Python标准图形化界面GUI库入门指南– Tkinter
AI算法与图像处理
共 7176字,需浏览 15分钟
·
2021-04-07 01:19
点击上方“AI算法与图像处理”,选择加"星标"或“置顶”
重磅干货,第一时间送达
Tkinter概述
执行
1.导包
import tkinter
import random
from random import randint
from tkinter import Button
import matplotlib.pyplot as plt
import numpy as np
2.创建一个GUI窗口和全局变量声明
root = tkinter.Tk()
root.title("Are you smart!!")
root.geometry("400x200")
correct_result=0
correct_answers=0
total_questions=0
incorrect_answer=0
3.评估结果的函数
def evaluate(event):
global correct_result
global user_input
user_input_given = user_input.get()
if str(user_input_given) == str(correct_result):
global correct_answers
correct_answers += 1
nextQuestion()
else:
global incorrect_answer
incorrect_answer += 1
result = tkinter.Label(root, text="Hard Luck!!nThe correct answer is : "+str(correct_result), font=('Helvetica', 10))
result.pack()
nextQuestion()
root.after(1500, result.destroy)
4.创建问题的函数
def nextQuestion():
user_input.focus_set()
user_input.delete(0, tkinter.END)
global first_num
first_num = randint(1,15)
global second_num
second_num = randint(1,15)
global character
character = random.choice("+-*")
global correct_result
if character == '*':
correct_result = first_num*second_num
if character == '+':
correct_result = first_num+second_num
if character == '-':
correct_result = first_num-second_num
text="Enter the value of "+str(first_num)+" "+character+" "+str(second_num)
global total_questions
total_questions += 1
user_question.config(text=text)
user_question.pack()
5.退出函数
def exitThis():
print("Total Questions attended : "+str(total_questions))
print("Total Correct Answers : "+str(correct_answers))
print("Total Incorrect Answers : "+str(incorrect_answer))
root.destroy()
6.最初的问题
first_num = randint(1,15)
second_num = randint(1,15)
character = random.choice("+-*")
if character == '*':
correct_result = first_num*second_num
if character == '+':
correct_result = first_num+second_num
if character == '-':
correct_result = first_num-second_num
7.标签创建
user_question = tkinter.Label(root, text="Enter the value of "+str(first_num)+" "+character+" "+str(second_num), font=('Helvetica', 10))
user_question.pack()
user_input = tkinter.Entry(root)
root.bind('<Return>',evaluate)
user_input.pack()
user_input.focus_set()
exitButton = Button(root, text="EXIT and Check Result", command=exitThis)
exitButton.pack(side="top", expand=True, padx=4, pady=4)
8.启动GUI
root.mainloop()
9.结果可视化
#Plotting the bar graph
plt.figure(0)
objects = ('Total Number of Questions','Correct Answers','Incorrect answers')
y_pos = np.arange(len(objects))
stats = [total_questions,correct_answers,incorrect_answer]
plt.bar(y_pos, stats, align='center', alpha=0.5)
plt.xticks(y_pos, objects)
plt.ylabel('Numbers')
plt.title('Your Result!')
#Plotting the pie chart
if str(total_questions) != "0":
plt.figure(1)
labels = 'Correct Answers','Incorrect answers'
sizes = [correct_answers,incorrect_answer]
colors = ['green', 'red']
explode = (0.1, 0) # explode 1st slice
plt.pie(sizes, explode=explode, labels=labels, colors=colors,
autopct='%1.1f%%', shadow=True, startangle=140)
plt.axis('equal')
#Show both the graphs
plt.show()
结论
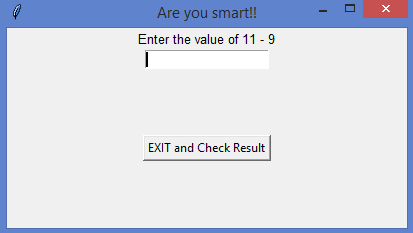
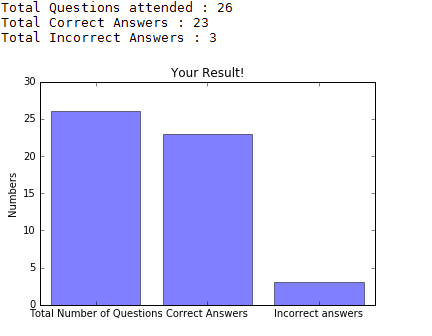
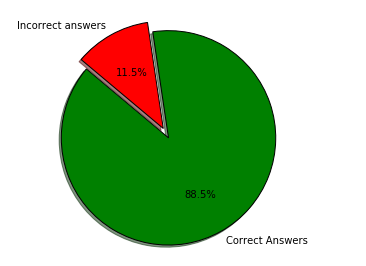
-
https://github.com/prachiprakash26/Play-with-Numbers
个人微信(如果没有备注不拉群!) 请注明:地区+学校/企业+研究方向+昵称
下载1:何恺明顶会分享
在「AI算法与图像处理」公众号后台回复:何恺明,即可下载。总共有6份PDF,涉及 ResNet、Mask RCNN等经典工作的总结分析
下载2:终身受益的编程指南:Google编程风格指南
在「AI算法与图像处理」公众号后台回复:c++,即可下载。历经十年考验,最权威的编程规范!
下载3 CVPR2021
在「AI算法与图像处理」公众号后台回复:CVPR,即可下载1467篇CVPR 2020论文 和 CVPR 2021 最新论文
点亮 ,告诉大家你也在看
评论