手写一个HTTP图片资源服务器,太容易了叭!
阅读本文大概需要 8 分钟。
来自:binhao.blog.csdn.net/article/details/112059406
摘要
一、什么是http服务器
http服务器用来干什么:
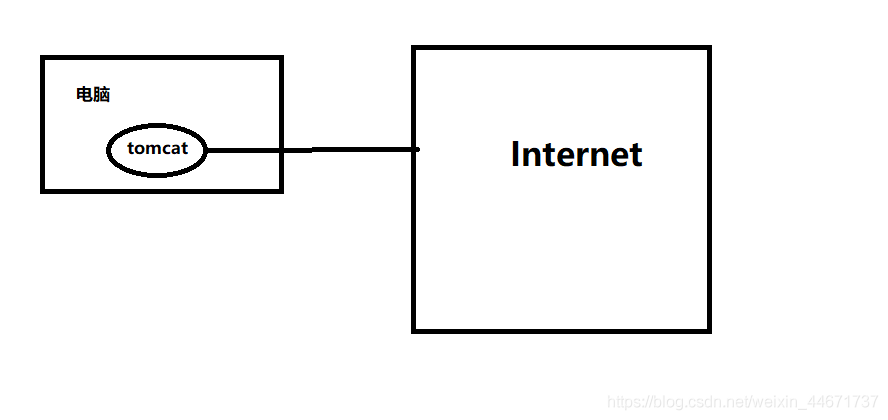
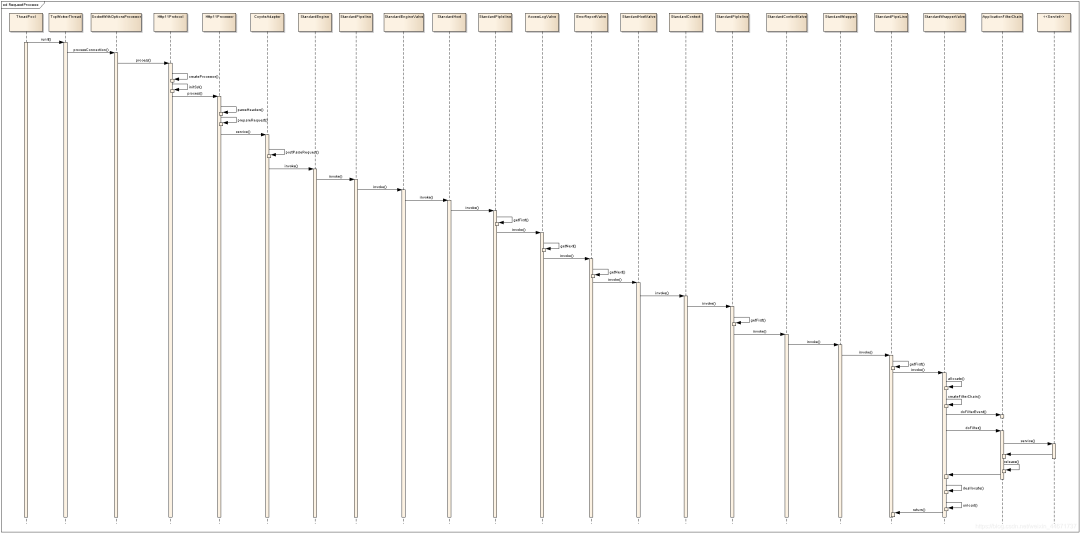
二、如何自己简单实现一个http服务器
什么样的请求能进来或者说是有效的?(第一层请求拦截) 请求的解析和响应结果的生成?(需要精通http协议) io模型如何选择,怎样充分利用发挥服务器性能?(涉及多线程,复杂的io模型) 可插拔要怎么实现?如tomcat可以通过一个servlet让开发人员进行处理。 …等等,实现一个http服务器绝对是一件很困难的事,所有网络应用层都是基于这个向上设计的。
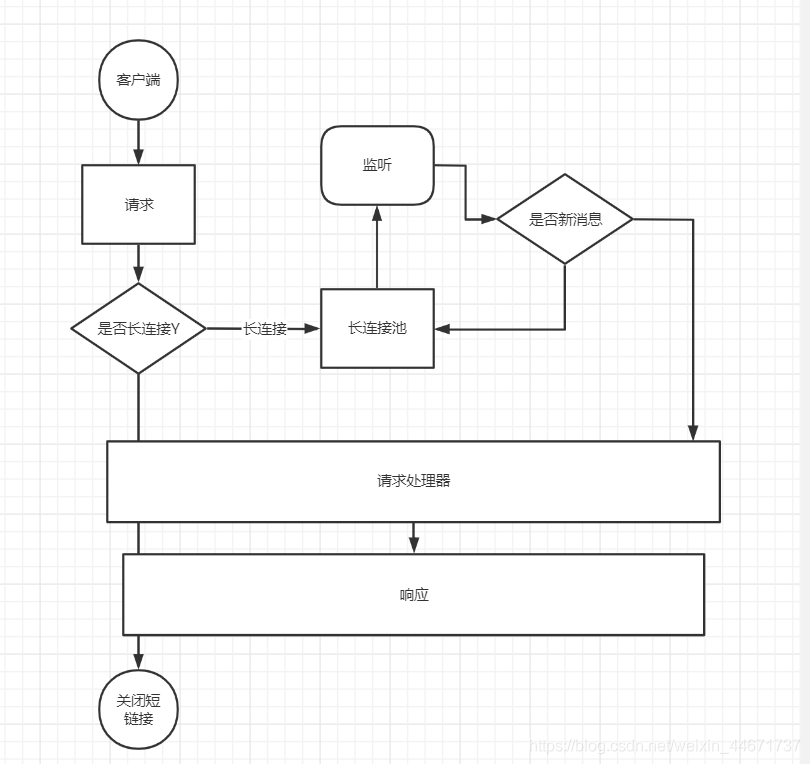
三、自己实现的http服务器
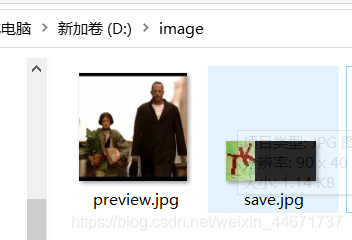
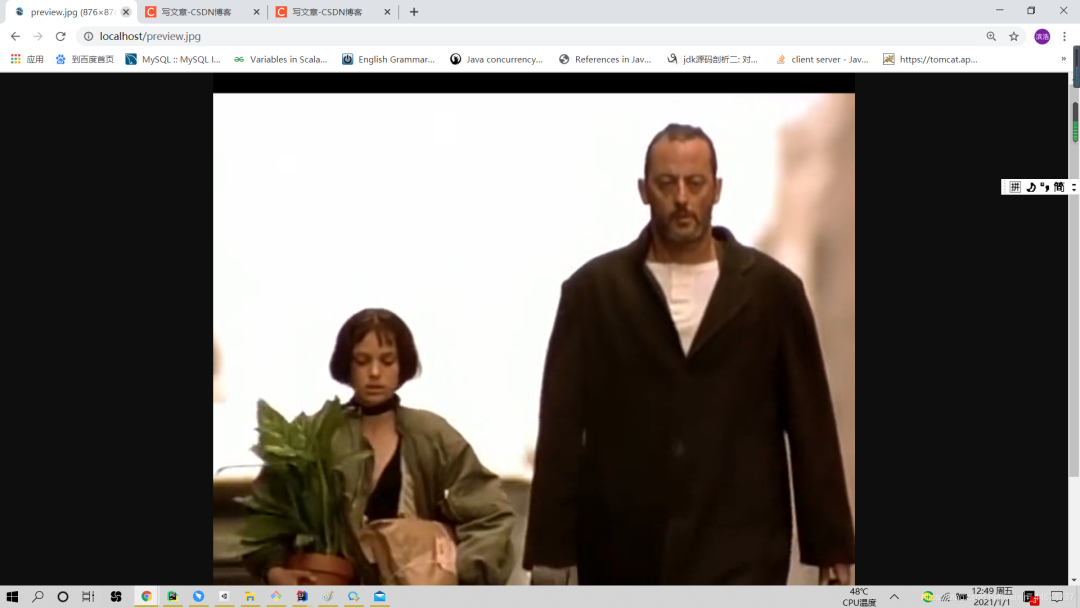
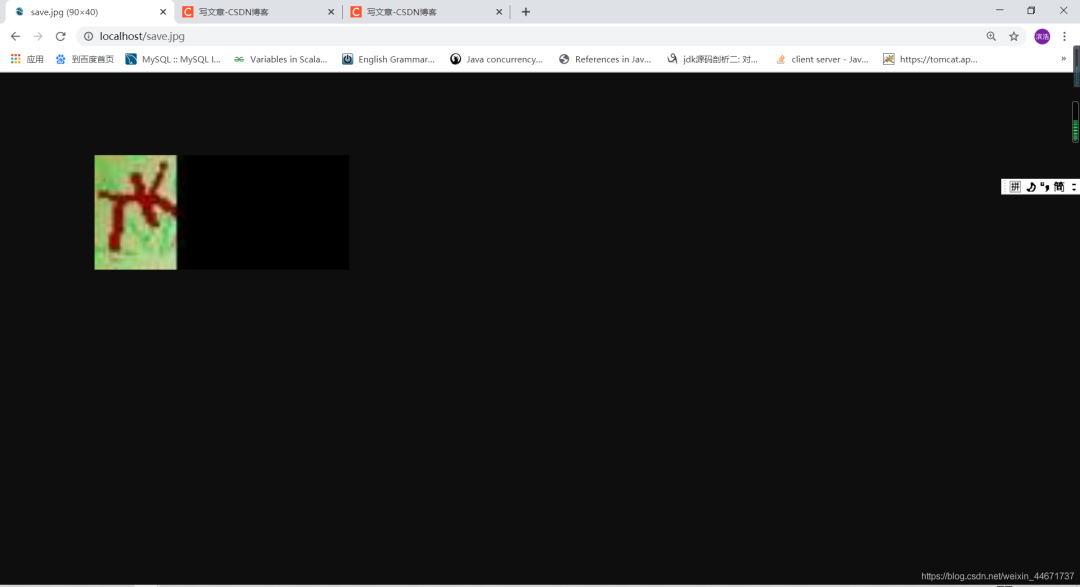
四、Http服务器实现(Java)
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.util.Properties;
/**
* Copyright(c)lbhbinhao@163.com
* @author liubinhao
* @date 2021/1/1
* ++++ ______ ______ ______
* +++/ /| / /| / /|
* +/_____/ | /_____/ | /_____/ |
* | | | | | | | | |
* | | | | | |________| | |
* | | | | | / | | |
* | | | | |/___________| | |
* | | |___________________ | |____________| | |
* | | / / | | | | | | |
* | |/ _________________/ / | | / | | /
* |_________________________|/b |_____|/ |_____|/
*/
public class HttpServer {
public static final String RESOURCE_PATH = "D:/image";
public static void main(String[] args) throws IOException {
ServerSocket server = new ServerSocket(80);
while (true){
Socket client = server.accept();
InputStream inputStream = client.getInputStream();
String s = handleNewClientMessage(inputStream);
Request request = Request.parse(s);
System.out.println(request);
//假设请求的都是文件
String uri = request.getUri();
File file = new File(RESOURCE_PATH + uri);
FileInputStream fileInputStream = new FileInputStream(file);
FileChannel channel = fileInputStream.getChannel();
ByteBuffer buffer = ByteBuffer.allocate((int) file.length());
channel.read(buffer);
buffer.rewind();
Response response = new Response();
response.setTopRow("HTTP/1.1 200 OK");
response.getHeaders().put("Content-Type",readProperties(getType(request.getUri())));
response.getHeaders().put("Content-Length",String.valueOf(file.length()));
response.getHeaders().put("Server","httpserver-lbh");
response.setBody(buffer.array());
response.setOutputStream(client.getOutputStream());
response.setClient(client);
System.out.println(response);
System.out.println(client.isClosed());
//响应客户端
response.service();
inputStream.close();
client.close();
}
}
private static String handleNewClientMessage(InputStream inputStream) throws IOException {
byte[] bytes = new byte[1024];
int read = inputStream.read(bytes);
StringBuilder buffer = new StringBuilder();
if (read != -1) {
for (byte b:bytes) {
buffer.append((char)b);
}
}
return buffer.toString();
}
/**
* 我个人放mimetype文件类型的文件,需要单独修改
*/
private static final String MIME_PROPERTIES_LOCATION =
"D:\\individual\\springcloudDemo\\src\\main\\java\\contentype.properties";
public static String readProperties(String key) {
System.out.println(System.getProperty("user.dir"));
Properties prop = new Properties();
try {prop.load(new FileInputStream(MIME_PROPERTIES_LOCATION));
return prop.get(key).toString();
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
public static String getType(String uri) {
System.out.println("uri:" + uri);
String stringDot = ".";
String dot = "\\.";
String[] fileName;
if (uri.contains(stringDot)) {
fileName = uri.split(dot);
return fileName[fileName.length - 1];
}
return null;
}
}
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
/**
* Copyright(c)lbhbinhao@163.com
* @author liubinhao
* @date 2021/1/1
* ++++ ______ ______ ______
* +++/ /| / /| / /|
* +/_____/ | /_____/ | /_____/ |
* | | | | | | | | |
* | | | | | |________| | |
* | | | | | / | | |
* | | | | |/___________| | |
* | | |___________________ | |____________| | |
* | | / / | | | | | | |
* | |/ _________________/ / | | / | | /
* |_________________________|/b |_____|/ |_____|/
*/
public class Request {
private String method;
private String uri;
private String version;
private byte[] body;
private Map<String, String> headers;
public String getMethod() {
return method;
}
public String getUri() {
return uri;
}
public String getVersion() {
return version;
}
public byte[] getBody() {
return body;
}
public Map<String, String> getHeaders() {
return headers;
}
public void setMethod(String method) {
this.method = method;
}
public void setUri(String uri) {
this.uri = uri;
}
public void setVersion(String version) {
this.version = version;
}
public void setHeaders(Map<String, String> headers) {
this.headers = headers;
}
public void setBody(byte[] body) {
this.body = body;
}
@Override
public String toString() {
return "Request{" +
"method='" + method + '\'' +
", uri='" + uri + '\'' +
", version='" + version + '\'' +
", body=" + Arrays.toString(body) +
", headers=" + headers +
'}';
}
private static final String CONTENT_SEPARATE_SIGN = "\r\n\r\n";
private static final String LINE_SEPARATE_SIGN = "\r\n";
private static final String SPACE_SEPARATE_SIGN = "\\s+";
private static final String KV_SEPARATE_SIGN = ":";
//**********************************解析请求*************************************
private static void parseTopReq(Request req, String[] topReq){
req.setMethod (topReq[0]);
req.setUri (topReq[1]);
req.setVersion(topReq[2]);
}
private static void parseHeaders(Request req, String[] headerStr){
HashMap<String, String> headers = new HashMap<>(12);
for (String s : headerStr) {
String[] keyValue = s.split(KV_SEPARATE_SIGN);
headers.put(keyValue[0], keyValue[1]);
}
req.setHeaders(headers);
}
public static Request parse(String reqStr) {
Request req = new Request();
String[] httpInfo = reqStr.split(CONTENT_SEPARATE_SIGN);
if (httpInfo.length==0){
return null;
}
if (httpInfo.length > 1){
req.setBody(httpInfo[1].getBytes());
}
String[] content = httpInfo[0].split(LINE_SEPARATE_SIGN);
// http first row of a http request
String[] firstRow = content[0].split(SPACE_SEPARATE_SIGN);
// http / get demo_path.type
parseTopReq(req, firstRow);
parseHeaders(req, Arrays.copyOfRange(content,1,content.length));
return req;
}
}
import java.io.IOException;
import java.io.OutputStream;
import java.net.Socket;
import java.util.HashMap;
import java.util.Map;
/**
* Copyright..@lbhbinhao@163.com
* Author:liubinhao
* Date:2020/12/28
* ++++ ______ ______ ______
* +++/ /| / /| / /|
* +/_____/ | /_____/ | /_____/ |
* | | | | | | | | |
* | | | | | |________| | |
* | | | | | / | | |
* | | | | |/___________| | |
* | | |___________________ | |____________| | |
* | | / / | | | | | | |
* | |/ _________________/ / | | / | | /
* |_________________________|/b |_____|/ |_____|/
*/
public class Response{
private String topRow;
private Map<String, String> headers = new HashMap<>(12);
private byte[] body;
private int responseCode;
private OutputStream outputStream;
public String getTopRow() {
return topRow;
}
public void setTopRow(String topRow) {
this.topRow = topRow;
}
public Map<String, String> getHeaders() {
return headers;
}
public void setHeaders(Map<String, String> headers) {
this.headers = headers;
}
public byte[] getBody() {
return body;
}
public void setBody(byte[] body) {
this.body = body;
}
public OutputStream getOutputStream() {
return outputStream;
}
public void setOutputStream(OutputStream outputStream) {
this.outputStream = outputStream;
}
public int getResponseCode() {
return responseCode;
}
public void setResponseCode(int responseCode) {
this.responseCode = responseCode;
}
public static final byte[] LINE_SEPARATE_SIGN_BYTES = "\r\n".getBytes();
public static final byte[] KV_SEPARATE_SIGN_BYTES = ":".getBytes();
private Socket client;
public Socket getClient() {
return client;
}
public void setClient(Socket client) {
this.client = client;
}
// 如果头数据里不包含Content-Length: x 类型为Transfer-Encoding:
// chunked 说明响应数据的长度不固定,结束符已"\r\n0\r\n"这5个字节为结束符
public void service() throws IOException {
outputStream.write(this.getTopRow().getBytes());
outputStream.write(LINE_SEPARATE_SIGN_BYTES);
for (Map.Entry<String, String> entry : this.getHeaders().entrySet()){
outputStream.write(entry.getKey().getBytes());
outputStream.write(KV_SEPARATE_SIGN_BYTES);
outputStream.write(entry.getValue().getBytes());
outputStream.write(LINE_SEPARATE_SIGN_BYTES);
}
outputStream.write(LINE_SEPARATE_SIGN_BYTES);
outputStream.write(this.getBody());
outputStream.flush();
}
@Override
public String toString() {
return "Response{" +
"topRow='" + topRow + '\'' +
", headers=" + headers +
// ", body=" + Arrays.toString(body) +
", responseCode=" + responseCode +
", client=" + client +
'}';
}
}
#mdb=application/x-mdb
mfp=application/x-shockwave-flash
mht=message/rfc822
mhtml=message/rfc822
mi=application/x-mi
mid=audio/mid
midi=audio/mid
mil=application/x-mil
mml=text/xml
mnd=audio/x-musicnet-download
mns=audio/x-musicnet-stream
mocha=application/x-javascript
movie=video/x-sgi-movie
mp1=audio/mp1
mp2=audio/mp2
mp2v=video/mpeg
mp3=audio/mp3
mp4=video/mp4
mpa=video/x-mpg
mpd=application/vnd
#ms-project
mpe=video/x-mpeg
mpeg=video/mpg
mpg=video/mpg
mpga=audio/rn-mpeg
mpp=application/vnd
#ms-project
mps=video/x-mpeg
mpt=application/vnd
五、总结
https://github.com/haobinliu/app-server/tree/server-x-test/server-x/src/com/lbh
推荐阅读:
还在用 Random生成随机数?试试 ThreadLocalRandom,超好用!
最近面试BAT,整理一份面试资料《Java面试BATJ通关手册》,覆盖了Java核心技术、JVM、Java并发、SSM、微服务、数据库、数据结构等等。
朕已阅
评论