【课程设计|C++】C++程序设计基础:小学生数学练习系统
功能需求
程序需要实现的功能如下:
加法、减法、乘法、除法简易练习 加减混合练习 查看错题(txt文件存储) 自我检测 大整数加法 大整数减法
难度 🌟🌟
程序运行图
系统主界面
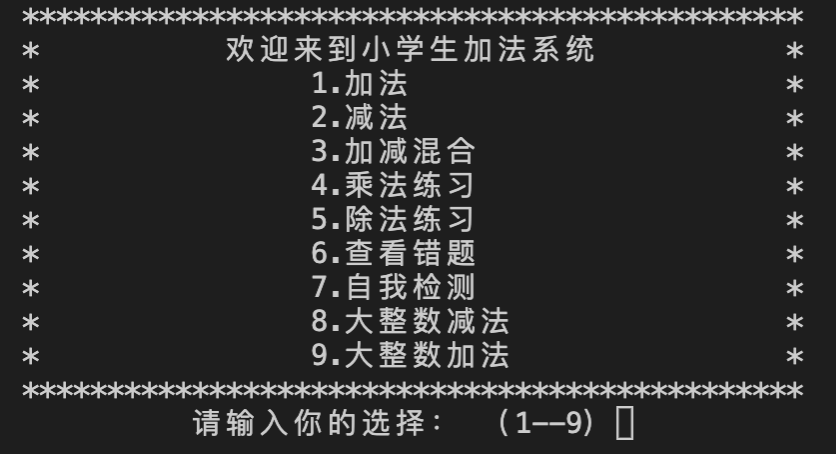
加法练习
用户输入需要练习题目的数量,程序随机生成算式
用户回答正确,得分+10
回答错误,该题存入错题本
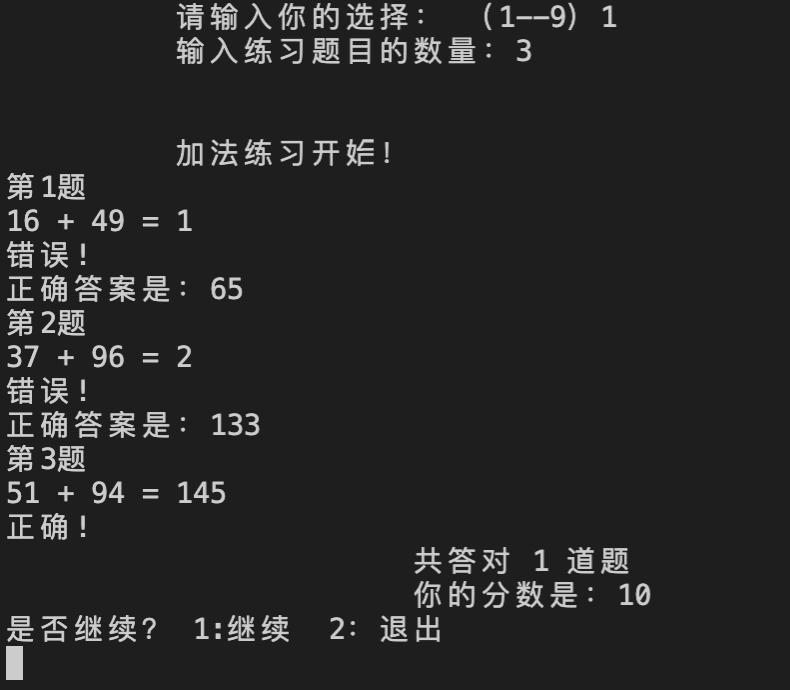
减法练习
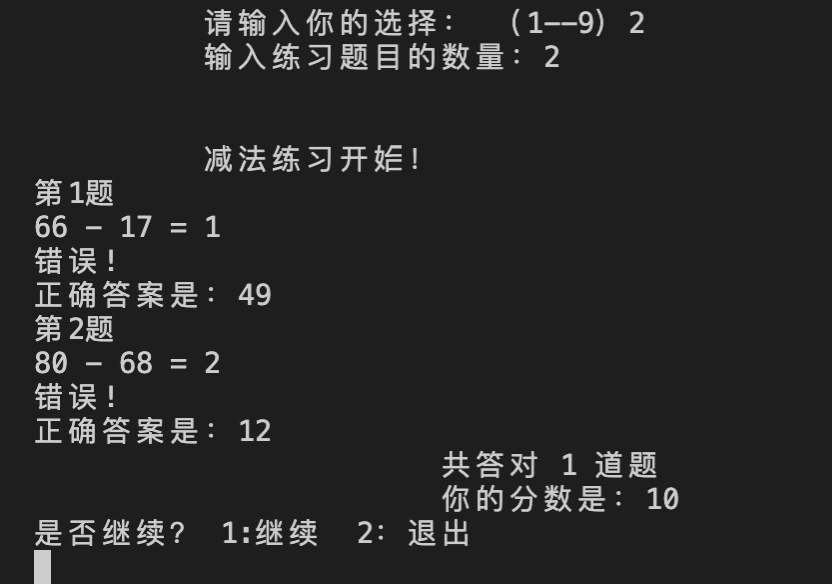
加减混合运算
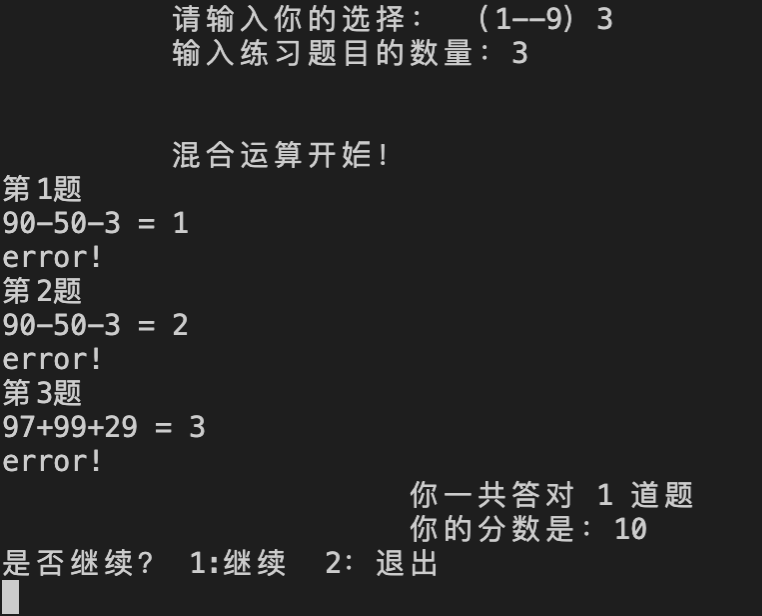
乘法运算
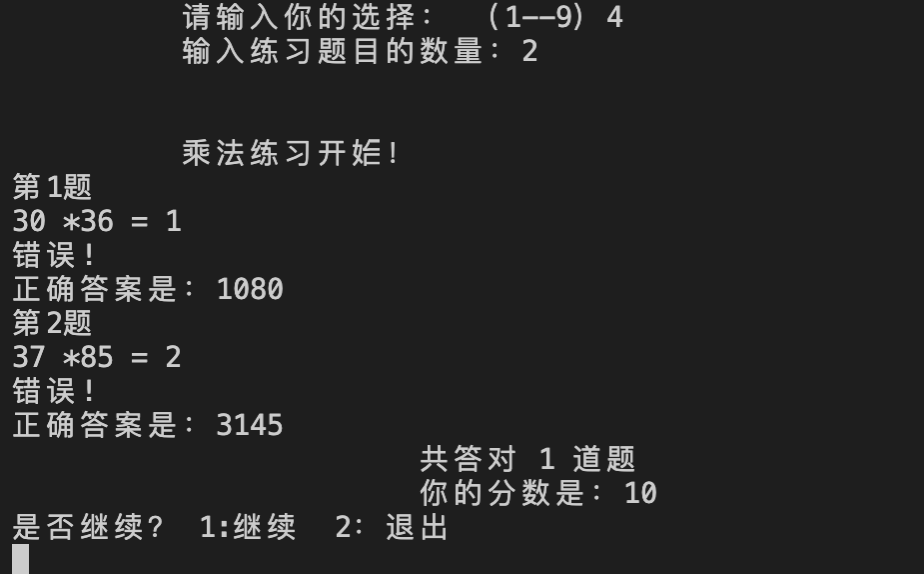
除法运算
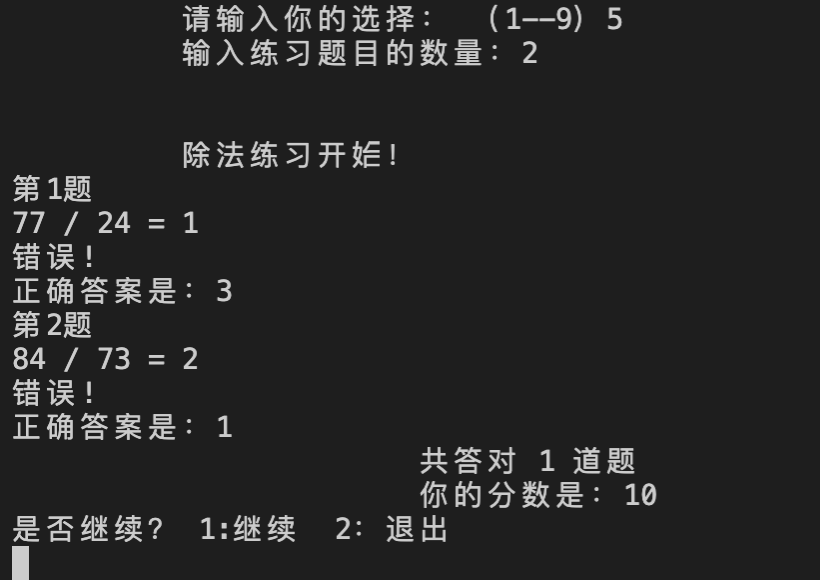
错题本查看
用户做错过的题均存在一个txt文件中
用户可以随时查看
并再做一遍
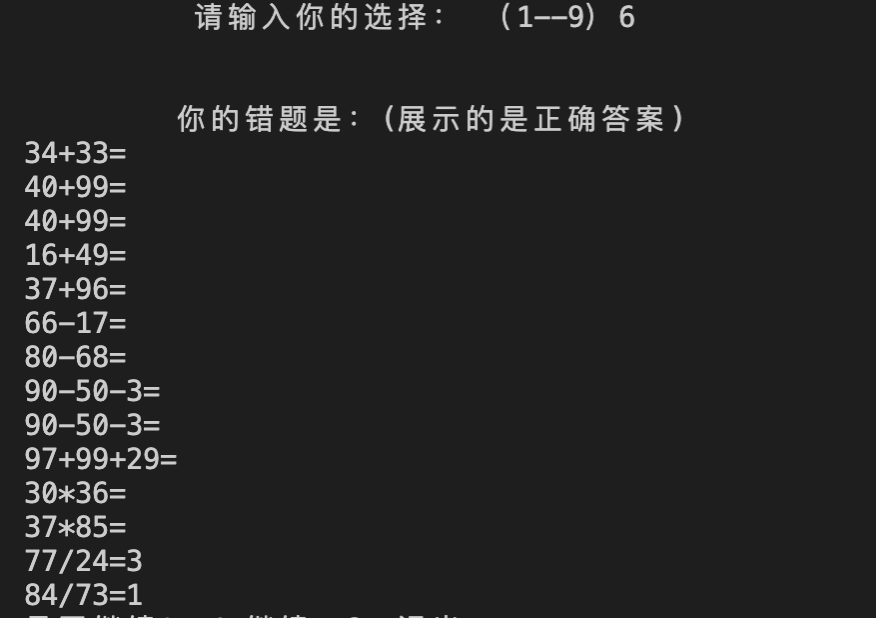
自测功能(难点)
用户使用该功能
可以自行输入一个多项式
如
1+2*3 2✖️3-3+5/4+2-3*3 1+2-3*4/5
程序会自动算出结果并与用户输入进行对比
(这个当时写的时候感觉还是有点难度)
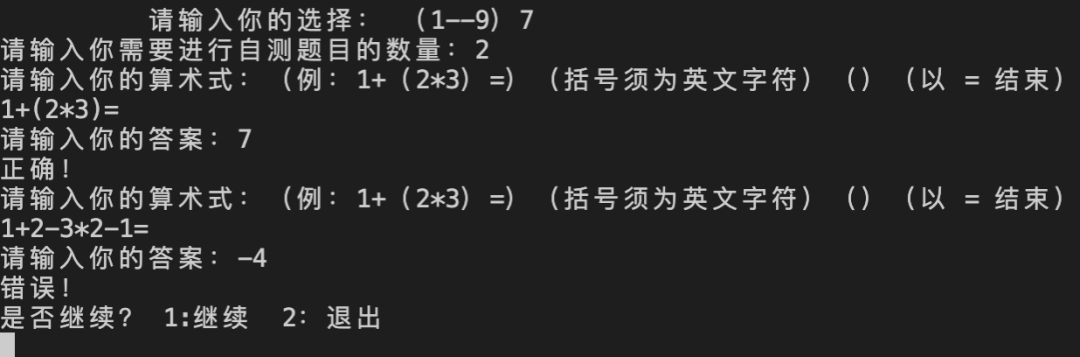
大整数减法(难点)
实现两个任意整数的减法运算,不用担心int、double类型溢出
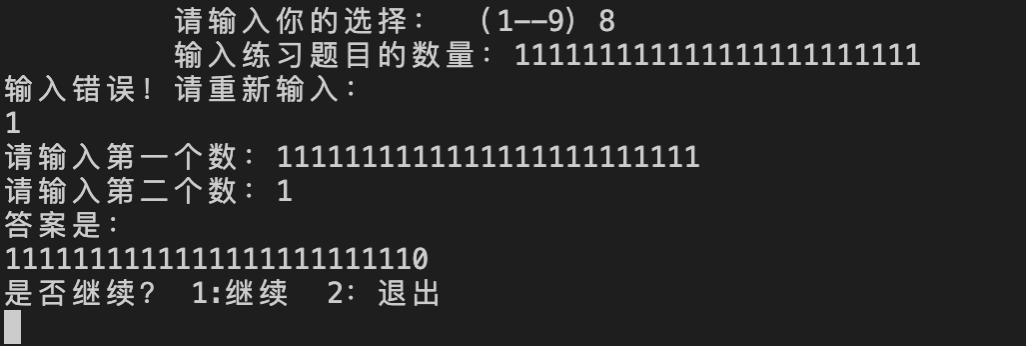
大整数加分(难点)
实现两个任意整数的加法运算,不用担心int、double类型溢出
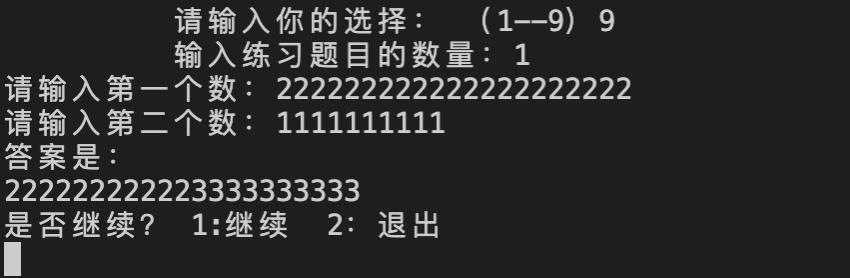
源码
main.cpp
/*
writed by Haihong
time 2018
*/
#include "student.h"
#include "Stack.h"
#include <iostream>
#include <ctime>
#include <sstream>
#include <string>
#include <cstdlib>
#include <string>
#include <fstream>
using namespace std;
//判断 符号优先级
char Priority(char ch1, char ch2)
{
int a;
int b;
switch (ch1)
{
case '#':
a = 0;
break;
case '(':
a = 1;
break;
case '+':
a = 3;
break;
case '-':
a = 3;
break;
case '*':
a = 5;
break;
case '/':
a = 5;
break;
case '%':
a = 5;
break;
case '^':
a = 7;
break;
case ')':
a = 8;
break;
}
switch (ch2)
{
case '#':
b = 0;
break;
case '(':
b = 8;
break;
case '+':
b = 2;
break;
case '-':
b = 2;
break;
case '*':
b = 4;
break;
case '/':
b = 4;
break;
case '%':
b = 4;
break;
case '^':
b = 6;
break;
case ')':
b = 1;
break;
}
if (a < b)
return '<';
else if (a == b)
return '=';
else
return '>';
}
//计算
int Compute(int a, int b, char sign)
{
int result;
switch (sign)
{
case '+':
result = a + b;
break;
case '-':
result = a - b;
break;
case '*':
result = a * b;
break;
case '/':
result = a / b;
break;
case '%':
result = a % b;
break;
case '^':
result = a ^ b;
break;
}
return result;
}
int main()
{
int key = 1;
int key1 = 1;
Student stu;
string str;
int choice;
int choice1;
int num;
while (key == 1)
{
cout << "**********************************************" << endl;
cout << "* 欢迎来到小学生加法系统 *" << endl;
cout << "* 1.加法 *" << endl;
cout << "* 2.减法 *" << endl;
cout << "* 3.加减混合 *" << endl;
cout << "* 4.乘法练习 *" << endl;
cout << "* 5.除法练习 *" << endl;
cout << "* 6.查看错题 *" << endl;
cout << "* 7.自我检测 *" << endl;
cout << "* 8.大整数减法 *" << endl;
cout << "* 9.大整数加法 *" << endl;
cout << "**********************************************" << endl;
cout << " 请输入你的选择: (1--9)";
cin >> choice;
while (cin.fail() || choice < 1 || choice > 10)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear(); //清除流标记
cin.sync(); //清空流
cin >> choice;
}
switch (choice)
{
case 1:
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
stu.jia();
break;
case 2:
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
stu.jian();
break;
case 3:
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
stu.hun();
break;
case 4:
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
stu.cheng();
break;
case 5:
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
stu.chu();
break;
case 6:
stu.again();
break;
case 7:
int n;
cout << "请输入你需要进行自测题目的数量:";
cin >> n;
while (cin.fail() || n < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> n;
}
int i;
for (i = 0; i < n; i++)
{
int ch1 = getchar();
Stack<int> number; //数字栈
Stack<char> character; //运算符栈
character.Push('=');
cout << "请输入你的算术式:(例:1+(2*3)=)(括号须为英文字符)()(以 = 结束)" << endl;
int ch = getchar();
char b;
b = static_cast<char>(ch);
while (b != '=' || character.getTop() != '=')
{
if (ch > 48 && ch <= 57)
{
number.Push(ch - 48);
ch = getchar();
b = static_cast<char>(ch);
}
else
{
switch (Priority(character.getTop(), b))
{
case '<': //栈顶元素优先级低
character.Push(b);
ch = getchar();
b = static_cast<char>(ch);
break;
case '=':
character.Pop();
ch = getchar();
b = static_cast<char>(ch);
break;
case '>': //出栈并且把运算结果入栈
number.Push(Compute(number.Pop(), number.Pop(), character.Pop()));
break;
}
}
}
int anwser;
cout << "请输入你的答案:";
cin >> anwser;
if (anwser == number.getTop())
cout << "正确!" << endl;
else
cout << "错误!" << endl;
}
break;
case 8:
//string numss1;
//string numss2;
// string aaaaaaaaaaaa;
{
string nums1;
string nums2;
// kkk;//答案
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
cout << "请输入第一个数:";
cin >> nums1;
cout << "请输入第二个数:";
cin >> nums2;
//kkk=stu.sub(nums1,nums2);//>>nums2;
//cout<<"1请输入你的答案:";
//cin>>kkk;
cout << "答案是:" << endl;
cout << stu.sub(nums1, nums2) << endl;
// string a;
}
break;
case 9:
string nums1;
string nums2;
cout << " 输入练习题目的数量:";
cin >> num;
while (cin.fail() || num < 0)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> num;
}
stu.setnums(num);
cout << "请输入第一个数:";
cin >> nums1;
cout << "请输入第二个数:";
cin >> nums2; //>>nums2;
cout << "答案是:" << endl;
cout << stu.plus(nums1, nums2) << endl;
break;
}
cout << "是否继续?1:继续 2:退出" << endl;
cin >> choice1;
while (cin.fail() || choice1 < 1 || choice1 > 2)
{
cout << "输入错误!请重新输入:" << endl;
cin.clear();
cin.sync();
cin >> choice1;
}
if (choice1 == 1)
key = 1;
else
key = 0;
system("cls");
system("pause");
}
return 0;
}
Stack.h
//...................................................................................节点类
#ifndef STACK_H
#define STACK_H
template <typename T>
class Node
{
public:
T element;
Node *next;
Node(T element)
{
this->element = element;
next = NULL;
}
};
template <typename T>
class Stack
{
private:
Node<T> *top;
int size;
public:
Stack()
{
Node<T> *newNode = new Node<T>(0);
top = newNode;
size = 0;
}
int stackLength()
{
return size;
}
//判栈为空
bool stackEmpty()
{
if (0 == size)
return true;
else
return false;
}
//入栈
void Push(T e)
{
Node<T> *newNode = new Node<T>(e);
newNode->next = top->next;
top->next = newNode;
size++;
}
//出栈
T Pop()
{
Node<T> *current = top->next;
top->next = current->next;
T f = current->element;
delete current;
size--;
return f;
}
//返回栈顶元素
T getTop()
{
return top->next->element;
}
};
#endif
student.h
#ifndef _student_h_
#define _student_h_
#include <iostream>
#include <ctime>
#include <sstream>
#include <string>
#include <cstdlib>
#include <string>
#include <fstream>
using namespace std;
class Student
{
public:
Student() {}
void jia();
void jian();
void setnums(int a)
{
nums = a;
}
void hun();
void again();
void cheng();
void chu();
string plus(string num1, string num2);
string sub(string num1, string num2);
private:
int nums;
};
static int num = 0, a, b, d, c, sum, result, aa, cc;
void Student::hun()
{
cout << endl;
cout << endl;
cout << " 混合运算开始!" << endl;
for (int i = 0; i < nums; i++)
{
char str1;
char str2;
cout << "第" << i + 1 << "题\n";
srand(time(NULL));
a = rand() % 100 + 1;
b = rand() % 100 + 1;
aa = rand() % 100 + 1;
d = (a % 2 == 1 ? a + b : a - b);
d = (b % 2 == 1 ? d + aa : d - aa);
str1 = (a % 2 == 1 ? '+' : '-');
str2 = (b % 2 == 1 ? '+' : '-');
cout << a << str1 << b << str2 << aa << " = ";
cin >> result;
if (result != d)
{
cout << "error!\n";
ofstream outfile("错题.txt", ios::app);
if (!outfile)
{
cerr << "open error!" << endl;
exit(1);
}
outfile << a << str1 << b << str2 << aa << "=" << endl;
outfile.close();
}
else
{
cout << "right!\n";
num++;
}
}
cout << "\t\t\t你一共答对 " << num << " 道题\n";
cout << "\t\t\t你的分数是:" << num * 10 << endl;
}
void Student::jia()
{
cout << endl;
cout << endl;
cout << " 加法练习开始!" << endl;
for (int i = 0; i < nums; i++)
{
cout << "第" << i + 1 << "题\n";
srand(time(NULL));
a = rand() % 100 + 1;
b = rand() % 100 + 1;
d = a + b;
cout << a << " + " << b << " = ";
cin >> result;
if (result != d)
{
cout << "错误!\n";
cout << "正确答案是:" << d << endl;
ofstream outfile("错题.txt", ios::app);
if (!outfile)
{
cerr << "open error!" << endl;
exit(1);
}
outfile << a << "+" << b << "=" << endl;
outfile.close();
}
else
{
cout << "正确!\n";
num++;
}
}
cout << "\t\t\t共答对 " << num << " 道题\n";
cout << "\t\t\t你的分数是:" << num * 10 << endl;
}
void Student::jian()
{
cout << endl;
cout << endl;
cout << " 减法练习开始!" << endl;
for (int i = 0; i < nums; i++)
{
cout << "第" << i + 1 << "题\n";
srand(time(NULL));
a = rand() % 100 + 1;
b = rand() % 100 + 1;
d = a - b;
cout << a << " - " << b << " = ";
cin >> result;
if (result != d)
{
cout << "错误!\n";
cout << "正确答案是:" << d << endl;
ofstream outfile("错题.txt", ios::app);
if (!outfile)
{
cerr << "open error!" << endl;
exit(1);
}
outfile << a << "-" << b << "=" << endl;
outfile.close();
}
else
{
cout << "正确!\n";
num++;
}
}
cout << "\t\t\t共答对 " << num << " 道题\n";
cout << "\t\t\t你的分数是:" << num * 10 << endl;
}
void Student::again()
{
string str;
cout << endl;
cout << endl;
cout << " 你的错题是:(展示的是正确答案)" << endl;
ifstream infile("错题.txt", ios::in);
if (infile)
{
stringstream buf;
buf << infile.rdbuf();
str = buf.str();
cout << str;
// cout<<a<<endl;
}
else
{
cout << "错误 不能打开文件!" << endl;
}
infile.close();
}
void Student::cheng()
{
cout << endl;
cout << endl;
cout << " 乘法练习开始!" << endl;
for (int i = 0; i < nums; i++)
{
cout << "第" << i + 1 << "题\n";
srand(time(NULL));
a = rand() % 100 + 1;
b = rand() % 100 + 1;
d = a * b;
cout << a << " *" << b << " = ";
cin >> result;
if (result != d)
{
cout << "错误!\n";
cout << "正确答案是:" << d << endl;
ofstream outfile("错题.txt", ios::app);
if (!outfile)
{
cerr << "open error!" << endl;
exit(1);
}
outfile << a << "*" << b << "=" << endl;
outfile.close();
}
else
{
cout << "正确!\n";
num++;
}
}
cout << "\t\t\t共答对 " << num << " 道题\n";
cout << "\t\t\t你的分数是:" << num * 10 << endl;
}
void Student::chu()
{
cout << endl;
cout << endl;
cout << " 除法练习开始!" << endl;
for (int i = 0; i < nums; i++)
{
cout << "第" << i + 1 << "题\n";
srand(time(NULL));
a = rand() % 100 + 1;
b = rand() % 100 + 1;
d = a / b;
cout << a << " / " << b << " = ";
cin >> result;
if (result != d)
{
cout << "错误!\n";
cout << "正确答案是:" << d << endl;
ofstream outfile("错题.txt", ios::app);
if (!outfile)
{
cerr << "open error!" << endl;
exit(1);
}
outfile << a << "/" << b << "=" << d << endl;
outfile.close();
}
else
{
cout << "正确!\n";
num++;
}
}
cout << "\t\t\t共答对 " << num << " 道题\n";
cout << "\t\t\t你的分数是:" << num * 10 << endl;
}
string Student::plus(string num1, string num2)
{
if (num1.size() < num2.size())
{
string temp = num1;
num1 = num2;
num2 = temp;
}
int length1 = num1.size(), length2 = num2.size(), flag = 0, a, b, sum; //flag是进位标记
while (length1 > 0)
{
a = num1[length1 - 1] - '0';
if (length2 > 0)
b = num2[length2 - 1] - '0';
else
b = 0;
sum = a + b + flag;
if (sum >= 10)
{
num1[length1 - 1] = '0' + sum % 10;
flag = 1;
}
else
{
num1[length1 - 1] = '0' + sum;
flag = 0;
}
length1--; //向高位移动1位
length2--; //向高位移动1位
}
if (1 == flag)
num1 = "1" + num1;
return num1;
}
string Student::sub(string num1, string num2)
{
string num11;
int k = 0;
if (num1.size() < num2.size() || num1 < num2)
{
string temp = num1;
num1 = num2;
num2 = temp;
k = 1;
}
int length1 = num1.size(), length2 = num2.size(), flag = 0, a, b, s;
while (length1 > 0)
{
a = num1[length1 - 1] - '0';
if (length2 > 0)
b = num2[length2 - 1] - '0';
else
b = 0;
s = a - b - flag;
if (s < 0)
{
num1[length1 - 1] = 10 + s + '0';
flag = 1;
}
else
{
num1[length1 - 1] = '0' + s;
flag = 0;
}
length1--; //向高位移动1位
length2--; //向高位移动1位
}
//return k=0? num1:'-'+num1;
if (k == 0)
return num1;
else
return '-' + num1;
}
#endif
结语
代码均为原创
版权归作者所有!
仅供小伙伴们参考学习使用!
请勿用于其他用途!
希望对您有所帮助
如有错误欢迎小伙伴指正~
我是 海轰ଘ(੭ˊᵕˋ)੭
创作不易
点个赞吧
谢谢支持❤️
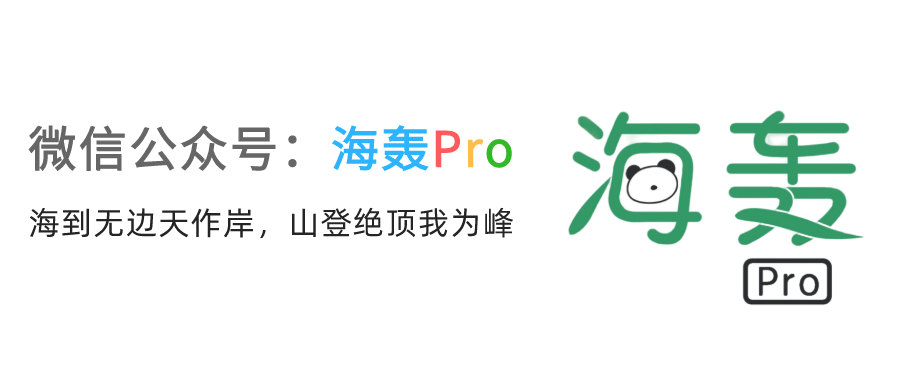
评论