-1] (see picture above). The company wants to find, for each pipe component, the point with maximal x-coordinate that the light will reach. The light is emitted by a segment source with endpoints [x1; (y1)-1] and [x1; y1] (endpoints are emitting light too). Assume that the light is not bent at the pipe bent points and the bent points do not stop the light beam.
Input
The input file contains several blocks each describing one pipe component. Each block starts with the number of bent points 2 <= n <= 20 on separate line. Each of the next n lines contains a pair of real values xi, yi separated by space. The last block is denoted with n = 0.
Output
The output file contains lines corresponding to blocks in input file. To each block in the input file there is one line in the output file. Each such line contains either a real value, written with precision of two decimal places, or the message Through all the pipe.. The real value is the desired maximal x-coordinate of the point where the light can reach from the source for corresponding pipe component. If this value equals to xn, then the message Through all the pipe. will appear in the output file.
Sample Input
4
0 1
2 2
4 1
6 4
6
0 1
2 -0.6
5 -4.45
7 -5.57
12 -10.8
17 -16.55
0
Sample Output
4.67
Through all the pipe.
管道
描述
GX 光管道公司开始为新的跨银河光管道准备弯管。在新管道形状的设计阶段,该公司遇到了确定光可以到达管道每个组件内部多远的问题。请注意,制成管道的材料不透明且不反射光。
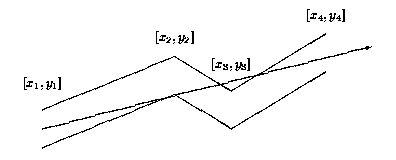
每个管道组件由许多紧密连接在一起的直管组成。出于编程目的,该公司将每个组件的描述开发为一系列点 [x1; y1], [x2; y2], . . ., [xn; yn],其中 x1 < x2 < 。. . xn . 这些是管道轮廓的上点。管道轮廓的底部点由 y 坐标减 1 的点组成。yi]有一个对应的底点[xi; (yi)-1](见上图)。该公司希望为每个管道组件找到光线将到达的最大 x 坐标点。光由具有端点 [x1; (y1)-1] 和 [x1; y1](端点也在发光)。假设光线在管道弯曲点处没有弯曲,并且弯曲点不停止光束。
输入
输入文件包含多个块,每个块描述一个管道组件。每个块以单独行上的弯曲点数 2 <= n <= 20 开始。接下来的 n 行中的每一行都包含一对由空格分隔的实数值 xi, yi。最后一个块用 n = 0 表示。
输出
输出文件包含与输入文件中的块相对应的行。对于输入文件中的每个块,输出文件中有一行。每个这样的行包含一个真实值,精确到两位小数,或者消息通过所有管道。真实值是光可以从相应管道的光源到达的点的所需最大 x 坐标成分。如果此值等于 xn,则消息通过所有管道。将出现在输出文件中。
样本输入
4
0 1
2 2
4 1
6 4
6
0 1
2 -0.6
5 -4.45
7 -5.57
12 -10.8
17 -16.55
0
样本输出
4.67
Through all the pipe.
代码:
#include
#include
#include
using namespace std;
const double precision=1e-3; //精度限制
const double inf=99999.0; //正无穷,注意下面使用的是负无穷
typedef class Node //折点坐标
{
public:
double x;
double y;
}point;
int max(int a,int b)
{
return a>b?a:b;
}
/*把浮点p的值转化为0,1或-1 (精度讨论)*/
int dblcmp(double p)
{
if(fabs(p) return 0; //只要是在0的邻域,就认为是0
return p>0?1:-1;
}
/*叉积运算*/
double det(double x1,double y1,double x2,double y2)
{
return x1*y2-x2*y1;
}
/*计算P点在AB的左侧还是右侧(AC与AB的螺旋关系)*/
double cross(point A,point B,point P)
{
return det(B.x-A.x , B.y-A.y , P.x-A.x , P.y-A.y);
}
/*判断直线AB、CD是否相交*/
bool check(point A,point B,point C,point D)
{
return (dblcmp(cross(A,B,C)) * dblcmp(cross(A,B,D)) <= 0);
//这里对黑书P353所述模板的相交约束条件做了修改
//目的是允许 入射光线L 与 折点处垂线 不规范相交(即垂线的端点可以落在L上 或者 允许延长线相交)
}
/*计算直线AB、CD的交点横坐标*/
//本题这里传参是有讲究的,AB是代表光线L与管道的交点,CD是代表上管壁或者下管壁的端点
//之所以这样做,是因为AB与CD实质上是不相交的,是AB的延长线与CD相交
//按照上述传参顺序,根据修改后的模板,那么仅仅判断C、D是否在AB的两侧,就能计算 AB延长线与CD的交点
//倘若传参顺序错了,就会判断A、B是否在CD的两侧,但是AB一定是在CD同侧的,也就不能求交点了
double intersection(point A,point B,point C,point D)
{
double area1=cross(A,B,C);
double area2=cross(A,B,D);
int c=dblcmp(area1);
int d=dblcmp(area2);
if(c*d<0) //CD在AB的两侧,规范相交
return (area2*C.x - area1*D.x)/(area2-area1); //黑书P357交点计算公式
if(c*d==0) //CD的其中一个端点在AB上,不规范相交
if(c==0)
return C.x;//C在AB上,返回AB与CD非规范相交时的交点C的横坐标
else
return D.x;//D在AB上,返回AB与CD非规范相交时的交点D的横坐标
return -inf; //CD在AB同侧,无交点,返回 负无穷
}
int main(int i,int j,int k)
{
int n; //折点数
while(cin>>n)
{
if(!n)
break;
point* up=new point[n+1]; //上折点
point* down=new point[n+1]; //下折点
double max_x=-inf; //最大可见度(管中最远可见点的横坐标)
/*Input*/
for(i=1;i<=n;i++)
{
cin>>up[i].x>>up[i].y;
down[i].x=up[i].x;
down[i].y=up[i].y-1;
}
bool flag=false; //标记当前光线L(直线up[i]->down[j])能否贯通全管
for(i=1;i<=n;i++) //枚举所有通过一个上折点、一个下折点的直线
{
for(j=1;j<=n;j++)
if(i!=j)
{
for(k=1;k<=n;k++) //直线L最大延伸到第k-1节管子
if(!check(up[i],down[j],up[k],down[k])) //up[k]->down[k]为折点处垂直x轴的直线
break;
if(k>n)
{
flag=true;
break;
}
else if(k>max(i,j)) //由于不清楚L究竟是与第k-1节管子的上管壁还是下管壁相交,因此都计算交点,取最优
{ //举例:若实际L是与上管壁相交,当计算下管壁时,得到的是第k-1个下折点,并不会是最优
//反之亦同理
double temp=intersection(up[i],down[j],up[k],up[k-1]);
if(max_x < temp) //L与第k-1节管子的上管壁相交
max_x=temp;
temp=intersection(up[i],down[j],down[k],down[k-1]);
if(max_x < temp) //L与第k-1节管子的上管壁相交
max_x=temp;
}
}
if(flag)
break;
}
if(flag)
cout<<"Through all the pipe."< else
cout<
/*Relax Room*/
delete up,down;
}
return 0;
}