将 Vue 渲染到嵌入式液晶屏
SegmentFault
共 8176字,需浏览 17分钟
·
2020-12-08 22:10
前言
之前看了雪碧大佬的将 React 渲染到嵌入式液晶屏觉得很有意思,React能被渲染到嵌入式液晶屏,那Vue是不是也可以呢?所以本文我们要做的就是:
如标题所示,就是将Vue渲染到嵌入式液晶屏。这里使用的液晶屏是0.96 寸大128x64分辨率的SSD1306。要将Vue渲染到液晶屏,我们还需要一个桥梁,它必须具备控制液晶屏及运行代码的能力。而树莓派的硬件对接能力和可编程性天然就具备这个条件。最后一个问题来了,我们用什么技术来实现呢?
这里我选择了 Node.js。原因:
Atwood 定律:“任何可以使用 JavaScript 来编写的应用,最终会由 JavaScript 编写。” ? 驱动硬件我大 Node.js 一行npm install走天下。?
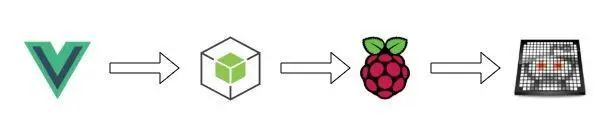
在 Node.js 运行 Vue 树莓派连接屏幕芯片 Node.js 驱动硬件
跨端渲染
React: ReactNative Taro ...
Vue: Weex UniApp ...
各种五花八门的前端框架纷纷袭来,前端工程师们纷纷抱怨学不动了~
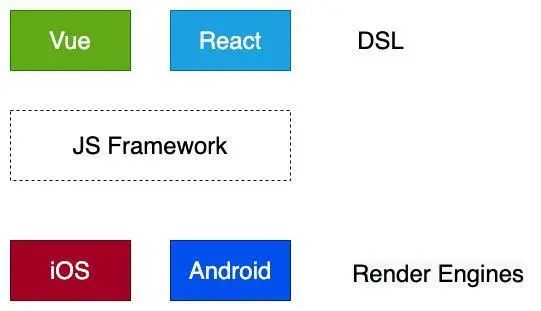
React Reconciler
const Reconciler = require("react-reconciler");
const HostConfig = {
// You'll need to implement some methods here.
// See below for more information and examples.
};
const MyRenderer = Reconciler(HostConfig);
const RendererPublicAPI = {
render(element, container, callback) {
// Call MyRenderer.updateContainer() to schedule changes on the roots.
// See ReactDOM, React Native, or React ART for practical examples.
},
};
module.exports = RendererPublicAPI;
Vue createRenderer
自定义渲染器可以传入特定于平台的类型,如下所示:
import { createRenderer } from 'vue'
const { render, createApp } = createRenderer({
patchProp,
...nodeOps
})
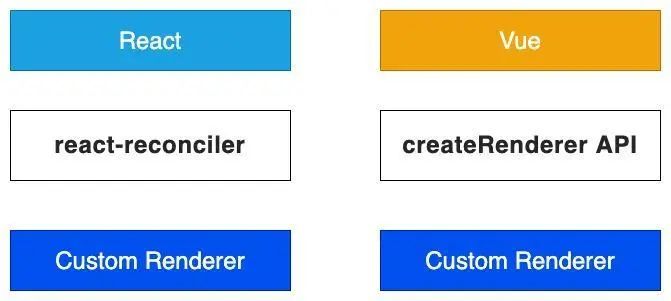
在 Node.js 上运行 Vue
SFC To JS
"0" y="0">Hello Vue
"0" y="20">{{ time }}
"0" y="40">Hi SSD3306
Create Custom Renderer
// index.js
// 自定义渲染器
import { createApp } from "./renderer.js";
// 组件
import App from "./App.vue";
// 容器
function getContainer() {
// ...
}
// 创建渲染器,将组件挂载到容器上
createApp(App).mount(getContainer());// renderer.js
import { createRenderer } from "vue";
// 定义渲染器,传入自定义nodeOps
const render = createRenderer({
// 创建元素
createElement(type) {},
// 插入元素
insert(el, parent) {},
// props更新
patchProp(el, key, preValue, nextValue) {},
// 设置元素文本
setElementText(node, text) {},
// 以下忽略,有兴趣的童鞋可自行了解
remove(el) {},
createText(type) {},
parentNode(node) {},
nextSibling(nide) {},
});
export function createApp(root) {
return render.createApp(root);
}
Adapter
创建元素实例 (create) 将元素实例插入容器,由容器进行管理 (insert) 状态改变时,通知容器进行更新 (update)
// adapter.js
// 文本元素
export class Text {
constructor(parent) {
// 提供一个父节点用于寻址调用更新 (前面提到状态更新由容器进行)
this.parent = parent;
}
// 元素绘制,这里需要实现文本元素渲染逻辑
draw(text) {
console.log(text);
}
}
// 适配器
export class Adapter {
constructor() {
// 装载容器
this.children = [];
}
// 装载子元素
append(child) {
this.children.push(child);
}
// 元素状态更新
update(node, text) {
// 找到目标渲染进行绘制
const target = this.children.find((child) => child === node);
target.draw(text);
}
clear() {}
}
// 容器 === 适配器实例
export function getContainer() {
return new Adapter();
}
Renderer Abstract
import { createRenderer } from "vue";
import { Text } from "./adapter";
let uninitialized = [];
const render = createRenderer({
// 创建元素,实例化Text
createElement(type) {
switch (type) {
case "text":
return new Text();
}
},
// 插入元素,调用适配器方法进行装载统一管理
insert(el, parent) {
if (el instanceof Text) {
el.parent = parent;
parent.append(el);
uninitialized.map(({ node, text }) => el.parent.update(node, text));
}
return el;
},
// props更新
patchProp(el, key, preValue, nextValue) {
el[key] = nextValue;
},
// 文本更新,重新绘制
setElementText(node, text) {
if (node.parent) {
console.log(text);
node.parent.clear(node);
node.parent.update(node, text);
} else {
uninitialized.push({ node, text });
}
},
remove(el) {},
createText(type) {},
parentNode(node) {},
nextSibling(nide) {},
});
export function createApp(root) {
return render.createApp(root);
}
树莓派连接屏幕芯片
SSD1306 OLED
硬件接线
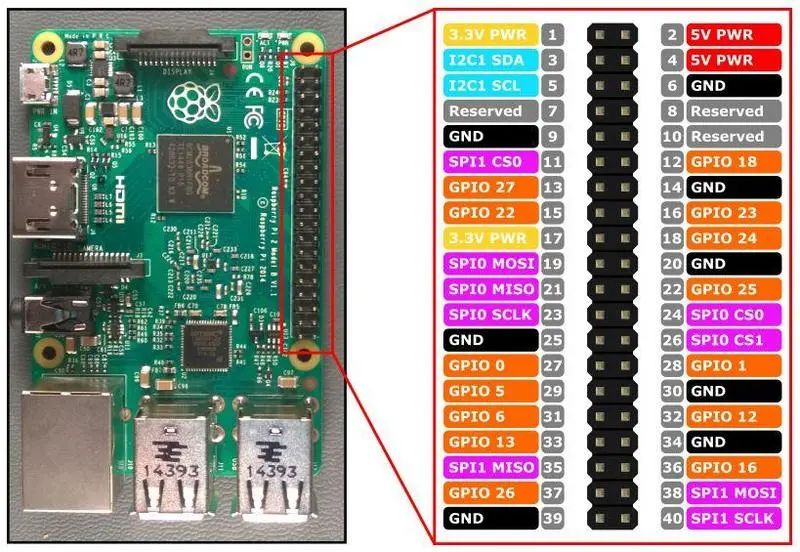
屏幕 VCC 接树莓派 1 号引脚。- 3.3v 电源 屏幕 GND 接树莓派 9 号引脚。- 地线 屏幕 SDA 接树莓派 3 号引脚。- IIC 通信中为数据管脚 屏幕 SCL 接树莓派 5 号引脚。- IIC 通信中为时钟管脚
树莓派启用 I2C
1.安装工具包
sudo apt-get install -y i2c-tools
2.启用 I2C
sudo raspi-config 选择 Interfacing Options Enable I2C
3.检查设备挂载状态
sudo i2cdetect -y 1
Node.js 驱动硬件
Node.js Lib
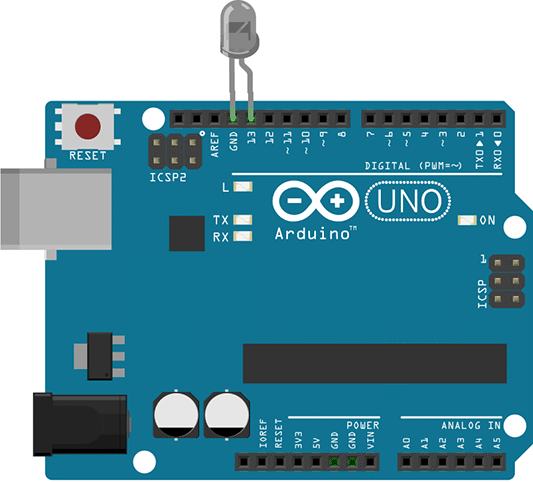
驱动程序实现
// oled.js
const five = require("johnny-five");
const Raspi = require("raspi-io").RaspiIO;
const font = require("oled-font-5x7");
const Oled = require("oled-js");
const OPTS = {
width: 128, // 分辨率 0.96寸 ssd1306 128*64
height: 64, // 分辨率
address: 0x3c, // 控制输入地址,ssd1306 默认为0x3c
};
class OledService {
constructor() {
this.oled = null;
}
/**
* 初始化: 创建一个Oled实例
* 创建后,我们就可以通过操作Oled实例来控制屏幕了
*/
init() {
const board = new five.Board({
io: new Raspi(),
});
// 监听程序退出,关闭屏幕
board.on("exit", () => {
this.oled && this.remove();
});
return new Promise((resolve, reject) => {
board.on("ready", () => {
// Raspberry Pi connect SSD 1306
this.oled = new Oled(board, five, OPTS);
// 打开屏幕显示
this.oled.turnOnDisplay();
resolve();
});
});
}
// 绘制文字
drawText({ text, x, y }) {
// 重置光标位置
this.oled.setCursor(+x, +y);
// 绘制文字
this.oled.writeString(font, 2, text, 1, true, 2);
}
clear({ x, y }) {
this.oled.setCursor(+x, +y);
}
// 刷新屏幕
update() {
this.oled.update();
}
remove() {
// 关闭显示
this.oled.turnOffDisplay();
this.oled = null;
}
}
export function oledService() {
return new OledService();
}
// index.js
import { createApp } from "./renderer.js";
import { getContainer } from "./adapter";
import { oledService } from "./oled";
import App from "./App.vue";
const oledIns = oledService();
oledIns.init().then(() => {
createApp(App).mount(getContainer(oledIns));
});
// adapter.js
export class Text {
constructor(parent) {
this.parent = parent;
}
draw(ints, opts) {
ints.drawText(opts);
ints.update();
}
}
export class Adapter {
constructor(oledIns) {
this.children = [];
this.oled = oledIns;
}
append(child) {
this.children.push(child);
}
update(node, text) {
const target = this.children.find((child) => child === node);
target.draw(this.oled, {
text,
x: node.x,
y: node.y,
});
}
clear(opts) {
this.oled.clear(opts);
}
}
export function getContainer(oledIns) {
return new Adapter(oledIns);
}
效果展示
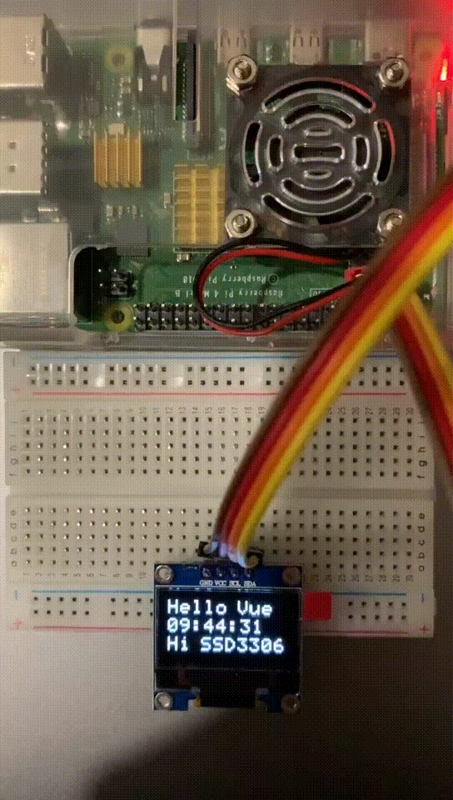
结语
评论