Redux通关简洁攻略 -- 看这一篇就够了!
共 26498字,需浏览 53分钟
·
2021-10-21 14:56
「Content」:本文章简要分析Redux & Redux生态的原理及其实现方式。
「Require」:理解本文需要一定redux的使用经验。
「Gain」:将收获
再写Redux,清楚地知道自己在做什么,每行代码会产生什么影响。 理解storeEnhancer middleware的工作原理,根据需求可以自己创造。 学习函数式范式是如何在实践中应用的大量优秀示例。 「Correction」:如有写错的地方,欢迎评论反馈
大厂技术 高级前端 Node进阶
点击上方 程序员成长指北,关注公众号
回复1,加入高级Node交流群
Redux设计哲学
Single source of truth
只能存在一个唯一的全局数据源,状态和视图是一一对应关系
Data - View Mapping
State is read-only
状态是只读的,当我们需要变更它的时候,用一个新的来替换,而不是在直接在原数据上做更改。
Changes are made with pure functions
状态更新通过一个纯函数(Reducer)完成,它接受一个描述状态如何变化的对象(Action)来生成全新的状态。
State Change
纯函数的特点是函数输出不依赖于任何外部变量,相同的输入一定会产生相同的输出,非常稳定。使用它来进行全局状态修改,使得全局状态可以被预测。当前的状态决定于两点:1. 初始状态 2. 状态存在期间传递的Action序列,只要记录这两个要素,就可以还原任何一个时间点的状态,实现所谓的“时间旅行”(Redux DevTools)
Single State + Pure Function
Redux架构
Redux组件
-
state: 全局的状态对象,唯一且不可变。 -
store: 调用createStore 函数生成的对象,里面封入了定义在createStore内部用于操作全局状态的方法,用户通过这些方法使用Redux。 -
action: 描述状态如何修改的对象,固定拥有一个type属性,通过store的dispatch方法提交。 -
reducer: 实际执行状态修改的纯函数,由用户定义并传入,接收来自dispatch的
action作为参数,计算返回全新的状态,完成state的更新,然后执行订阅的监听函数。
-
storeEnhancer: createStore的高阶函数封装,用于加强store的能力,redux提供的applyMiddleware是官方实现的一个storeEnhancer。 -
middleware: dispatch的高阶函数封装,由applyMiddleware把原dispatch替换为包含middleware链式调用的实现。
Redux构成
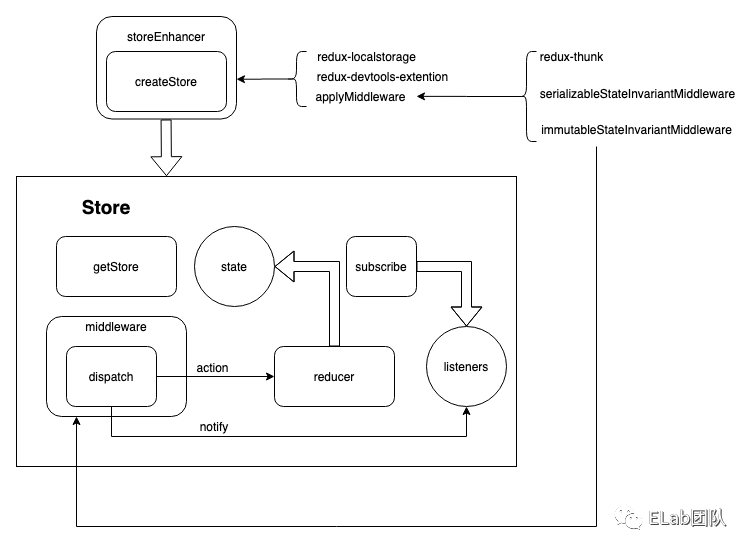
Redux API 实现
Redux Core
createStore
createStore 是一个大的闭包环境,里面定义了store本身,以及store的各种api。环境内部有对如获取state 、触发dispatch 、改动监听等副作用操作做检测的标志,因此reducer 被严格控制为纯函数。
redux设计的所有核心思想都在这里面实现,整个文件只有三百多行,简单但重要,下面简要列出了这个闭包中实现的功能及源码解析,以加强理解。
如果有storeEnhancer,则应用storeEnhancer
if (typeof enhancer !== 'undefined') {
// 类型检测
if (typeof enhancer !== 'function') {
...
}
// enhancer接受一个storeCreator返回一个storeCreator
// 在应用它的时候直接把它返回的storeCreatetor执行了然后返回对应的store
return enhancer(createStore)(reducer,preloadedState)
}
否则dispatch一个INIT的action,目的是让reducer产生一个初始的state。注意这里的INIT是Redux内部定义的随机数,reducer无法对它有明确定义的处理,而且此时的state可能为undefined,故为了能够顺利完成初始化,编写reducer时候我们需要遵循下面的两点规则:
-
处理未定义type的action,直接返回入参的state。 -
createStore如没有传入初始的state,则reducer中必须提供默认值。
// When a store is created, an "INIT" action is dispatched so that every
// reducer returns their initial state. This effectively populates
// the initial state tree.
dispatch({ type: ActionTypes.INIT } as A)
最后把闭包内定义的方法装入store对象并返回
const store = {
dispatch,
subscribe,
getState,
replaceReducer, // 不常用,故略过
[$$observable]: observable // 不常用,故略过
}
return store;
下面是这些方法的实现方式
getState
规定不能在reducer里调用getState,符合条件就返回当前状态,很清晰,不再赘述。
function getState(){
if (isDispatching) {
...
}
return currentState
}
dispatch
内置的dispatch 只提供了普通对象Action 的支持,其余像AsyncAction 的支持放到了middleware 中。dispatch做了两件事 :
-
调用reducer 产生新的state。 -
调用订阅的监听函数。
/*
* 通过原型链判断是否是普通对象
对于一个普通对象,它的原型是Object
*/
function isPlainObject(obj){
if (typeof obj !== 'object' || obj === null) return false
let proto = obj
// proto出循环后就是Object
while (Object.getPrototypeOf(proto) !== null) {
proto = Object.getPrototypeOf(proto)
}
return Object.getPrototypeOf(obj) === proto
}
function dispatch(action: A) {
// 判断一下是否是普通对象
if (!isPlainObject(action)) {
...
}
// redux要求action中需要有个type属性
if (typeof action.type === 'undefined') {
...
}
// reducer中不允许使用
if (isDispatching) {
...
}
// 调用reducer产生新的state 然后替换掉当前的state
try {
isDispatching = true
currentState = currentReducer(currentState, action)
} finally {
isDispatching = false
}
// 调用订阅的监听
const listeners = (currentListeners = nextListeners)
for (let i = 0; i < listeners.length; i++) {
const listener = listeners[i]
listener()
}
return action
}
subscribe
订阅状态更新,并返回取消订阅的方法。实际上只要发生dispatch调用,就算reducer 不对state做任何改动,监听函数也一样会被触发,所以为了减少渲染,各个UI bindings中会在自己注册的listener中做 state diff来优化性能。注意listener 是允许副作用存在的。
// 把nextListeners做成currentListeners的一个切片,之后对切片做修改,替换掉currentListeners
function ensureCanMutateNextListeners() {
if (nextListeners === currentListeners) {
nextListeners = currentListeners.slice()
}
}
function subscribe(listener: () => void) {
// 类型检测
if(typeof listener !== 'function'){
...
}
// reducer 中不允许订阅
if (isDispatching) {
...
}
let isSubscribed = true
ensureCanMutateNextListeners()
nextListeners.push(listener)
return function unsubscribe() {
// 防止重复取消订阅
if (!isSubscribed) {
return
}
// reducer中也不允许取消订阅
if (isDispatching) {
...
}
isSubscribed = false
ensureCanMutateNextListeners()
const index = nextListeners.indexOf(listener)
nextListeners.splice(index, 1)
currentListeners = null
}
}
applyMiddleware
applyMiddleware 是官方实现的一个storeEnhance,用于给redux提供插件能力,支持各种不同的Action。
storeEnhancer
从函数签名可以看出是createStore的高阶函数封装。
type StoreEnhancer = (next: StoreCreator) => StoreCreator;
CreateStore 入参中只接受一个storeEnhancer ,如果需要传入多个,则用compose把他们组合起来,关于高阶函数组合的执行方式下文中的Redux Utils - compose有说明,这对理解下面middleware 是如何链式调用的至关重要,故请先看那一部分。
middleware
type MiddlewareAPI = { dispatch: Dispatch, getState: () => State }
type Middleware = (api: MiddlewareAPI) => (next: Dispatch) => Dispatch
最外层函数的作用是接收middlewareApi ,给middleware提供store 的部分api,它返回的函数参与compose,以实现middleware的链式调用。
export default function applyMiddleware(...middlewares) {
return (createStore) =>{
// 初始化store,拿到dispatch
const store = createStore(reducer, preloadedState)
// 不允许在middlware中调用dispath
let dispatch: Dispatch = () => {
throw new Error(
'Dispatching while constructing your middleware is not allowed. ' + 'Other middleware would not be applied to this dispatch.'
)
}
const middlewareAPI: MiddlewareAPI = {
getState: store.getState,
dispatch: (action, ...args) => dispatch(action, ...args)
}
// 把api注入middlware
const chain = middlewares.map(middleware => middleware(middlewareAPI)) // 重点理解
// compose后传入dispatch,生成一个新的经过层层包装的dispath调用链
dispatch = compose<typeof dispatch>(...chain)(store.dispatch)
// 替换掉dispath,返回
return {
...store,
dispatch
}
}
}
再来看一个middleware加深理解:redux-thunk 使 redux 支持asyncAction ,它经常被用于一些异步的场景中。
// 最外层是一个中间件的工厂函数,生成middleware,并向asyncAction中注入额外参数
function createThunkMiddleware(extraArgument) {
return ({ dispatch, getState }) => (next) =>
(action) => {
// 在中间件里判断action类型,如果是函数那么直接执行,链式调用在这里中断
if (typeof action === 'function') {
return action(dispatch, getState, extraArgument); } // 否则继续
return next(action);
};
}
Redux Utils
compose
compose(组合)是函数式编程范式中经常用到的一种处理,它创建一个从右到左的数据流,右边函数执行的结果作为参数传入左边。
compose是一个高阶函数,接受n个函数参数,返回一个以上述数据流执行的函数。如果参数数组也是高阶函数,那么它compose后的函数最终执行过程就变成了如下图所示,高阶函数数组返回的函数将是一个从左到右链式调用的过程。
export default function compose(...funcs) {
if (funcs.length === 0) {
return (arg) => arg
}
if (funcs.length === 1) {
return funcs[0]
}
// 简单直接的compose
return funcs.reduce(
(a, b) =>
(...args: any) =>
a(b(...args))
)
}
combineReducers
它也是一种组合,但是是树状的组合。可以创建复杂的Reducer,如下图
实现的方法也较为简单,就是把map对象用函数包一层,返回一个mapedReducer,下面是一个简化的实现。
function combineReducers(reducers){
const reducerKeys = Object.keys(reducers)
const finalReducers = {}
for (let i = 0; i < reducerKeys.length; i++) {
const key = reducerKeys[i]
finalReducers[key] = reducers[key]
}
const finalReducerKeys = Object.keys(finalReducers)
// 组合后的reducer
return function combination(state, action){
let hasChanged = false
const nextState = {}
// 遍历然后执行
for (let i = 0; i < finalReducerKeys.length; i++) {
const key = finalReducerKeys[i]
const reducer = finalReducers[key]
const previousStateForKey = state[key]
const nextStateForKey = reducer(previousStateForKey, action)
if (typeof nextStateForKey === 'undefined') {
...
}
nextState[key] = nextStateForKey
hasChanged = hasChanged || nextStateForKey !== previousStateForKey
}
hasChanged = hasChanged || finalReducerKeys.length !== Object.keys(state).length
return hasChanged ? nextState : state
}
}
}
bindActionCreators
用actionCreator创建一个Action,立即dispatch它
function bindActionCreator(actionCreator,dispatch) {
return function (this, ...args) {
return dispatch(actionCreator.apply(this, args))
}
}
Redux UI bindings
React-redux
React-redux 是Redux官方实现的React UI bindings。它提供了两种使用Redux的方式:HOC和Hooks,分别对应Class组件和函数式组件。我们选择它的Hooks实现来分析,重点关注UI组件是如何获取到全局状态的,以及当全局状态变更时如何通知UI更新。
UI如何获取到全局状态
-
通过React Context存储全局状态
export const ReactReduxContext = /*#__PURE__*/
React.createContext<ReactReduxContextValue | null>(null)
-
把它封装成Provider组件
function Provider({ store, context, children }: ProviderProps) {
const Context = context || ReactReduxContext
return <Context.Provider value={contextValue}>{children}</Context.Provider>
}
-
提供获取store的 hook: useStore
function useStore(){
const { store } = useReduxContext()!
return store
}
State变更时如何通知UI更新
react-redux提供了一个hook:useSelector,这个hook向redux subscribe了一个listener,当状态变化时被触发。它主要做下面几件事情。
When an action is dispatched, useSelector() will do a reference comparison of the previous selector result value and the current result value. If they are different, the component will be forced to re-render. If they are the same, the component will not re-render.
-
subscribe
const subscription = useMemo(
() => createSubscription(store),
[store, contextSub]
)
subscription.onStateChange = checkForUpdates
-
state diff
function checkForUpdates() {
try {
const newStoreState = store.getState()
const newSelectedState = latestSelector.current!(newStoreState)
if (equalityFn(newSelectedState, latestSelectedState.current)) {
return
}
latestSelectedState.current = newSelectedState
latestStoreState.current = newStoreState
} catch (err) {
// we ignore all errors here, since when the component
// is re-rendered, the selectors are called again, and
// will throw again, if neither props nor store state
// changed
latestSubscriptionCallbackError.current = err as Error
}
forceRender()
}
-
re-render
const [, forceRender] = useReducer((s) => s + 1, 0)
forceRender()
脱离UI bindings,如何使用redux
其实只要完成上面三个步骤就能使用,下面是一个示例:
const App = ()=>{
const state = store.getState();
const [, forceRender] = useReducer(c=>c+1, 0);
// 订阅更新,状态变更刷新组件
useEffect(()=>{
// 组件销毁时取消订阅
return store.subscribe(()=>{
forceRender();
});
},[]);
const onIncrement = ()=> {
store.dispatch({type: 'increment'});
};
const onDecrement = ()=> {
store.dispatch({type: 'decrement'});
}
return (
<div style={{textAlign:'center', marginTop:'35%'}}>
<h1 style={{color: 'green', fontSize: '500%'}}>{state.count}</h1>
<button onClick={onDecrement} style={{marginRight: '10%'}}>decrement</button>
<button onClick={onIncrement}>increment</button>
</div>
)
}
小结
Redux核心部分单纯实现了它“单一状态”、“状态不可变”、“纯函数”的设定,非常小巧。对外暴露出storeEnhancer 与 middleware以在此概念上添加功能,丰富生态。redux的发展也证明这样的设计思路使redux拓展性非常强。
其中关于高阶函数的应用是我觉得非常值得借鉴的一个插件体系构建方式,不是直接设定生命周期,而是直接给予核心函数一次高阶封装,然后内部依赖compose完成链式调用,这可以降低外部开发者的开发心智。
Redux想要解决的问题是复杂状态与视图的映射难题,但Redux本身却没有直接实现,它只做了状态管理,然后把状态更新的监听能力暴露出去,剩下的状态缓存、状态对比、更新视图就抛给各大框架的UI-bindings,这既在保持了自身代码的单一性、稳定性、又能给真正在视图层使用redux状态管理的开发者提供“类响应式”的State-View开发体验。
我组建了一个氛围特别好的 Node.js 社群,里面有很多 Node.js小伙伴,如果你对Node.js学习感兴趣的话(后续有计划也可以),我们可以一起进行Node.js相关的交流、学习、共建。下方加 考拉 好友回复「Node」即可。
“分享、点赞、在看” 支持一波👍