Flutter 旋转轮
共 13258字,需浏览 27分钟
·
2021-05-12 10:20
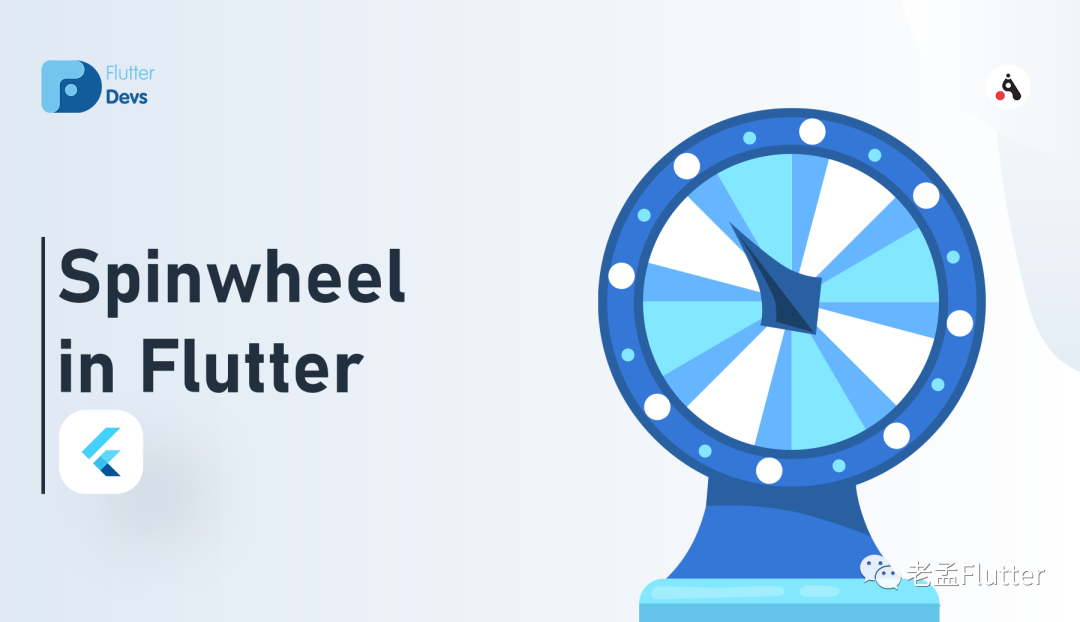
在在本文中,我们将探讨 “Flutter 中的旋转轮”。我们还将在flutter应用程序中使用「flutter_spinwheel」包来实现带有自定义选项的「Spinwheel」演示程序。
pub 地址:https://pub.dev/packages/flutter_spinwheel
效果演示:
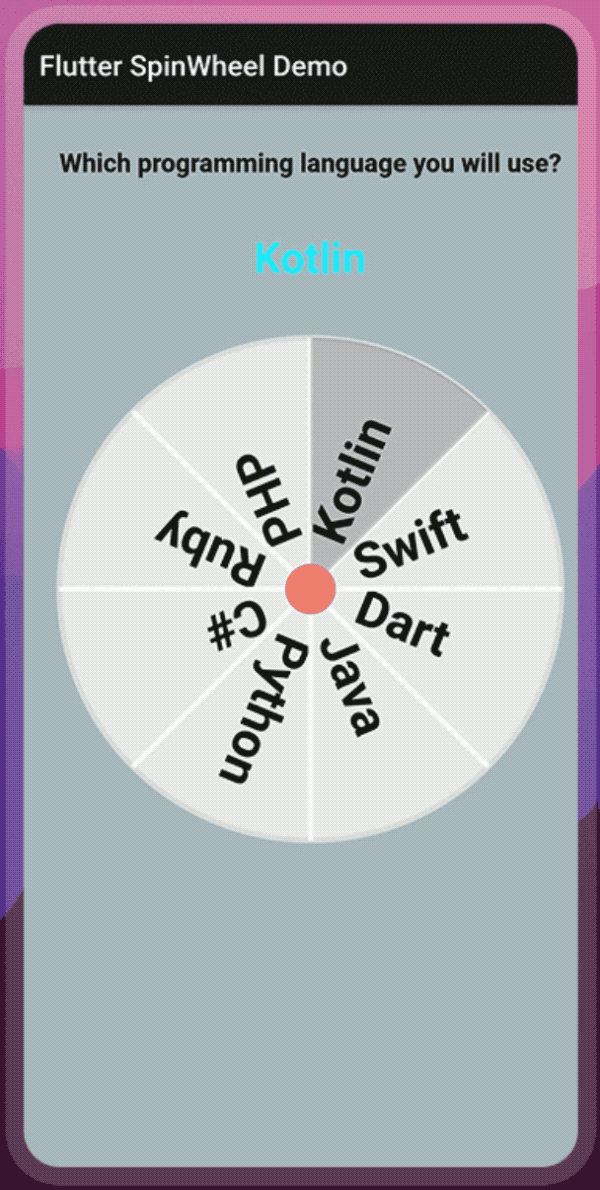
该演示视频展示了如何在Flutter中使用自旋轮。它显示了如何在flutter应用程序中使用「flutter_spinwheel」软件包运行「旋转轮」,并显示了当您点击该项目时,旋转器将移动。同样,您将沿顺时针/逆时针的任何方向移动微调器。它将在您的设备上显示所选的文本。
自旋轮有一些功能:
自动播放(启用/禁用) 长按以暂停(启用/禁用) 尺寸调整 文字/图片支持 图像调整支持 顺时针和逆时针平移进行导航 触摸即可在先前平移的方向上导航 绘画定制以改变外观 回调功能通知选定的项目
SDK中属性说明如下:
**touchToRotate:**此属性用于确定触摸微调器是否将使其沿以前的平移方向旋转(默认为顺时针方向)。 「itemCount:「此属性用于分配给」Spinwheel」类的菜单项数。应该在构造函数中处理它。 **shouldDrawBorder:**此属性用于确定是否应绘制边框。 **hideOthers:**此属性用于确定是否应绘制快门以隐藏除选定以外的所有选项。 **shutterPaint:**如果适用,此属性用于绘制用于绘制快门的设置。此外,它是可定制的。 **onChanged:**此 属性用于在每次更改选择时从微调器菜单返回所选值的回调。 「select」:此 属性用于选择(突出显示)圆的扇区。范围是0(项目大小)。想象它就像一个数组。选择编号从0开始。
使用
添加依赖
flutter_spinwheel: ^0.1.0
引入
import 'package:flutter_spinwheel/flutter_spinwheel.dart';
运行命令:「flutter packages get」
启用「AndriodX」
org.gradle.jvmargs=-Xmx1536M
android.enableR8=true
android.useAndroidX=true
android.enableJetifier=true
在libs目录下创建 「spinwheel_demo.dart」 文件,我们将创建一个由名称给定的字符串的两个列表,称为问题和答案。我们将创建由名称选择给出的动态列表的列表。同样,我们将创建一个由名称select给定的整数。
List<String> questions;
List<List<dynamic>> choices;
List<String> answers;
int select;
**initState()**方法 中初始化所有变量,例如问题,选择,选择和答案。
@override
void initState() {
super.initState();
questions = [
'Which programming language you will use?',
];
choices = [
['Kotlin', 'Swift', 'Dart', 'Java', 'Python', 'C#', 'Ruby', 'PHP'],
];
select = 0;
answers = ['', '', ''];
}
添加**ListView.builder()。**在此构建器中,我们将添加itemCount和itemBuilder。在itemBuilder中,我们将导航容器小部件。在小部件内,我们将添加一个边距,即容器的高度。他的子属性,我们将添加一个列小部件。在此小部件中,我们将添加两个文本,分别是问题和答案。
ListView.builder(
itemCount: 1,
itemBuilder: (context, index) => Container(
margin: EdgeInsets.all(30.0),
height: MediaQuery.of(context).size.height / 1.7,
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
Text(questions[index],style: TextStyle(fontSize: 18,
color: Colors.black, fontWeight: FontWeight.bold),),
Text(answers[index],style: TextStyle(fontSize: 30,
color: Colors.cyanAccent,fontWeight: FontWeight.bold),),
Center(
child: Spinwheel(
size: MediaQuery.of(context).size.height*0.6,
items: choices[index][0] is String
? choices[index].cast<String>()
: null,
select: select,
autoPlay: false,
hideOthers: false,
shouldDrawBorder: true,
onChanged: (val) {
if (this.mounted)
setState(() {
answers[index] = val;
});
},
),
)
],
),
),
)),
现在,我们将添加一个「Spinwheel()「包。在此程序包中,我们将添加」size」表示将在其上绘制圆形微调器的正方形,「item」表示将在微调器上显示该大小。每个人将获得一个相等分开的圈子部分;select表示圆的选择**(突出显示)「扇区的位置,「autoPlay」表示设置为true进行自动播放,「hideOthers」表示确定是否应绘制快门以隐藏除选定」项「以外的所有选项,应当绘制边框」指令**确定是否应绘制边框,「onChanged」表示每次更改选择时从微调器菜单返回所选值的回调。当我们运行应用程序时,我们应该获得屏幕的输出,如屏幕下方的截图所示。
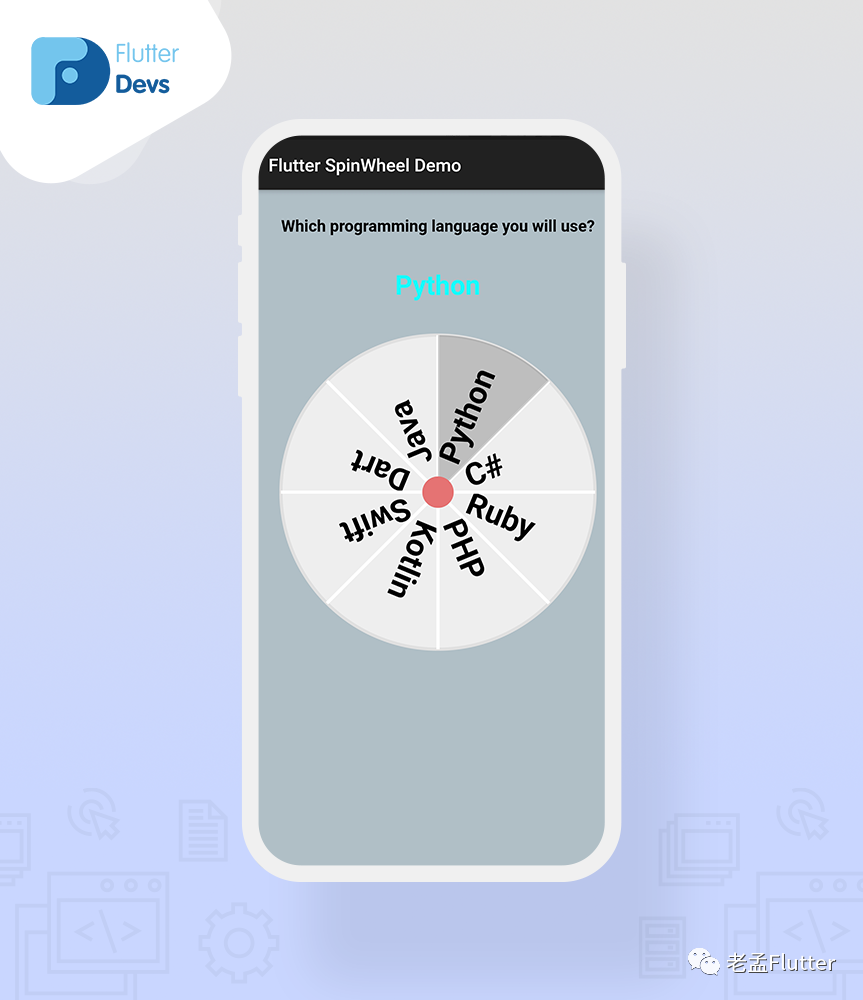
完整实现:
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter_spinwheel/flutter_spinwheel.dart';
class SpinWheelDemo extends StatefulWidget {
@override
_SpinWheelDemoState createState() => _SpinWheelDemoState();
}
class _SpinWheelDemoState extends State<SpinWheelDemo> {
List<String> questions;
List<List<dynamic>> choices;
List<String> answers;
int select;
@override
void initState() {
super.initState();
questions = [
'Which programming language you will use?',
];
choices = [
['Kotlin', 'Swift', 'Dart', 'Java', 'Python', 'C#', 'Ruby', 'PHP'],
];
select = 0;
answers = ['', '', ''];
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.blueGrey[200],
appBar: AppBar(
title: Text('Flutter SpinWheel Demo '),
automaticallyImplyLeading: false,
),
body: Scrollbar(
child: ListView.builder(
itemCount: 1,
itemBuilder: (context, index) => Container(
margin: EdgeInsets.all(30.0),
height: MediaQuery.of(context).size.height / 1.7,
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
Text(questions[index],style: TextStyle(fontSize: 18,
color: Colors.black, fontWeight: FontWeight.bold),),
Text(answers[index],style: TextStyle(fontSize: 30,
color: Colors.cyanAccent,fontWeight: FontWeight.bold),),
Center(
child: Spinwheel(
size: MediaQuery.of(context).size.height*0.6,
items: choices[index][0] is String
? choices[index].cast<String>()
: null,
select: select,
autoPlay: false,
hideOthers: false,
shouldDrawBorder: true,
onChanged: (val) {
if (this.mounted)
setState(() {
answers[index] = val;
});
},
),
)
],
),
),
)),
),
);
}
}