Python 3.14 将比 C++ 更快🤭
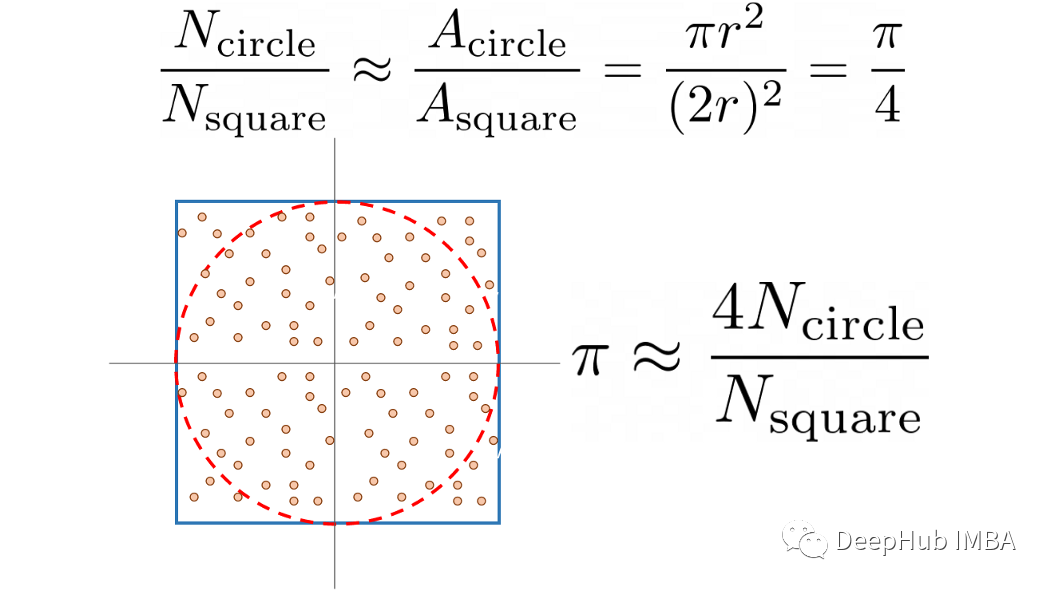
def estimate_pi( n_points: int, show_estimate: bool, ) -> None: """ Simple Monte Carlo Pi estimation calculation. Parameters ---------- n_points number of random numbers used to for estimation. show_estimate if True, will show the estimation of Pi, otherwise will not output anything. """ within_circle = 0 for _ in range(n_points): x, y = (random.uniform(-1, 1) for v in range(2)) radius_squared = x**2 + y**2 if radius_squared <= 1: within_circle += 1 pi_estimate = 4 * within_circle / n_points if not show_estimate: print("Final Estimation of Pi=", pi_estimate) def run_test( n_points: int, n_repeats: int, only_time: bool, ) -> None: """ Perform the tests and measure required time. Parameters ---------- n_points number of random numbers used to for estimation. n_repeats number of times the test is repeated. only_time if True will only print the time, otherwise will also show the Pi estimate and a neat formatted time. """ start_time = time.time() for _ in range(n_repeats): estimate_pi(n_points, only_time) if only_time: print(f"{(time.time() - start_time)/n_repeats:.4f}") else: print( f"Estimating pi took {(time.time() - start_time)/n_repeats:.4f} seconds per run." )
docker run -it --rm \
-v $PWD/your_script.py:/your_script.py \
python:3.11-rc-slim \
python /yourscript.py
def test_version(image: str) -> float:
"""
Run single_test on Python Docker image.
Parameter
---------
image
full name of the the docker hub Python image.
Returns
-------
run_time
runtime in seconds per test loop.
"""
output = subprocess.run([
'docker',
'run',
'-it',
'--rm',
'-v',
f'{cwd}/{SCRIPT}:/{SCRIPT}',
image,
'python',
f'/{SCRIPT}',
'--n_points',
str(N_POINTS),
'--n_repeats',
str(N_REPEATS),
'--only-time',
],
capture_output=True,
text=True,
)
avg_time = float(output.stdout.strip())
return avg_time
# Get test time for current Python version
base_time = test_version(NEW_IMAGE['image'])
print(f"The new {NEW_IMAGE['name']} took {base_time} seconds per run.\n")
# Compare to previous Python versions
for item in TEST_IMAGES:
ttime = test_version(item['image'])
print(
f"{item['name']} took {ttime} seconds per run."
f"({NEW_IMAGE['name']} is {(ttime / base_time) - 1:.1%} faster)"
)
The new Python 3.11 took 6.4605 seconds per run.
Python 3.5 took 11.3014 seconds.(Python 3.11 is 74.9% faster)
Python 3.6 took 11.4332 seconds.(Python 3.11 is 77.0% faster)
Python 3.7 took 10.7465 seconds.(Python 3.11 is 66.3% faster)
Python 3.8 took 10.6904 seconds.(Python 3.11 is 65.5% faster)
Python 3.9 took 10.9537 seconds.(Python 3.11 is 69.5% faster)
Python 3.10 took 8.8467 seconds.(Python 3.11 is 36.9% faster)
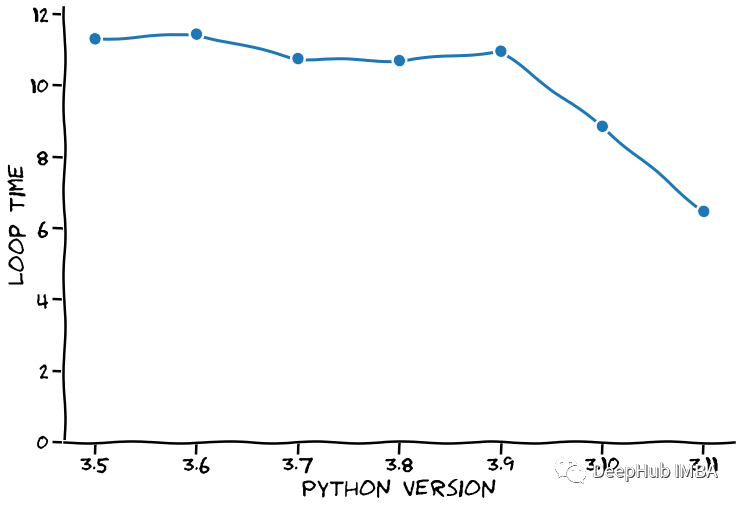
C 比 Python 快得多!
float estimate_pi(int n_points) {
double x, y, radius_squared, pi;
int within_circle=0;
for (int i=0; i < n_points; i++) {
x = (double)rand() / RAND_MAX;
y = (double)rand() / RAND_MAX;
radius_squared = x*x + y*y;
if (radius_squared <= 1) within_circle++;
}
pi=(double)within_circle/N_POINTS * 4;
return pi;
}
int main() {
double avg_time = 0;
srand(42);
for (int i=0; i < N_REPEATS; i++) {
auto begin = std::chrono::high_resolution_clock::now();
double pi = estimate_pi(N_POINTS);
auto end = std::chrono::high_resolution_clock::now();
auto elapsed = std::chrono::duration_cast<std::chrono::nanoseconds>(end - begin);
avg_time += elapsed.count() * 1e-9;
printf("Pi is approximately %g and took %.5f seconds to calculate.\n", pi, elapsed.count() * 1e-9);
}
printf("\nEach loop took on average %.5f seconds to calculate.\n", avg_time / N_REPEATS);
}
g++ -o pi_estimate pi_estimate.c
Pi is approximately 3.14227 and took 0.25728 seconds to calculate.
Pi is approximately 3.14164 and took 0.25558 seconds to calculate.
Pi is approximately 3.1423 and took 0.25740 seconds to calculate.
Pi is approximately 3.14108 and took 0.25737 seconds to calculate.
Pi is approximately 3.14261 and took 0.25664 seconds to calculate.
Each loop took on average 0.25685 seconds to calculate.
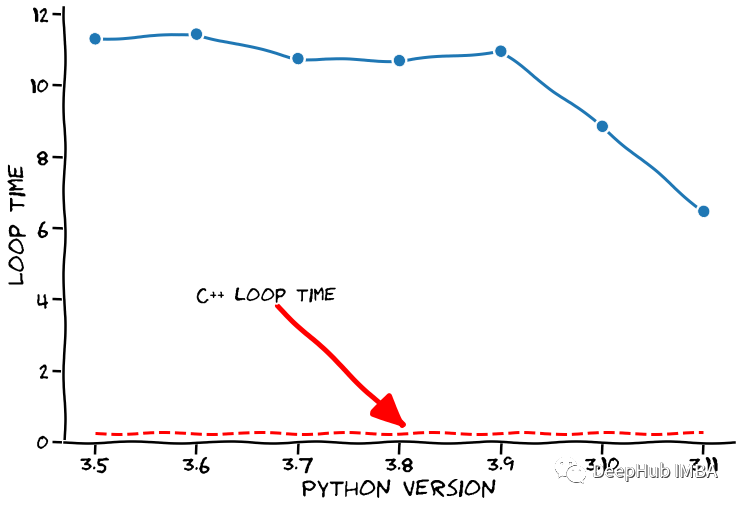
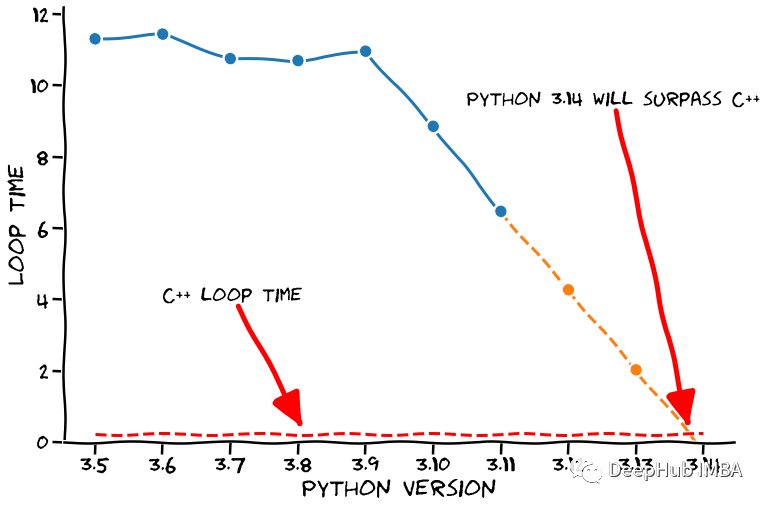
-End-
最近有一些小伙伴,让我帮忙找一些 面试题 资料,于是我翻遍了收藏的 5T 资料后,汇总整理出来,可以说是程序员面试必备!所有资料都整理到网盘了,欢迎下载!
面试题
】即可获取
评论