实现字符串split的6种方法
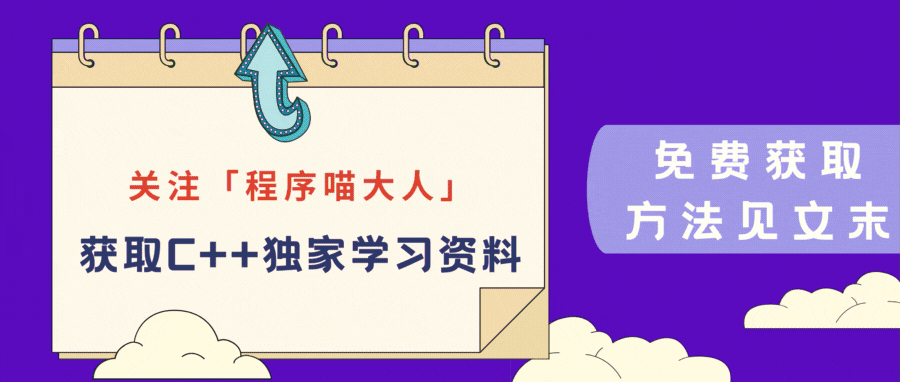
std::vector<std::string> stringSplit(const std::string& str, char delim) {
std::stringstream ss(str);
std::string item;
std::vector<std::string> elems;
while (std::getline(ss, item, delim)) {
if (!item.empty()) {
elems.push_back(item);
}
}
return elems;
}
std::vector<std::string> stringSplit(const std::string& str, char delim) {
std::size_t previous = 0;
std::size_t current = str.find(delim);
std::vector<std::string> elems;
while (current != std::string::npos) {
if (current > previous) {
elems.push_back(str.substr(previous, current - previous));
}
previous = current + 1;
current = str.find(delim, previous);
}
if (previous != str.size()) {
elems.push_back(str.substr(previous));
}
return elems;
}
std::vector<std::string> stringSplit(const std::string& str, char delim) {
std::size_t previous = 0;
std::size_t current = str.find_first_of(delim);
std::vector<std::string> elems;
while (current != std::string::npos) {
if (current > previous) {
elems.push_back(str.substr(previous, current - previous));
}
previous = current + 1;
current = str.find_first_of(delim, previous);
}
if (previous != str.size()) {
elems.push_back(str.substr(previous));
}
return elems;
}
std::vector<std::string> stringSplit(const std::string& strIn, char delim) {
char* str = const_cast<char*>(strIn.c_str());
std::string s;
s.append(1, delim);
std::vector<std::string> elems;
char* splitted = strtok(str, s.c_str());
while (splitted != NULL) {
elems.push_back(std::string(splitted));
splitted = strtok(NULL, s.c_str());
}
return elems;
}
std::vector<std::string> stringSplit(const std::string& str, char delim) {
std::vector<std::string> elems;
auto lastPos = str.find_first_not_of(delim, 0);
auto pos = str.find_first_of(delim, lastPos);
while (pos != std::string::npos || lastPos != std::string::npos) {
elems.push_back(str.substr(lastPos, pos - lastPos));
lastPos = str.find_first_not_of(delim, pos);
pos = str.find_first_of(delim, lastPos);
}
return elems;
}
std::vector<std::string> stringSplit(const std::string& str, char delim) {
std::string s;
s.append(1, delim);
std::regex reg(s);
std::vector<std::string> elems(std::sregex_token_iterator(str.begin(), str.end(), reg, -1),
std::sregex_token_iterator());
return elems;
}
https://www.zhihu.com/question/36642771
http://www.martinbroadhurst.com/how-to-split-a-string-in-c.html
评论