LeetCode刷题实战317:离建筑物最近的距离
You want to build a house on an empty land which reaches all buildings in the shortest amount of distance. You can only move up, down, left and right. You are given a 2D grid of values 0, 1 or 2, where:
Each 0 marks an empty land which you can pass by freely.
Each 1 marks a building which you cannot pass through.
Each 2 marks an obstacle which you cannot pass through.
示例
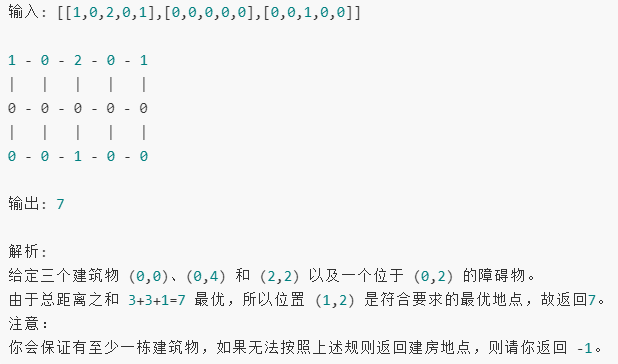
解题
class Solution {
public:
int shortestDistance(vector<vector<int>>& grid) {
int rows=grid.size();
int cols=grid[0].size();
//记录各个建筑到各个空格的距离
vector<vector<int>> dist(rows,vector<int>(cols,0));
//记录各个建筑能到各个空格的距离之和
vector<vector<int>> sum_dist(rows,vector<int>(cols,0));
//可以移动的方向
vector<vector<int>> step={{0,1},{0,-1},{1,0},{-1,0}};
//主要标识之前的各个建筑都能到达的空格,这样减少计算量
int times=0;
int res=INT_MAX;//从当前各个建筑都能到达的空地中,找出最小的距离之和值
//遍历各个位置
for(int i=0;i<rows;++i){
for(int j=0;j<cols;++j){
if(grid[i][j]==1){//若当前位置是建筑,从该位置开始进行广度优先搜索
res=INT_MAX;//初始化该值
pair<int,int> cur_pos;//辅助变量
queue<pair<int,int>> q;//广度优先搜索存储结构
q.push({i,j});//初始化
while(!q.empty()){
cur_pos=q.front();//当前点
q.pop();
for(int k=0;k<step.size();++k){//尝试的四个方向
//尝试的位置
int x=cur_pos.first+step[k][0];
int y=cur_pos.second+step[k][1];
//若当前位置没有越界,既有效,且之前的各个建筑都能够到达
if(x>=0&&x<rows&&y>=0&&y<cols&&grid[x][y]==times){
//则更新当前距离
dist[x][y]=dist[cur_pos.first][cur_pos.second]+1;
//更新对应的距离之和
sum_dist[x][y]+=dist[x][y];
//保存最小的距离和
res=min(res,sum_dist[x][y]);
//对应的值减一,标识当前建筑也访问过
--grid[x][y];
//压入当前位置
q.push({x,y});
}
}
}
//若该值没有变化,说明该建筑不能到达之前建筑都到达过的空地,则直接返回-1
if(res==INT_MAX){
return -1;
}
//标识当前建筑也访问过
--times;
}
}
}
return res;
}
};
评论