15个必须知道的JavaScript数组方法
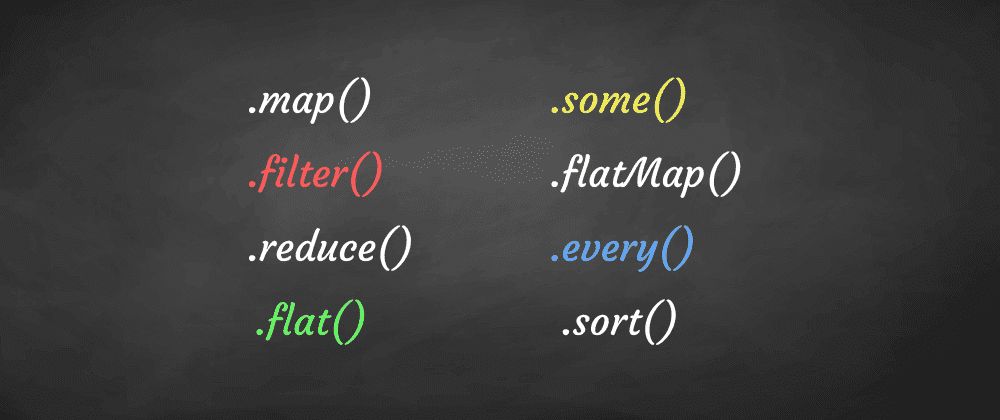
1.some() 2. reduce() 3. Every() 4. map() 5. flat() 6. filter() 7. forEach() 8. findIndex() 9. find() 10. sort() 11. concat() 12. fill() 13. includes() 14. reverse() 15. flatMap()
注意,大多数情况下,我们将简化作为参数传递的函数。
// Instead of using this waymyAwesomeArray.some(test => { if (test === "d") { return test }})// We'll use the shorter onemyAwesomeArray.some(test => test === "d")
1、some()
const myAwesomeArray = ["a", "b", "c", "d", "e"]
myAwesomeArray.some(test => test === "d")
//-------> Output : true
2、reduce()
const myAwesomeArray = [1, 2, 3, 4, 5]
myAwesomeArray.reduce((total, value) => total * value)
// 1 * 2 * 3 * 4 * 5
//-------> Output = 120
3、Every()
const myAwesomeArray = ["a", "b", "c", "d", "e"]
myAwesomeArray.every(test => test === "d")
// -------> Output : falseconst myAwesomeArray2 = ["a", "a", "a", "a", "a"]
myAwesomeArray2.every(test => test === "a")
//-------> Output : true
4、map()
const myAwesomeArray = [5, 4, 3, 2, 1]myAwesomeArray.map(x => x * x)
//-------> Output : 25
// 16
// 9
// 4
// 1
5、flat()
const myAwesomeArray = [[1, 2], [3, 4], 5]
myAwesomeArray.flat()
//-------> Output : [1, 2, 3, 4, 5]
6、filter()
const myAwesomeArray = [ { id: 1, name: "john" },
{ id: 2, name: "Ali" }, { id: 3, name: "Mass" },
{ id: 4, name: "Mass" },]
myAwesomeArray.filter(element => element.name === "Mass")
//-------> Output : 0:{id: 3, name: "Mass"},
// 1:{id: 4, name: "Mass"}
7、forEach()
const myAwesomeArray = [ { id: 1, name: "john" },
{ id: 2, name: "Ali" }, { id: 3, name: "Mass" },]
myAwesomeArray.forEach(element => console.log(element.name))
//-------> Output : john
// Ali
// Mass
8、 findIndex()
const myAwesomeArray = [ { id: 1, name: "john" },
{ id: 2, name: "Ali" }, { id: 3, name: "Mass" },]myAwesomeArray.findIndex(element => element.id === 3)// -------> Output : 2myAwesomeArray.findIndex(element => element.id === 7)//-------> Output : -1
9、 find()
const myAwesomeArray = [ { id: 1, name: "john" },
{ id: 2, name: "Ali" }, { id: 3, name: "Mass" },]
myAwesomeArray.find(element => element.id === 3)
// -------> Output : {id: 3, name: "Mass"}
myAwesomeArray.find(element => element.id === 7)
//-------> Output : undefined
10、 sort()
const myAwesomeArray = [5, 4, 3, 2, 1]
// Sort from smallest to largestmyAwesomeArray.sort((a, b) => a - b)
// -------> Output : [1, 2, 3, 4, 5]
// Sort from largest to smallestmyAwesomeArray.sort((a, b) => b - a)
//-------> Output : [5, 4, 3, 2, 1]
11、 concat()
const myAwesomeArray = [1, 2, 3, 4, 5]const
myAwesomeArray2 = [10, 20, 30, 40, 50]
myAwesomeArray.concat(myAwesomeArray2)
//-------> Output : [1, 2, 3, 4, 5, 10, 20, 30, 40, 50]
12、 fill()
const myAwesomeArray = [1, 2, 3, 4, 5]
// The first argument (0) is the value
// The second argument (1) is the starting index
// The third argument (3) is the ending indexmyAwesomeArray.fill(0, 1, 3)
//-------> Output : [1, 0, 0, 4, 5]
13、 includes()
const myAwesomeArray = [1, 2, 3, 4, 5]
myAwesomeArray.includes(3)
// -------> Output : truemyAwesomeArray.includes(8)
// -------> Output : false
14、 reverse()
const myAwesomeArray = ["e", "d", "c", "b", "a"]
myAwesomeArray.reverse()
// -------> Output : ['a', 'b', 'c', 'd', 'e']
15、 flatMap()
const myAwesomeArray = [[1], [2], [3], [4], [5]]
myAwesomeArray.flatMap(arr => arr * 10)
//-------> Output : [10, 20, 30, 40, 50]
// With .flat() and .map()myAwesomeArray.flat().map(arr => arr * 10)
//-------> Output : [10, 20, 30, 40, 50]
专注分享当下最实用的前端技术。关注前端达人,与达人一起学习进步!
长按关注"前端达人"
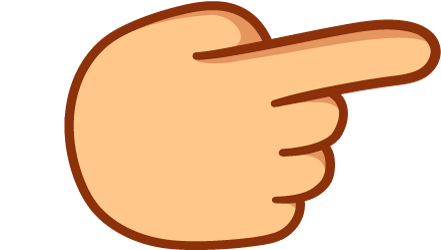
评论