如何在 ASP.Net Core 中使用 MediatR
共 6640字,需浏览 14分钟
· 2021-03-12
MediatR 是一个 中介者模式
的.NET开源实现, 中介者模式
管控了一组对象之间的相互通讯并有效的减少了对象之间错综复杂的相互依赖,在 中介者模式
中,一个对象不需要直接和另一个对象进行通讯,而是通过 中介者
进行转达,这篇文章将会讨论如何在 ASP.Net Core 中使用 MediatR 。
安装 MediatR
在 ASP.Net Core 中使用 MediatR 非常简单,你只需要通过 Nuget 安装如下两个包即可。
MediatR
MediatR.Extensions.Microsoft.DependencyInjection
当前最新的版本为 9.0.0,如下图所示:
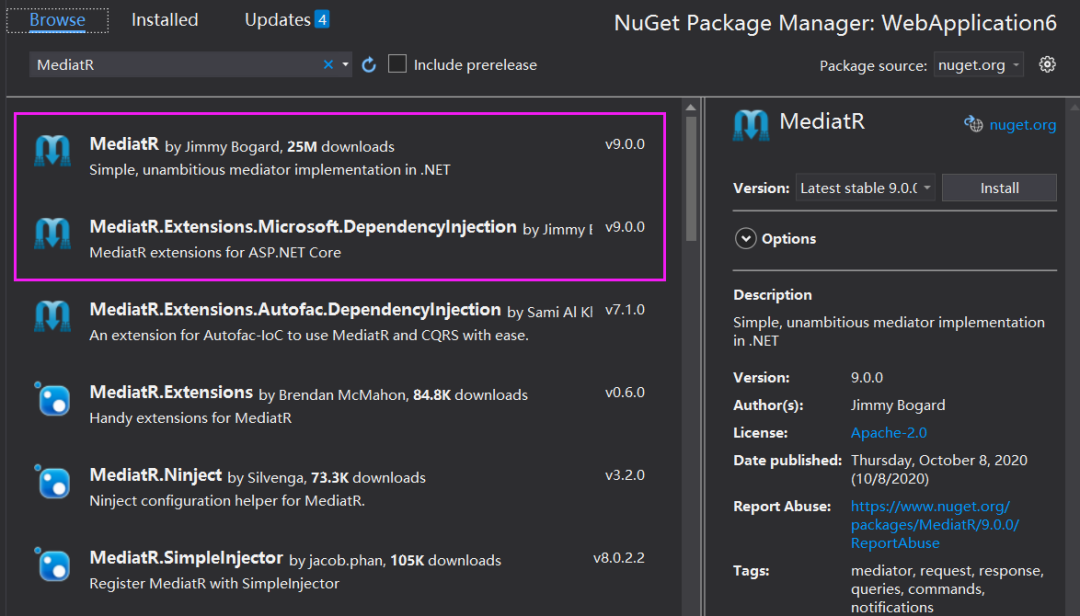
配置 MediatR
一旦上面的两个 Nuget 包安装到项目之后,接下来就可以在 Startup 类中进行 MediatR 的配置了,做法就是在 ConfigureServices()
方法中将 MediaR 注入到 IServiceCollection 容器中,如下代码所示:
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddMediatR(typeof(Startup));
services.AddControllers();
}
使用 MediaR 处理 通知事件
MediatR 支持两种消息模式。
Request / Response 模式
Notification 模式
这篇文章我们将会讨论 Notification,接下来创建一个实现 INotification 接口的类,如下代码所示:
public class LogEvent : INotification
{
public string message;
public LogEvent(string message)
{
this.message = message;
}
}
为了能够处理 LogEvent 事件,还需再创建一个实现 INotificationHandler 接口的类,如下代码所示:
public class FileNotificationHandler : INotificationHandler<LogEvent>
{
public Task Handle(LogEvent notification, CancellationToken cancellationToken)
{
string message = notification.message;
Log(message);
return Task.FromResult(0);
}
private void Log(string message)
{
//Write code here to log message(s) to a text file
Debug.WriteLine("Write code here to log message(s) to a text file");
}
}
public class DBNotificationHandler : INotificationHandler<LogEvent>
{
public Task Handle(LogEvent notification, CancellationToken cancellationToken)
{
string message = notification.message;
Log(message);
return Task.FromResult(0);
}
private void Log(string message)
{
//Write code here to log message(s) to the database
Debug.WriteLine("Write code here to log message(s) to the database");
}
}
依赖注入 IMediator
刚才我已经为了 LogEvent 创建了两个处理 handler 类,接下来就可以通过 依赖注入
的方式将其注入到 Controller 中,如下代码所示:
[ApiController]
[Route("[controller]")]
public class WeatherForecastController : ControllerBase
{
private readonly ILogger<WeatherForecastController> _logger;
private readonly IMediator _mediator;
public WeatherForecastController(IMediator mediator, ILogger<WeatherForecastController> logger)
{
this._mediator = mediator;
this._logger = logger;
}
}
最后我们可以在 Action 中通过 publish 发布消息,如下代码所示:
[HttpGet]
public IEnumerable<WeatherForecast> Get()
{
_mediator.Publish(new LogEvent("Hello World"));
}
值得注意的是,执行程序后将会调用上面的 publish 方法,继而触发 DBNotificationHandler 和 FileNotificationHandler 的 Handle 方法,如下图所示:
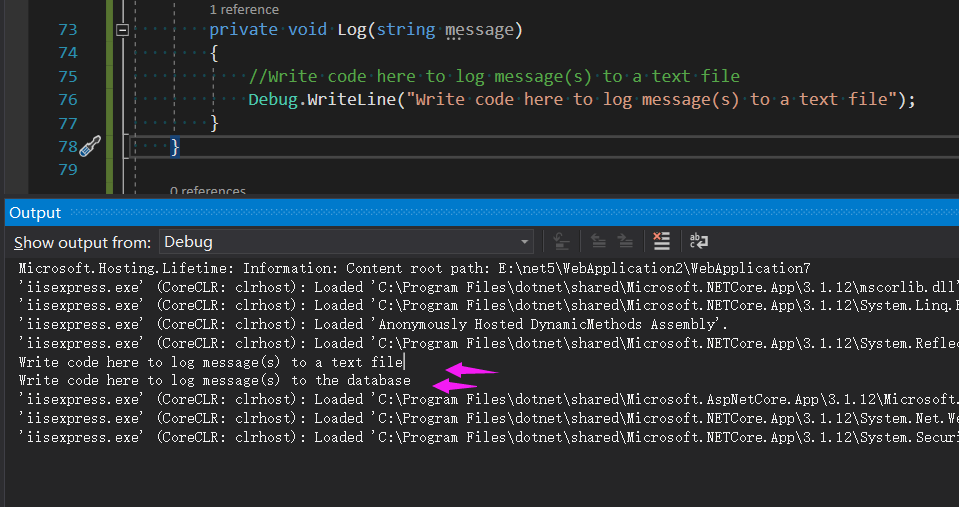
中介者模式
是一种行为式的设计模式,它可以有效地管控多个对象之间的交互方式并有效的减少交互双方的依赖关系,刚好 MediatR
就是这样一款成品的 中介者模式
的实现,关于 MediatR 的 request/response
模式,我会在后面的文章中和大家细说。
译文链接:https://www.infoworld.com/article/3393974/how-to-use-mediatr-in-aspnet-core.html
.NET Core实战项目之CMS 第一章 入门篇-开篇及总体规划
【.NET Core微服务实战-统一身份认证】开篇及目录索引
Redis基本使用及百亿数据量中的使用技巧分享(附视频地址及观看指南)
.NET Core中的一个接口多种实现的依赖注入与动态选择看这篇就够了
用abp vNext快速开发Quartz.NET定时任务管理界面