TypeScript 中提升幸福感的 10 个高级技巧
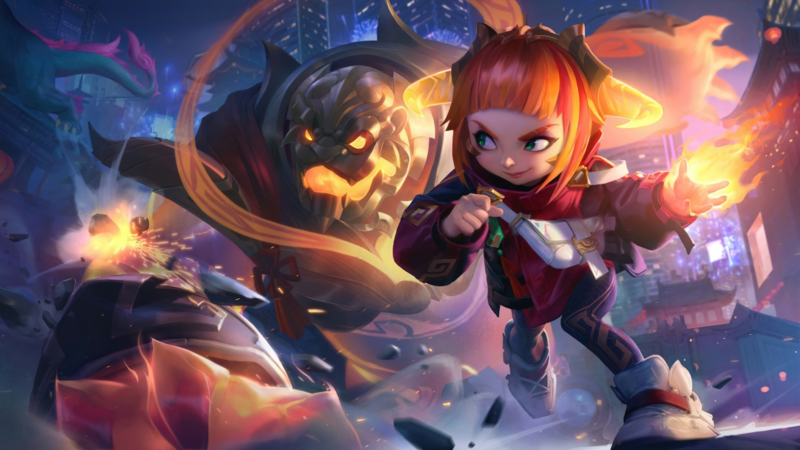
1. 注释
/** This is a cool guy. */
interface Person {
/** This is name. */
name: string,
}
const p: Person = {
name: 'cool'
}
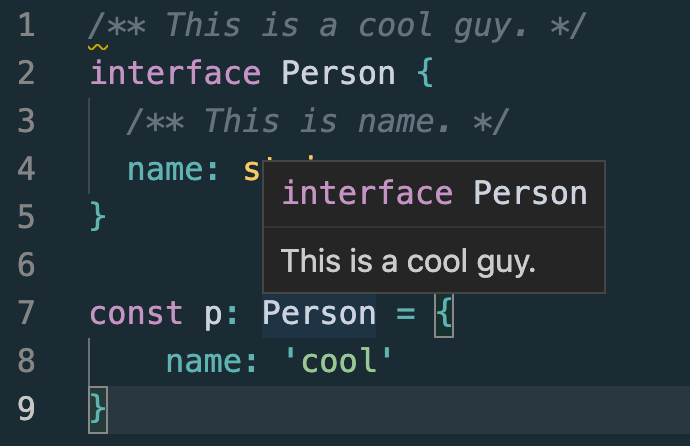
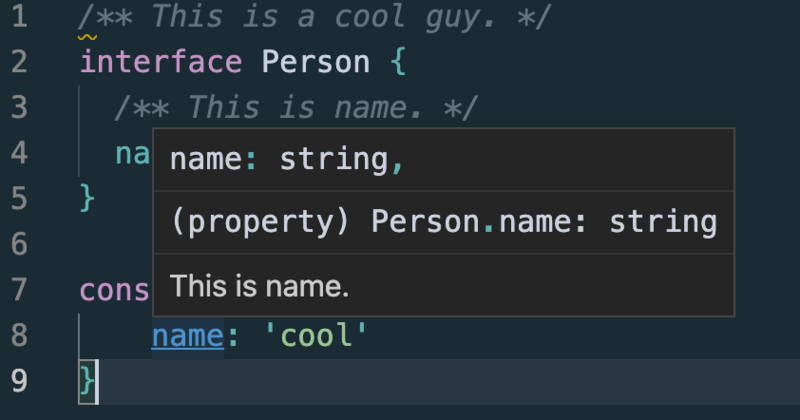
2. 接口继承
interface Shape {
color: string;
}
interface Square extends Shape {
sideLength: number;
}
let square ={};
square.color = "blue";
square.sideLength = 10;
interface Shape {
color: string;
}
interface PenStroke {
penWidth: number;
}
interface Square extends Shape, PenStroke {
sideLength: number;
}
let square ={};
square.color = "blue";
square.sideLength = 10;
square.penWidth = 5.0;
3. interface & type
interface Point {
x: number;
y: number;
}
interface SetPoint {
(x: number, y: number): void;
}
type Point = {
x: number;
y: number;
};
type SetPoint = (x: number, y: number) => void;
interface PartialPointX { x: number; }
interface Point extends PartialPointX { y: number; }
type PartialPointX = { x: number; };
type Point = PartialPointX & { y: number; };
type PartialPointX = { x: number; };
interface Point extends PartialPointX { y: number; }
interface PartialPointX { x: number; }
type Point = PartialPointX & { y: number; };
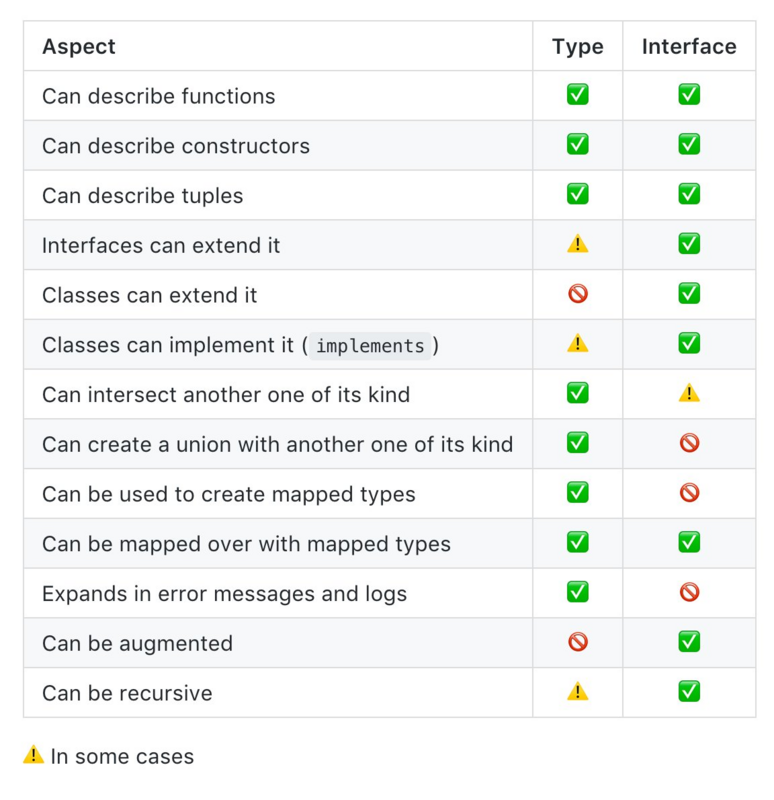
4. typeof
interface Opt {
timeout: number
}
const defaultOption: Opt = {
timeout: 500
}
有时候可以反过来:
const defaultOption = {
timeout: 500
}
type Opt = typeof defaultOption
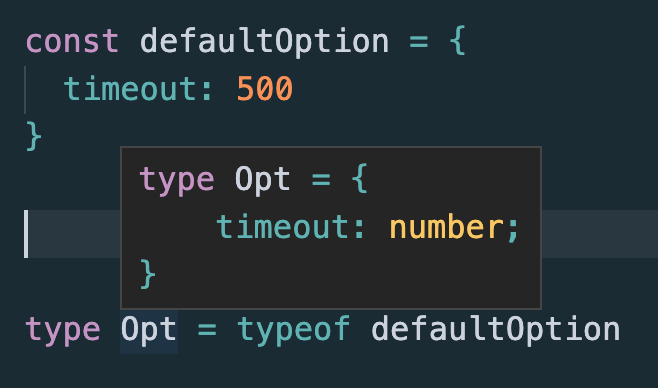
5. keyof
const persion = {
age: 3,
text: 'hello world'
}
// type keys = "age" | "text"
type keys = keyof Point;
function get1(o: object, name: string) {
return o[name];
}
const age1 = get1(persion, 'age');
const text1 = get1(persion, 'text');
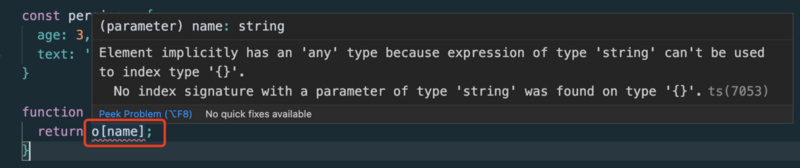
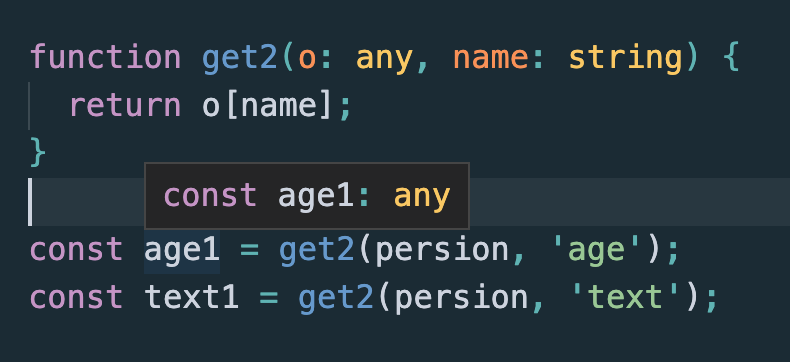
function get
(o: T, name: K): T[K] {
return o[name]
}
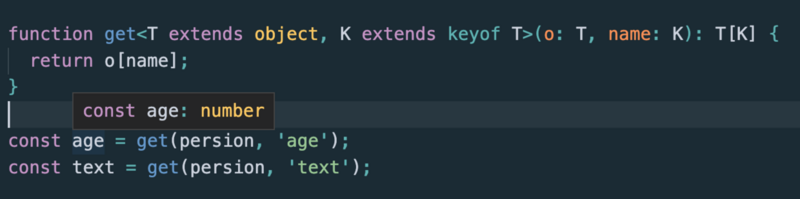
6. 查找类型
interface Person {
addr: {
city: string,
street: string,
num: number,
}
}
interface Address {
city: string,
street: string,
num: number,
}
interface Person {
addr: Address,
}
Person["addr"] // This is Address.
const addr: Person["addr"] = {
city: 'string',
street: 'string',
num: 2
}
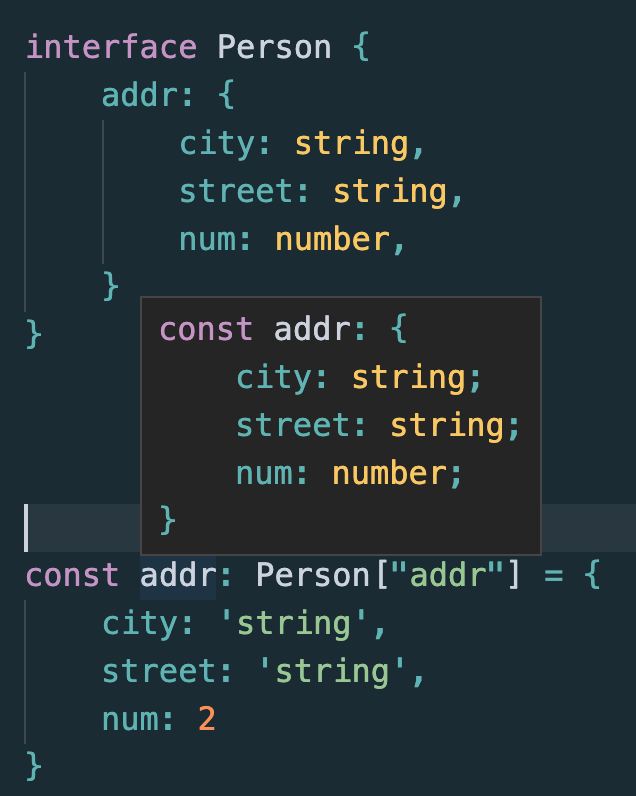
7. 查找类型 + 泛型 + keyof
interface API {
'/user': { name: string },
'/menu': { foods: string[] }
}
const get =(url: URL): Promise => {
return fetch(url).then(res => res.json());
}
get('');
get('/menu').then(user => user.foods);
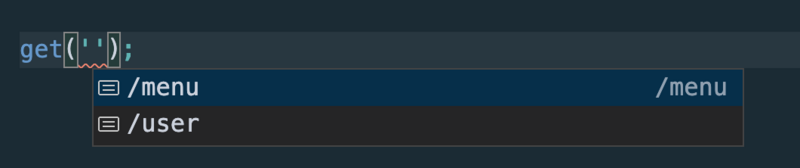

8. 类型断言
"home">
ref="helloRef"
msg="Welcome to Your Vue.js + TypeScript App"
/>
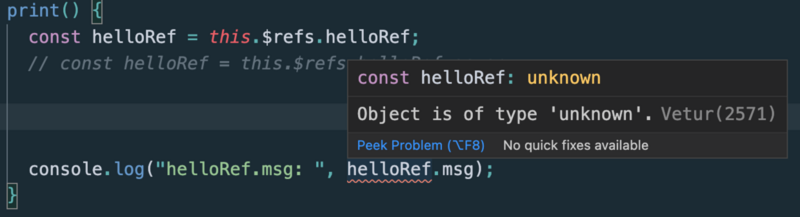
print() {
// const helloRef = this.$refs.helloRef;
const helloRef = this.$refs.helloRef as any;
console.log("helloRef.msg: ", helloRef.msg); // helloRef.msg: Welcome to Your Vue.js + TypeScript App
}
9. 显式泛型
function $
(id: string): T {
return (document.getElementById(id)) as T;
}
// 不确定 input 的类型
// const input = $('input');
// Tell me what element it is.
const input = $('input');
console.log('input.value: ', input.value);
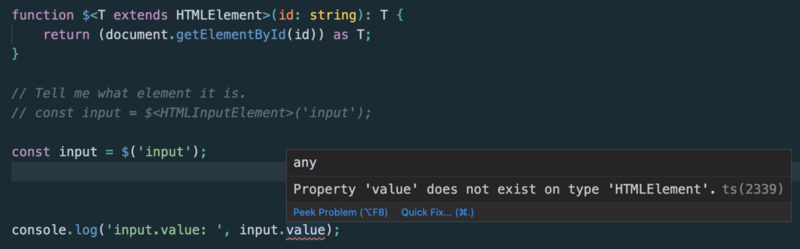
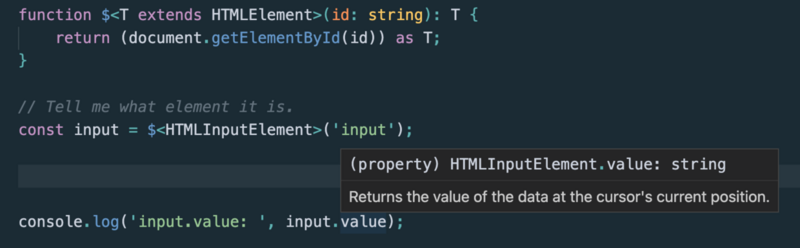
10. DeepReadonly
type DeepReadonly
= {
readonly [P in keyof T]: DeepReadonly;
}
const a = { foo: { bar: 22 } }
const b = a as DeepReadonly
b.foo.bar = 33 // Cannot assign to 'bar' because it is a read-only property.ts(2540)
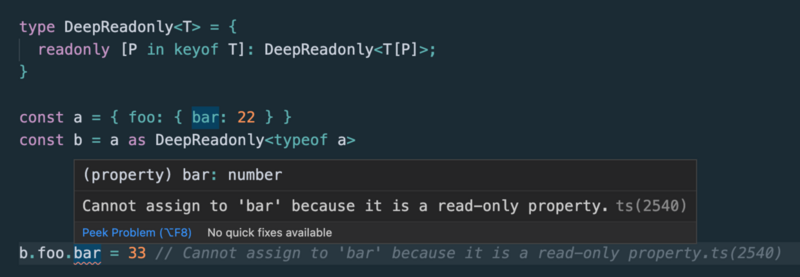
最后
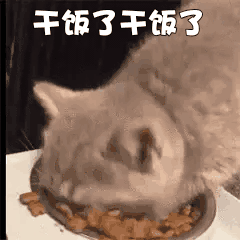
评论